The Windows user interface – that familiar landscape of windows, icons, and controls – often appears seamless and intuitive to the end user. However, for developers, particularly those new to the platform, the underlying architecture can feel like a complex maze. This blog aims to demystify the intricate workings of the Windows UI, offering a high-level roadmap to navigate its core components and their interactions.
Table of Contents
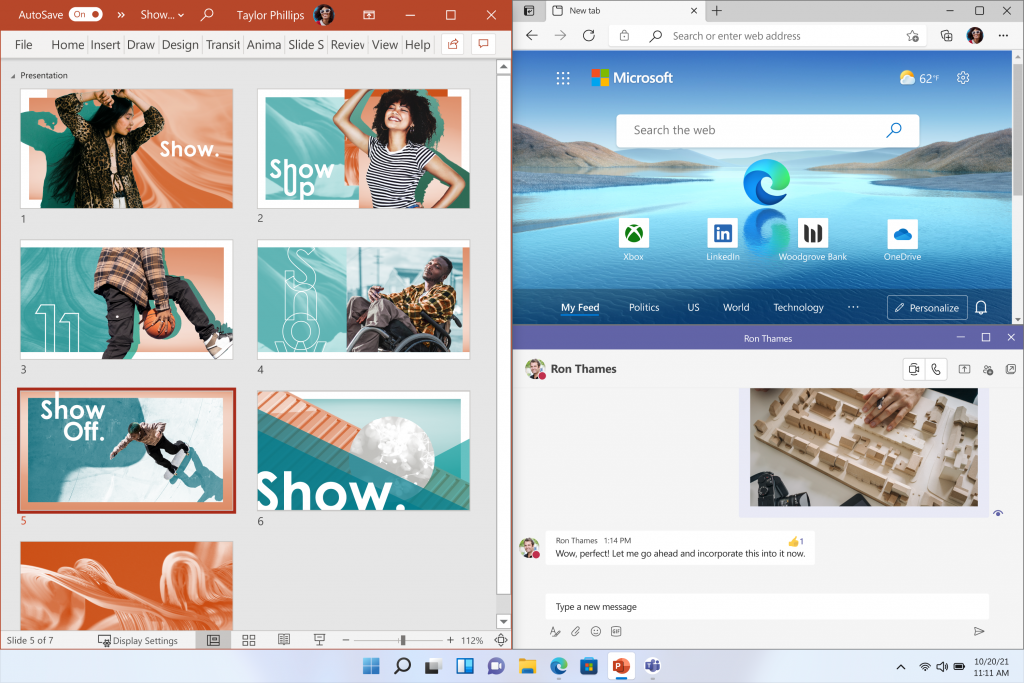
Key Components of the Windows UI
Before diving into specific APIs or code snippets, it’s crucial to establish a clear understanding of the major players orchestrating the Windows UI experience. This understanding forms the bedrock upon which all subsequent development efforts will be built. In this first installment, we’ll introduce the four fundamental elements that collaboratively shape every visual aspect of a Windows application:
- Desktop Window Manager: At the heart of the UI lies the Desktop Window Manager, a system-level component responsible for managing the state and behavior of all windows in a Windows session. Think of it as the traffic controller, directing the flow of information between applications and the display. It maintains crucial details like window size, position, layering (Z-order), and handles user input events, ensuring each application receives the appropriate messages.
- The System Compositor: While the Window Manager manages the logical representation of windows, the System Compositor tackles the visual aspect. This component leverages hardware acceleration to create a visually rich and responsive experience. It takes the instructions from the Window Manager and translates them into pixels on the screen, seamlessly handling effects like transparency, animations, and high-refresh-rate rendering.
- The Shell: Serving as the user’s primary interface to the operating system, the Shell encompasses familiar elements like the Start menu, taskbar, and file explorer. It provides tools for launching applications, managing files, and customizing the user environment. From a developer’s perspective, the Shell offers a set of APIs and extension points to integrate applications into the Windows ecosystem, enabling features like jump lists, notification area icons, and taskbar interactions.
- Applications: Finally, we have the applications themselves, the very reason this intricate system exists. Applications utilize the APIs provided by the Window Manager and other components to create windows, display content, process user input, and interact with the system. Understanding the responsibilities and limitations placed upon applications within this ecosystem is vital for creating well-behaved and user-friendly software.
While our focus remains on these four core elements, it’s important to acknowledge that a multitude of other systems contribute to the overall user experience. Low-level input handling, advanced graphics rendering pipelines, high-level UI frameworks, and assistive technologies all play crucial roles.
By grasping the roles and relationships between the Window Manager, System Compositor, Shell, and Applications, developers gain a solid foundation for building robust and visually appealing applications. This architectural overview serves as your compass, guiding you through the intricacies of Windows UI development. As we continue this series, we’ll equip you with the knowledge and tools to confidently navigate this exciting domain.
The Window Manager
Imagine a bustling city with countless buildings, each representing an application window. The Window Manager acts as the city planner, meticulously tracking and controlling the size, position, and layering of each structure, ensuring visual harmony and preventing chaos.
Technically, the Windows Window Manager (NTUSER) resides primarily in the kernel mode, specifically within win32k.sys. This privileged position grants it direct access to system resources and unparalleled control over the UI environment.
Key Responsibilities
While its name suggests a singular focus, the Window Manager’s responsibilities extend far beyond just windows:
- Window State Management: The Window Manager diligently tracks the state of every window in the system – whether it’s minimized, maximized, restored, or even hidden. It ensures seamless transitions between these states, managing the complex choreography of window resizing, repositioning, and redrawing.
- Message Routing: Communication is key in any well-functioning system, and the Windows UI is no exception. The Window Manager acts as the central message hub, receiving input events from the user (mouse clicks, keystrokes, touch interactions) and routing them to the appropriate application window for processing.
- Z-Order Orchestration: Ever wondered how Windows determines which window appears on top of another? The answer lies in the Z-order – a hierarchical arrangement managed by the Window Manager. It dictates the layering of windows, ensuring the active window always remains visible and interactive.
- Focus Management: The Window Manager determines which window has the current input focus, highlighting it visually and directing keyboard input accordingly. This seemingly simple task involves managing complex rules and interactions, especially when multiple windows compete for attention.
Cooperation and Control
The Windows UI philosophy emphasizes a cooperative model, granting applications significant freedom in managing their windows. However, this freedom necessitates a delicate balance. The Window Manager must ensure system stability and responsiveness even when applications misbehave or become unresponsive.
Next, we’ll explore this intricate dance between application autonomy and system control, diving into the fascinating world of window properties, messages, and the potential pitfalls of a cooperative UI model.
Anatomy of a Window
While users perceive windows as simple rectangular containers for application content, developers understand their intricate nature. Each window possesses a unique set of properties defining its appearance and behavior. These properties, often hidden from plain sight, dictate how a window interacts with the user and the system.
Key Window Properties:
- Window Handle: Like a social security number for windows, the Window Handle (HWND) is a unique identifier assigned by the Window Manager. This numerical value allows the system to track and manage individual windows within the vast sea of UI elements.
- Window Class: Think of a Window Class as a blueprint defining a window’s default appearance and behavior. It determines aspects like the presence of a title bar, system menu, minimize/maximize buttons, and even the iconic rounded corners.
- Window Procedure (WinProc): At the heart of every window lies its Window Procedure – a callback function responsible for handling messages sent by the Window Manager. This function acts as the window’s brain, interpreting user actions and system events, and responding accordingly.
- Window Relationships: Windows don’t exist in isolation. They form complex family trees through parent-child and owner-owned relationships. Understanding these relationships is crucial for managing window activation, focus, and even window destruction.
Window Messages
Communication is paramount in the UI realm, and Windows relies on a sophisticated messaging system to facilitate this exchange. The Window Manager acts as the central post office, receiving and dispatching messages to windows, effectively enabling them to “talk” to each other and the system.
Anatomy of a Window Message
- Message ID: Each message carries a unique numerical ID, indicating its purpose. For example, WM_CLOSE signals a window to close, while WM_PAINT instructs it to redraw its contents.
- WPARAM and LPARAM: These two parameters carry additional data specific to the message. For instance, in a mouse click message, they might contain the cursor coordinates.
The Message Loop
Every application with a UI thread runs a message loop – an infinite loop continuously retrieving messages from the message queue and dispatching them to the appropriate window procedure. This constant cycle ensures that the application remains responsive to user actions and system events.
From Cooperation to Potential Conflict
While the message-based system fosters flexibility and customization, it also introduces an element of uncertainty. Applications have the power to ignore or handle messages in unexpected ways, leading to potential conflicts and unexpected behavior.
In this world of cooperative UI, where applications wield significant control, raises a critical question: How does Windows maintain security and stability in such a seemingly open environment?
Security Isolation Mechanisms
Imagine each Windows user session as a fortified castle, with layers of defenses protecting it from intruders and internal conflicts. Windows implements robust security measures to ensure that applications, despite their freedom, operate within clearly defined boundaries:
- Sessions: At the outermost level, each user logs into their own isolated session. This fundamental division prevents one user’s actions from impacting another’s applications or data.
- Window Stations: Within a session, Window Stations act like separate wings of the castle. Each UI process is associated with a specific Window Station, typically the “interactive” one where the user interacts with applications. This isolation prevents background services or processes running under different user accounts from directly manipulating UI elements within the user’s active desktop.
- Desktops: Each Window Station contains at least one Desktop, representing the visual space where windows are displayed. While less common today, multiple desktops can exist within a Window Station, further isolating groups of applications. However, the primary security significance lies in the distinction between the “input desktop” and others. This prevents malicious applications from hijacking sensitive user interactions, such as those involving UAC prompts.
- User Interface Privilege Isolation (UIPI): UIPI acts as the final line of defense, preventing lower-privileged processes from interfering with higher-privileged ones. This ensures that a standard user application, for instance, cannot send messages or tamper with the windows of an application running with administrator privileges.
The App-Centric View
Despite these security measures, Windows UI remains fundamentally app-centric. Applications enjoy significant freedom in creating and managing their windows, rendering content, and handling user interactions. However, this freedom comes with inherent responsibilities:
- Well-Behaved Applications: Developers are entrusted to write applications that respect system conventions, handle messages appropriately, and avoid interfering with other applications.
- Potential for Conflict: The cooperative nature of the system, while enabling great flexibility, opens the door for potential conflicts. An unresponsive application or one that disregards UI guidelines can disrupt the user experience.
The Windows UI architecture reflects a delicate balance between empowering developers and maintaining system integrity. While applications enjoy considerable freedom, security mechanisms and UI guidelines provide essential guardrails to prevent chaos.
UI Toolkits and the Rise of UWP
Our journey through the Windows UI landscape has taken us from the meticulous control of the Window Manager to the chaotic potential of rogue applications. We’ve witnessed firsthand the power and flexibility offered by the Win32 API, but also the complexity and responsibility that comes with it.
For many developers, venturing directly into the wilds of raw Win32 UI development feels akin to crafting a spaceship from scrap metal. Fortunately, just as aspiring astronauts don’t need to reinvent rocket science, Windows developers have a wealth of tools and frameworks at their disposal.
This chapter explores the evolution of Windows UI development, examining the rise of toolkits and frameworks that streamline the process and, importantly, the emergence of the Universal Windows Platform (UWP).
The Rise of UI Toolkits
Over the years, developers sought to tame the complexities of Win32 UI development, leading to a proliferation of toolkits and frameworks. These tools aimed to:
- Abstract Away Complexity: Hiding the intricacies of window messages, device contexts, and GDI drawing functions behind higher-level abstractions like buttons, text boxes, and layouts.
- Boost Productivity: Providing pre-built components, visual design tools, and reusable code modules to accelerate development and reduce boilerplate code.
- Enhance Visual Appeal: Offering easier ways to incorporate graphics, animations, and advanced visual effects, moving beyond the limitations of basic Win32 controls.
Frameworks:
- Common Controls: The earliest attempt at standardization, Common Controls provided a basic set of reusable UI elements (buttons, list boxes, etc.), albeit with limited customization options.
- MFC and WTL: These C++ frameworks offered more comprehensive abstractions and tools for building complex applications, but often came with their own learning curves and complexities.
- Windows Forms (WinForms): Introduced with .NET, WinForms brought managed code and visual design tools to Windows UI development, significantly improving developer productivity.
- WPF and XAML: WPF revolutionized UI development with its declarative markup language (XAML) and support for hardware-accelerated graphics, enabling visually rich and interactive applications.
Universal Windows Platform (UWP)
With the rise of mobile devices and the need for applications that could run seamlessly across various form factors, Microsoft introduced UWP. This platform marked a significant shift in Windows UI development, embracing:
- A Device-Independent Approach: UWP applications are designed to adapt to different screen sizes, resolutions, and input methods, providing a consistent experience across PCs, tablets, Xbox consoles, and even HoloLens devices.
- A Modern App Container: UWP apps run within a secure sandbox, limiting their access to system resources and preventing them from interfering with other applications or the operating system itself.
- A New UI Framework: Built upon XAML, the UWP UI framework offers a rich set of controls, APIs, and design patterns optimized for touch input, fluid animations, and a modern aesthetic.
Win32 vs. UWP
The choice between Win32 and UWP depends largely on the specific needs of your application and target audience:
Win32:
- Unparalleled Flexibility and Control: Ideal for applications requiring deep system integration, low-level hardware access, or the ability to leverage legacy code and APIs.
- Mature Ecosystem: Decades of development have resulted in a vast ecosystem of libraries, tools, and documentation.
UWP:
- Cross-Device Compatibility: Perfect for applications targeting a wide range of Windows devices, ensuring a consistent user experience across different form factors.
- Modern Features and Security: Provides access to the latest UI features, touch-optimized controls, and a secure app container model.
Interoperability and the Future of Windows UI
While Win32 and UWP represent distinct approaches, Microsoft has made strides in bridging the gap between them, enabling developers to leverage the strengths of both platforms:
- XAML Islands: This technology allows developers to embed modern UWP controls and features within existing Win32 applications, gradually modernizing their UI without a complete rewrite.
- Windows App SDK: The latest evolution of the Windows development platform, the Windows App SDK provides a unified set of APIs and tools for building both Win32 and UWP applications, simplifying development and enhancing interoperability.
The System Compositor
Throughout our journey into the depths of the Windows UI, we’ve encountered the Window Manager meticulously orchestrating windows and the diverse toolkit ecosystem empowering developers. Yet, behind the scenes, a silent magician works tirelessly to transform abstract instructions into the vibrant visuals we see on screen – the System Compositor.
This often-overlooked component, known as the Desktop Window Manager (DWM) in Windows, plays a crucial role in shaping the modern Windows experience. It’s time we shine a light on this unsung hero and uncover the secrets behind its visual wizardry.
The Rise of Desktop Compositing
Cast your mind back to the early days of graphical user interfaces. Dragging a window across another often resulted in unsightly tearing and flickering. Why? Because applications directly drew their content to the screen, leading to conflicts and visual artifacts.
The solution arrived with desktop compositing, a paradigm shift where a dedicated system component intercepts and manages all visual elements before they reach the display. Enter the System Compositor – a silent guardian, diligently composing a unified, flicker-free desktop from individual application windows and system UI elements.
DWM
In Windows Vista, Microsoft introduced DWM, marking a turning point in UI performance and visual fidelity. No longer just a smoother of jagged edges, DWM unleashed a wave of innovations:
- Hardware Acceleration: By harnessing the power of modern GPUs, DWM offloads rendering tasks from the CPU, freeing it for other computations and enabling smoother animations, transitions, and visual effects.
- Advanced Visuals: Transparency, blurring, shadows, and sophisticated animations became standard UI fare, elevating the visual experience beyond the limitations of traditional Win32 controls.
- Improved Responsiveness: By handling window manipulations and animations independently, DWM ensures a fluid and responsive UI, even when individual applications lag or become unresponsive.
DWM’s Contributions
While visually striking effects often steal the spotlight, DWM quietly toils away at numerous tasks that enhance the user experience:
- Seamless Window Management: DWM’s off-screen buffers enable smooth window transitions, eliminating the tearing and flickering of yore. Minimizing, maximizing, and even closing windows become visually graceful affairs.
- Live Thumbnails and Aero Peek: Remember the delight of hovering over a taskbar icon to preview a window’s content? DWM’s ability to capture and render live thumbnails enables these engaging interactions.
- Virtual Desktop Infrastructure: DWM’s prowess extends beyond individual desktops. It plays a crucial role in modern virtual desktop environments, seamlessly switching between desktop instances while maintaining visual fidelity and responsiveness.
While DWM primarily operates behind the scenes, developers can tap into its power through various APIs:
- Windows UI Composition API: This API provides low-level access to DWM’s composition engine, enabling developers to create custom animations, effects, and even build their own high-performance UI elements.
- DirectX and Direct2D: For applications demanding the utmost in graphics performance and control, DWM provides interoperability with DirectX and Direct2D, allowing developers to seamlessly blend 2D and 3D content with the Windows desktop.
- High-DPI Awareness: As high-resolution displays become increasingly prevalent, DWM plays a crucial role in ensuring that applications scale correctly and display content sharply, regardless of screen DPI.
The Composition Process
DWM’s magic hinges on its ability to intercept and manage the visual output of all applications. Here’s a simplified glimpse into this intricate dance:
- Redirection: When an application attempts to draw content to the screen, DWM intercepts the request, redirecting it to an off-screen buffer associated with that window.
- Composition: DWM combines the off-screen buffers of all visible windows, along with system UI elements, into a final, unified desktop image.
- Presentation: This final image is then presented to the display, resulting in the seamless, flicker-free experience we’ve come to expect from modern Windows.
By understanding DWM’s role and capabilities, developers gain a deeper appreciation for the intricate machinery behind the Windows UI. This knowledge empowers them to create visually stunning, responsive, and high-performance applications that harness the full potential of the platform.
The Windows Shell
Throughout our exploration of the Windows UI, we’ve ventured from the meticulous workings of the Window Manager to the visual wizardry of the System Compositor. Yet, no journey into this intricate landscape would be complete without encountering the element that ties it all together: the Windows Shell.
The Shell serves as the primary interface between users, applications, and the underlying operating system. It’s the conductor orchestrating the symphony of everyday computing tasks, providing a consistent and intuitive experience for navigating the digital world.
While often associated solely with the file explorer, the Windows Shell encompasses a far broader range of responsibilities:
- Application Launching and Management: The Start Menu, taskbar, and Run dialog are all part of the Shell’s arsenal for launching, switching between, and managing running applications.
- File System Navigation and Manipulation: The iconic file explorer, with its familiar folder hierarchy, provides a visual interface for navigating, organizing, and interacting with files and folders.
- System Settings and Customization: From managing user accounts to configuring network connections, the Shell provides a centralized gateway to a wealth of system-level settings and personalization options.
- Extensibility and Integration: One of the Shell’s most powerful aspects lies in its extensibility. Developers can leverage its APIs and frameworks to integrate applications seamlessly into the Windows experience.
Shell Features
The Shell isn’t just a user-facing environment; it’s a rich ecosystem for developers seeking to create well-integrated and user-friendly applications. Here’s a glimpse of the possibilities:
- Taskbar Integration: Enhance your application’s presence with jump lists providing quick access to common tasks, progress indicators for long-running operations, and notification badges to grab the user’s attention.
- File Type Associations: Seamlessly associate your application with specific file types, allowing users to open, edit, or interact with those files directly from the file explorer.
- Context Menus and Shell Extensions: Extend the functionality of the file explorer and other Shell components with custom context menu items, providing users with contextual actions relevant to their workflow.
- Thumbnail Providers and Preview Handlers: Enrich the user experience by providing custom thumbnails for your application’s files or even interactive previews directly within the file explorer.
Shell Integration in Action
Imagine a user installs your image editing application. Through careful Shell integration, you can:
- Register your application with the Start Menu: Ensuring users can easily launch it.
- Associate your application with image file types: Enabling users to open images directly in your editor from the file explorer.
- Provide a thumbnail preview handler: Allowing users to see thumbnails of your application’s proprietary image format within the file explorer.
- Add a context menu item to the file explorer: Offering users quick access to your editor when right-clicking on an image file.
A Glimpse into the Future
The Windows Shell, like the operating system it represents, is in a constant state of evolution. New features, APIs, and design paradigms emerge with each iteration of Windows.
As developers, embracing the Shell’s capabilities and staying abreast of its evolution is crucial for crafting applications that feel like natural extensions of the Windows ecosystem. In the final chapter of our Windows UI journey, we’ll put our accumulated knowledge to the test, venturing into a lab where we’ll explore advanced UI techniques, harnessing the power of the Shell, the System Compositor, and the Win32 API to build a truly compelling and integrated Windows experience.
Closing Thoughts
We began our expedition into the world of Windows UI as explorers charting an unfamiliar territory. Starting with the fundamental building blocks of the Window Manager and its intricate dance of messages and properties, we ventured deeper, uncovering the hidden magician behind the scenes – the System Compositor. This powerful component, often overlooked, breathes life into our applications, transforming code into the fluid, visually rich experiences we’ve come to expect from modern operating systems.
Our journey wasn’t limited to system-level components. We donned the mantle of both the diligent developer and the mischievous hacker, experimenting with UI toolkits, pushing the boundaries of application behavior, and discovering the delicate balance between freedom and responsibility that governs the Windows UI ecosystem.
Along the way, we encountered the ever-present Shell – the bridge between users, applications, and the vast capabilities of the Windows platform. We explored its role in launching applications, managing files, and providing a consistent user experience. Most importantly, we discovered the power it affords developers to seamlessly integrate their creations into this intricate ecosystem.
Key Takeaways
This exploration has been more than just a technical deep dive. It’s been a journey of understanding:
- The Power of Cooperation: The Windows UI thrives on a delicate balance between application autonomy and system-level control. Understanding this interplay is crucial for crafting applications that are both powerful and well-behaved.
- The Importance of User Experience: While technical prowess is admirable, it’s the user who ultimately judges the success of our creations. Prioritizing usability, accessibility, and visual appeal should be at the forefront of every design decision.
- The Continuous Journey of Learning: The Windows platform is a living, breathing entity, constantly evolving with new technologies and paradigms. Embracing continuous learning, experimenting with new tools, and engaging with the developer community are essential for staying ahead of the curve.
Continue Reading
- Salesforce Solutions: Mastering Common Scenarios with Ease
- WebAuthn FAQs – Part 13
- WebAuthn FAQs – Part 12
- WebAuthn FAQs – Part 11
- WebAuthn FAQs – Part 10