Table of Contents
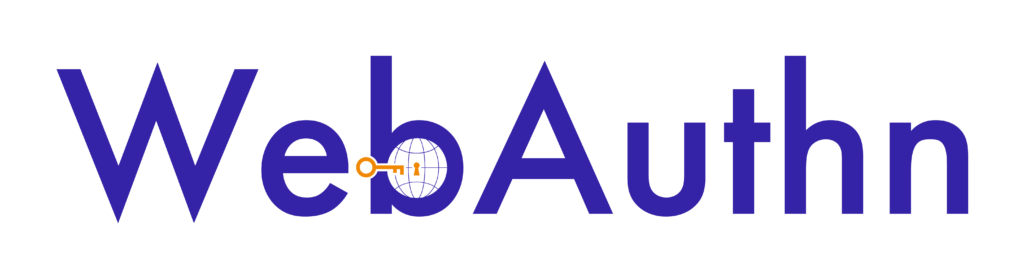
Question: What information is contained within the attestationObject attribute of an AuthenticatorAttestationResponse?
The attestationObject
attribute of an AuthenticatorAttestationResponse
is a crucial part of the WebAuthn registration ceremony. It contains a cryptographically protected package of information that the Relying Party can use to assess the trustworthiness of the new credential.
Here’s a breakdown of the information contained within the attestationObject
:
- Authenticator Data (
authData
): This is a binary structure containing crucial information about the authenticator and the newly generated credential.- AAGUID: A 128-bit Globally Unique Identifier (GUID) assigned to the authenticator model. This helps the Relying Party understand what type of authenticator generated the credential.
- Credential ID: A unique identifier for the credential, used to identify it in future authentication ceremonies.
- Credential Public Key: The public key portion of the generated key pair. This key is used by the Relying Party to verify authentication assertions.
- Flags: A set of bits indicating various properties, such as whether user presence and/or user verification were performed during the ceremony.
- Signature Counter: A counter that increments with each successful authentication assertion. This helps detect cloned authenticators.
- Extensions: Optional data added by specific extensions used during the ceremony.
- Attestation Statement (
attStmt
): This is a signed statement asserting the authenticity of the authenticator and the origin of the credential public key.- Format (
fmt
): A string identifier indicating the specific format used for the attestation statement. - Statement Data: Varies based on the chosen format, but typically includes a signature over the authenticator data, a certificate chain linking the signing key to a trusted root, and potentially additional metadata.
- Format (
How it’s used:
- Verification: The Relying Party uses the data in the
attestationObject
to verify that the attestation statement is valid and signed by a trusted entity. This verification process varies based on the chosen attestation statement format. - Trust Assessment: The Relying Party assesses the trustworthiness of the new credential based on the verified attestation statement. This may involve checking the AAGUID against a list of known authenticator models, evaluating the certificate chain, and potentially using additional information from metadata services.
- Credential Storage: Once verified and assessed, the Relying Party stores the credential public key and ID, along with other relevant information from the
attestationObject
, for use in future authentication ceremonies.
Important Notes:
- The
attestationObject
is opaque to the client and protected against tampering. - Relying Parties can choose to request different levels of attestation, ranging from no attestation to highly detailed statements.
- Understanding attestation is crucial for Relying Parties to make informed trust decisions about new credentials.
Question: What are the helper functions provided for easily accessing credential data during registration?
The WebAuthn specification provides two helper functions for easily accessing credential data during registration, specifically within the AuthenticatorAttestationResponse
interface:
getPublicKey()
:
- This function returns the credential public key as an
ArrayBuffer
in the SubjectPublicKeyInfo format. This format makes it readily usable with common cryptographic libraries in various programming languages:- Java:
java.security.spec.X509EncodedKeySpec
- .NET:
System.Security.Cryptography.ECDsa.ImportSubjectPublicKeyInfo
- Go:
crypto/x509.ParsePKIXPublicKey
- Java:
- It simplifies the process for Relying Parties that don’t require attestation, as they no longer need to parse the COSE formatted public key from the nested
attestationObject
. - Limitations: This function may return
null
if the Relying Party negotiated a public key algorithm viapubKeyCredParams
that the user agent doesn’t understand. In such cases, the public key must be parsed directly fromattestationObject
orauthenticatorData
. - Supported Algorithms: User agents MUST support
getPublicKey()
for the following COSEAlgorithmIdentifier values:-7
(ES256) withkty
2 (uncompressed points) andcrv
1 (P-256)-257
(RS256)-8
(EdDSA) withcrv
6 (Ed25519)
getAuthenticatorData()
:
- This function returns the
authenticatorData
as anArrayBuffer
extracted directly from theattestationObject
. - It eliminates the need for CBOR parsing to access the
authenticatorData
, making it more convenient for Relying Parties. - While
authenticatorData
contains other fields in a binary format, helper functions aren’t provided to access them. Relying Parties already extract these fields during assertion verification, as it always involves signature verification and thus requires parsing theauthenticatorData
.
These helper functions were introduced in WebAuthn Level 2. To ensure compatibility, Relying Parties should use feature detection by checking 'getPublicKey'
in AuthenticatorAttestationResponse.prototype
before utilizing these functions.
Question: Describe the purpose and content of the AuthenticatorAssertionResponse interface.
The AuthenticatorAssertionResponse
interface is a core part of the Web Authentication API (WebAuthn). It represents the data sent back from an authenticator (like a security key or a built-in platform authenticator) to the web browser after a successful user authentication. This data is then forwarded to the website’s server for final verification.
Here’s a breakdown of its purpose and content:
Purpose
The primary purpose of AuthenticatorAssertionResponse
is to provide cryptographic proof to the website that:
- The user possesses the private key associated with a specific credential: This is done via the
signature
field, which is a digital signature generated using the credential’s private key. - The user has authorized the authentication request: The authenticator internally verifies the user (e.g., through a fingerprint scan, PIN, or button press) and includes data indicating the success of this verification in the
authenticatorData
field. - The authentication is tied to a specific website and request: The
clientDataJSON
field, inherited from the parent interface, contains information about the website’s origin and the specific authentication challenge issued.
Content
The AuthenticatorAssertionResponse
interface consists of the following fields:
clientDataJSON
(inherited): A byte array containing a JSON serialization of client data. This data includes information about the website requesting authentication (origin), the challenge issued, and other contextual details.authenticatorData
: A byte array containing authenticator-specific data. This includes:- RP ID Hash: A hash of the website’s identifier (RP ID).
- Flags: Bits indicating various aspects of the authentication, such as whether user presence was verified (
UP
flag), user verification was performed (UV
flag), and other optional flags. - Signature Counter: A value incremented with each successful authentication, helping to detect cloned or compromised authenticators.
- Optional Attested Credential Data: Only included if attestation is requested during authentication. This would include the AAGUID, credential ID, and public key of the credential.
- Optional Extensions: Additional data from authenticator extensions.
signature
: The digital signature generated by the authenticator using the credential’s private key. This signature covers theauthenticatorData
and the hash of theclientDataJSON
, binding the authentication to the specific website and request.userHandle
: An optional byte array containing the user handle provided by the website during registration. This can be used to identify the user if the website didn’t specify a list of acceptable credentials beforehand.attestationObject
: An optional byte array containing an attestation object, only included if attestation is requested during authentication. This provides information about the authenticator itself.
Question: How does the isPasskeyPlatformAuthenticatorAvailable() method assist Relying Parties in guiding user experience?
The isPasskeyPlatformAuthenticatorAvailable()
method is crucial for Relying Parties (websites or apps) to provide a smooth and tailored user experience when registering new passkeys (FIDO credentials). Here’s a breakdown of how it helps:
1. Detection of Passkey Support:
- This method allows the Relying Party to determine if the user’s device (and browser/OS combination) supports creating and storing passkeys. This is essential because passkeys are a relatively new technology, and not all devices have the necessary capabilities.
2. Conditional UI Flows:
- Based on the result of
isPasskeyPlatformAuthenticatorAvailable()
, the Relying Party can adjust the registration flow:- If
true
(passkeys are supported): The website can prominently display passkey registration as a recommended option. They might even hide or downplay less secure methods like passwords. - If
false
(passkeys are not supported): The website can either:- Offer alternative methods: Such as traditional passwords, OTPs, or other FIDO authenticators that might be available (like security keys).
- Explain passkeys and encourage upgrade: The website can educate users about the benefits of passkeys and guide them to upgrade their device or browser if possible to gain support.
- If
3. Reducing User Friction:
- By checking for passkey availability upfront, the Relying Party avoids presenting options that won’t work. This prevents users from going through a frustrating registration process only to encounter an error at the end.
4. Promoting Modern Authentication:
- Relying Parties that prioritize security and user-friendliness want to encourage the adoption of passkeys. This method enables them to make passkeys the default or primary registration choice whenever possible, subtly nudging users toward stronger authentication practices.
Example:
Imagine a user visits a website to create an account. The website runs isPasskeyPlatformAuthenticatorAvailable()
:
- Scenario 1: The method returns
true
- The website displays a large, visually appealing button prompting the user to “Register with Passkey.”
- Options to register with a password or other methods might be hidden behind a “More Options” link.
- Scenario 2: The method returns
false
- The website might display a message: “This device doesn’t yet support passkeys. For a simpler and more secure experience, consider upgrading your device or browser.”
- It then proceeds to offer a standard password registration form.
In essence, isPasskeyPlatformAuthenticatorAvailable()
empowers Relying Parties to dynamically adapt their registration experience, promoting passkeys when available and providing suitable fallbacks when needed. This leads to a smoother and more intuitive experience for users, regardless of their device capabilities.
Question: Explain the purpose and functionality of the parseCreationOptionsFromJSON() and parseRequestOptionsFromJSON() methods.
The parseCreationOptionsFromJSON()
and parseRequestOptionsFromJSON()
methods in the Web Authentication API serve as crucial deserialization tools for Relying Parties during WebAuthn ceremonies. They provide a convenient way to convert JSON representations of ceremony options received from a server into native JavaScript objects that the browser can understand.
Let’s break down the purpose and functionality of each method:
1. parseCreationOptionsFromJSON()
- Purpose:
- This method is used during the registration ceremony to parse the
PublicKeyCredentialCreationOptionsJSON
object sent from the Relying Party’s server. This JSON object contains the parameters required for creating a new public key credential.
- This method is used during the registration ceremony to parse the
- Functionality:
- Takes a
PublicKeyCredentialCreationOptionsJSON
object (a JSON representation of registration options) as input. - Decodes any base64url-encoded
DOMString
attributes (representing binary data like challenge and user ID) back intoArrayBuffers
. - Parses the JSON structure into a native
PublicKeyCredentialCreationOptions
object, which the browser’s WebAuthn API can directly use to initiate credential creation with an authenticator. - If any parsing errors occur, it throws an
EncodingError
DOMException
.
- Takes a
2. parseRequestOptionsFromJSON()
- Purpose:
- This method is used during the authentication ceremony to parse the
PublicKeyCredentialRequestOptionsJSON
object sent from the Relying Party’s server. This JSON object contains the parameters for authenticating the user using an existing credential.
- This method is used during the authentication ceremony to parse the
- Functionality:
- Takes a
PublicKeyCredentialRequestOptionsJSON
object (a JSON representation of authentication options) as input. - Decodes any base64url-encoded
DOMString
attributes back intoArrayBuffers
. - Parses the JSON structure into a native
PublicKeyCredentialRequestOptions
object, which the browser’s WebAuthn API can directly use to initiate authentication with an authenticator. - If any parsing errors occur, it throws an
EncodingError
DOMException
.
- Takes a
Why are these methods important?
- Server-side convenience: Relying Party servers usually work with JSON for data transmission. These methods allow the server to send options in a standard, easily serializable format.
- Client-side efficiency: The browser’s WebAuthn API works with JavaScript objects. These methods handle the necessary conversion, eliminating the need for Relying Party developers to write their own parsing logic.
- Security: Base64url decoding ensures that binary data is handled correctly and securely transmitted, preventing potential manipulation or errors.
parseCreationOptionsFromJSON()
and parseRequestOptionsFromJSON()
simplify WebAuthn integration for Relying Parties by providing a safe and standardized way to handle the transfer and processing of ceremony options between the server and the client.
Question: What are the implications of using the [[preventSilentAccess]]() method for WebAuthn credentials?
The [[preventSilentAccess]]()
method, inherited from the Credential Management API, is designed to prevent credentials from being automatically accessed without explicit user interaction. However, its implications for WebAuthn credentials are nuanced and might not behave as expected for some authenticators.
Here’s a breakdown:
How [[preventSilentAccess]]()
is intended to work:
- General Credentials: It signals to the browser that a credential should only be used after an explicit user action (like a button click). This helps prevent websites from silently checking for the existence of credentials or automatically logging in users without their knowledge.
How it works with WebAuthn:
- Authenticators requiring user interaction: WebAuthn already inherently mandates user interaction (authorization gesture) for both registration and authentication ceremonies. This means that calling
[[preventSilentAccess]]()
has no real impact on authenticators like security keys or platform authenticators that require a touch, PIN, or biometric verification. The authorization gesture already serves the purpose of preventing silent access. - Potentially excluding some authenticators: The method could potentially prevent the use of certain hypothetical authenticators that might operate without user intervention. Imagine an authenticator that could silently provide assertions based on proximity or some other passive signal. Setting
[[preventSilentAccess]]()
might exclude these from consideration.
Key Points:
- Redundant for most WebAuthn scenarios: WebAuthn’s built-in user interaction requirement makes
[[preventSilentAccess]]()
largely redundant for common authenticator types. - Potential future impact: The method might become relevant if new authenticator types emerge that allow credential use without explicit user action.
- Limited practical effect currently: In practice, most browsers and authenticators do not alter their behavior significantly when
[[preventSilentAccess]]()
is set for WebAuthn credentials.
Recommendation:
While setting [[preventSilentAccess]]()
doesn’t harm anything, it’s usually unnecessary for WebAuthn implementations due to the inherent security mechanisms of the protocol. Focus on correctly implementing the core WebAuthn security practices outlined in the specification.
Reference
Web Authentication: An API for accessing Public Key Credentials – Level 3 (w3.org)