Table of Contents
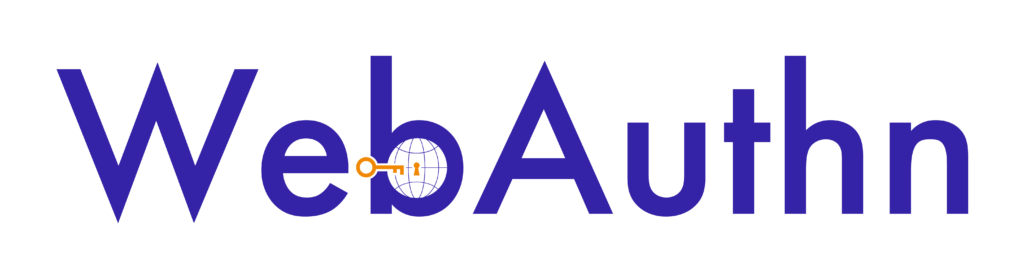
Question: What are the main attributes of the PublicKeyCredential interface?
The PublicKeyCredential
interface, which inherits from the Credential
interface, plays a crucial role in the WebAuthn API. It represents a public key credential obtained during a registration or authentication ceremony. Here are its main attributes:
id
(Inherited fromCredential
but overridden): This attribute represents the credential’s identifier, which is the credential ID chosen by the authenticator. It is aDOMString
representing the Base64URL-encoded version of the credential ID. Unlike theCredential
interface,PublicKeyCredential
returns the Base64URL-encoded representation instead of the raw bytes.rawId
: This attribute gives you the raw credential ID as anArrayBuffer
. This is useful when you need the raw bytes for cryptographic operations.response
: This attribute, of typeAuthenticatorResponse
, holds the authenticator’s response to the request, whether for credential creation or assertion generation.
- For registration (
create()
),response
will be anAuthenticatorAttestationResponse
object, containing information about the new credential and attestation data. - For authentication (
get()
),response
will be anAuthenticatorAssertionResponse
object, containing the authentication assertion and related data.
authenticatorAttachment
: This attribute, a nullableDOMString
, indicates the attachment modality of the authenticator used:
"platform"
: Denotes a platform authenticator, integrated into the user’s device."cross-platform"
: Denotes a roaming authenticator, like a security key.
These four attributes are the core properties of a PublicKeyCredential
object. They provide the essential information about the credential itself, its origin, and how it was obtained.
In addition to these main attributes, the PublicKeyCredential
interface also provides several methods to access extension results, check for specific authenticator availability, and parse ceremony options from JSON format.
Question: Describe the purpose and content of the response attribute in the PublicKeyCredential interface.
The response
attribute of the PublicKeyCredential
interface is crucial for both registration and authentication ceremonies in WebAuthn. It encapsulates the authenticator’s response to the client’s request, offering detailed information about the operation’s outcome.
Let’s break down its purpose and content:
Purpose
- Proof of interaction with the authenticator: It provides evidence that the client successfully interacted with the authenticator to create a new credential or generate an assertion.
- Details of the operation: It contains data specific to the performed ceremony (registration or authentication), allowing the Relying Party to validate the process and the user’s action.
Content
The response
attribute holds an object that implements the AuthenticatorResponse
interface. However, the specific type of the object depends on the ceremony:
- Registration Ceremony (
navigator.credentials.create
):
- The
response
attribute contains anAuthenticatorAttestationResponse
object. - This object includes:
clientDataJSON
: A byte array carrying the client data in JSON format.attestationObject
: A byte array containing the attestation object, which is cryptographically protected and offers proof of the authenticator’s origin and the generated credential’s properties.
- Authentication Ceremony (
navigator.credentials.get
):
- The
response
attribute contains anAuthenticatorAssertionResponse
object. - This object includes:
clientDataJSON
: A byte array holding the client data in JSON format.authenticatorData
: A byte array with the authenticator data, including details like the user presence and verification flags.signature
: A byte array representing the cryptographic signature generated using the credential’s private key, verifying possession of the credential.userHandle
: An optional byte array containing the user handle, used to identify the user account.attestationObject
: An optional byte array holding the attestation object, providing additional assurance about the authenticator.
Question: How does the authenticatorAttachment attribute help Relying Parties guide user experience?
The authenticatorAttachment
attribute, available on the PublicKeyCredential
object returned by both navigator.credentials.create()
and navigator.credentials.get()
, provides valuable information about the type of authenticator used in a WebAuthn ceremony. This information allows Relying Parties to tailor user experiences and guide users towards more convenient or secure options.
Here’s how authenticatorAttachment
helps:
1. Identifying Authenticator Type:
authenticatorAttachment
can have two values:- “platform”: Indicates a platform authenticator, usually built-in to the user’s device (like a fingerprint sensor or Windows Hello).
- “cross-platform”: Indicates a roaming authenticator, a separate device like a security key or a mobile phone acting as one.
2. Guiding Registration Flows:
- Encouraging Platform Authenticator Registration: If
authenticatorAttachment
is “cross-platform” andisUserVerifyingPlatformAuthenticatorAvailable()
returnstrue
, the Relying Party knows the user has a platform authenticator available but chose a roaming authenticator. The RP can then prompt the user to also register their platform authenticator for more convenient future logins. - Enforcing Roaming Authenticator: If the RP requires a roaming authenticator (e.g., for account recovery or higher security), they can check
authenticatorAttachment
during registration and guide the user accordingly.
3. Optimizing Authentication Experience:
- Streamlined Login: If
authenticatorAttachment
is “platform” during authentication, the RP can expect a faster, more integrated login process. They might skip additional steps or prompts that would be needed for a roaming authenticator. - Context-Aware Prompts: The RP can tailor authentication prompts based on the authenticator type. For example, a prompt for a platform authenticator might say “Use your fingerprint” while a prompt for a roaming authenticator might say “Insert your security key”.
4. Analytics and Monitoring:
authenticatorAttachment
can help RPs gather data about authenticator usage patterns, which can inform decisions about security policies and feature development.
Example Scenario:
Imagine a user signs up for an account using a security key (cross-platform). During registration, the RP detects that the user also has a platform authenticator. They then prompt the user to “Register this device for easier logins” using their platform authenticator. In the future, the user can choose to log in quickly with their platform authenticator or use their security key for higher security or when accessing the account from a different device.
In summary, the authenticatorAttachment
attribute empowers Relying Parties to:
- Understand user authenticator preferences.
- Encourage registration of more convenient authenticators.
- Optimize and customize authentication flows.
- Improve overall user experience.
Question: Explain the algorithm for creating a new credential via the [[Create]]() method.
Let’s break down the [[Create]](origin, options, sameOriginWithAncestors)
method algorithm, which is the heart of WebAuthn’s registration process. This is what happens when a Relying Party calls navigator.credentials.create()
to register a new credential with an authenticator.
1. Setup and Initial Checks
- Input: The method receives the
origin
(website making the request),options
(registration preferences), andsameOriginWithAncestors
(flag indicating if the request originated from the same site). - Permissions: It first checks if the request is allowed by the site’s Permissions Policy (is the website allowed to use WebAuthn). It throws an error if not.
- Options Validation: The
options.publicKey
object is validated:- The
timeout
value is adjusted to a reasonable range if necessary. - The
user.id
length is checked (between 1 and 64 bytes). - The origin is validated to be a secure origin.
- The
rp.id
(Relying Party ID) is checked for validity against the origin’s domain.
- The
- Algorithm Preparation:
- A list of supported credential types and algorithms is created based on the
pubKeyCredParams
option. - Client and authenticator extension maps are initialized.
- Client data (type, challenge, origin) is collected and hashed.
- A list of supported credential types and algorithms is created based on the
2. Authenticator Discovery and Selection
- Timeout: A timer is set based on the
timeout
value. The algorithm iterates until the timer expires. - Available Authenticators: The client searches for available authenticators on the user’s device.
- Authenticator Filtering: The algorithm iterates through discovered authenticators and filters them according to the
authenticatorSelection
criteria:authenticatorAttachment
: platform vs. cross-platformresidentKey
/requireResidentKey
: Whether a client-side discoverable credential is required or preferreduserVerification
: Whether user verification is required
- Exclude Credentials: Any authenticators that contain credentials listed in
excludeCredentials
are handled specially (more on this below). - User Interaction: During this process, the browser displays UI to guide the user in selecting an authenticator (if multiple are available) and authorize the operation.
3. Credential Creation on the Authenticator
- User Consent: If
requireUserVerification
is true, user verification (e.g., fingerprint, PIN) is performed. If the user refuses, the process stops. - Key Generation: A new key pair is generated on the authenticator using the preferred algorithm.
- Credential Source Creation: A
PublicKeyCredentialSource
object is created, containing:- Type (“public-key”)
- Private key
- Relying Party ID (
rp.id
) - User handle (
user.id
) - Optional UI information (e.g., user’s display name)
- Credential ID and Storage:
- Resident Key: If a resident key is required or preferred, a new random
credentialId
is generated, and thePublicKeyCredentialSource
is stored on the authenticator, linked to the RP ID and user handle. - Non-Resident Key: If a resident key is not required, the
PublicKeyCredentialSource
is encrypted using a key specific to the authenticator, and the ciphertext becomes thecredentialId
.
- Resident Key: If a resident key is required or preferred, a new random
- Attestation Object Generation: The authenticator generates an attestation object, which includes:
- Authenticator data: Encodes metadata about the authenticator and the generated key (e.g., RP ID hash, flags, signature counter).
- Attestation statement: A cryptographic signature that verifies the authenticity of the authenticator and the generated key. The format of this statement depends on the authenticator.
4. Response and Cleanup
- Return Value: A new
PublicKeyCredential
object is created, containing:- Credential ID
- Authenticator attachment
- Authenticator response (
AuthenticatorAttestationResponse
) with:- Client data
- Attestation object
- Supported transports
- Cancel Operations: Any pending operations on other authenticators are canceled.
Special Handling of excludeCredentials
The excludeCredentials
parameter is used to prevent creating duplicate credentials on the same authenticator. The special handling ensures that the mere presence of an excluded credential doesn’t leak information:
- If an
excludeCredentials
match is found, the authenticator requests user consent not to create a credential. This step might seem odd, but it’s a privacy measure. - If the user consents (to not create a credential), the authenticator returns an
InvalidStateError
. This signals to the Relying Party that a duplicate credential might exist and guides the user to use a different authenticator. - If the user doesn’t consent, the authenticator returns a
NotAllowedError
, hiding the fact that an excluded credential was found.
Key Points
- User Control: The user is actively involved through the selection and authorization of the authenticator.
- Security and Privacy: The algorithm is designed to prevent various attacks (e.g., replay, man-in-the-middle) and protect user privacy.
- Flexibility: WebAuthn supports different authenticator types, key algorithms, and attestation formats, giving flexibility to Relying Parties and users.
Question: What are the recommended ranges and default values for the pkOptions.timeout parameter during credential creation?
The recommended ranges and default value for the pkOptions.timeout
parameter during credential creation, as per the WebAuthn Level 3 specification, are:
- Recommended range: 300,000 milliseconds (5 minutes) to 600,000 milliseconds (10 minutes).
- Recommended default value: 300,000 milliseconds (5 minutes).
This means that relying parties should ideally set a timeout between 5 and 10 minutes for the navigator.credentials.create()
operation. If they don’t specify a timeout, the user agent should default to 5 minutes.
It’s worth noting that these are recommendations, and clients may override the provided timeout value. They should also take cognitive guidelines into consideration regarding timeouts for users with special needs.
Question: How does the excludeCredentials parameter help prevent duplicate credential registrations?
The excludeCredentials
parameter plays a crucial role in preventing duplicate credential registrations in WebAuthn. It functions as a safeguard against inadvertently creating multiple credentials for the same user account on a single authenticator. Here’s a detailed explanation:
How it Works:
- Relying Party Supplies Known Credentials: When initiating a registration ceremony (using
navigator.credentials.create()
), the Relying Party (website or service) can optionally populate theexcludeCredentials
parameter. This parameter is a list ofPublicKeyCredentialDescriptor
objects, each representing a credential already associated with the user account. - Client Checks for Matching Credentials: The WebAuthn client (usually the browser) receives this list and compares it against the credentials stored on each available authenticator.
- Authenticator Confirmation and User Consent: If a match is found (meaning an authenticator already holds a credential for the user), the client takes the following steps:
- Confirmation: The client interacts with the authenticator to confirm the presence of the matching credential. This is done in a privacy-preserving way to prevent malicious Relying Parties from probing for the existence of credentials.
- User Consent: If confirmed, the client seeks user consent to create a new credential. This is crucial because the user may have intentionally registered with the same authenticator before (e.g., for backup purposes).
- Preventing Duplicate Registration:
- User Declines: If the user declines, the ceremony is aborted, preventing the creation of a duplicate credential.
- User Consents: If the user consents, the client signals an error (
InvalidStateError
), indicating to the Relying Party that a credential already exists on the chosen authenticator. The Relying Party can then guide the user to select a different authenticator or manage their existing credentials.
Benefits of Using excludeCredentials
:
- Improved User Experience: Prevents users from accidentally creating multiple credentials on the same device, which can lead to confusion during authentication.
- Enhanced Security: Reduces the risk of account compromise. If multiple credentials exist on a single authenticator, losing that authenticator could grant an attacker access to all associated accounts.
- Streamlined Account Recovery: By guiding users to use distinct authenticators, Relying Parties can provide better support for account recovery scenarios.
Important Considerations:
- Accuracy of Transport Hints: The
transports
field withinPublicKeyCredentialDescriptor
provides hints about how to communicate with the authenticator. However, these hints may become outdated due to software updates or changes in connectivity options. Inaccurate hints could result in the client failing to detect an existing credential, potentially leading to duplicates. - User Consent: User consent is paramount. Even if a matching credential is found, the client must respect the user’s decision on whether to create a new credential.
Question: How does the authenticatorSelection parameter influence the selection of an authenticator for credential creation?
The authenticatorSelection
parameter within PublicKeyCredentialCreationOptions
plays a crucial role in guiding the WebAuthn client to select the most suitable authenticator for credential creation. It allows Relying Parties to specify preferences and requirements for authenticator capabilities. Let’s break down how this parameter influences authenticator selection:
1. authenticatorAttachment
:
- Values:
"platform"
,"cross-platform"
, or absent (meaning any attachment is acceptable). - Influence: This field narrows down the choices based on the physical attachment of the authenticator.
"platform"
: Prefers an authenticator built into the user’s device (e.g., fingerprint sensor, Windows Hello)."cross-platform"
: Prefers an external authenticator that can connect to various devices (e.g., USB security key, phone as an authenticator).
2. residentKey
:
- Values:
"discouraged"
,"preferred"
,"required"
, or absent. - Influence: Determines the preference for a client-side discoverable credential (formerly “resident key”), which is stored on the authenticator and allows authentication without providing a credential ID.
"discouraged"
: Prefers a server-side credential, which is not stored on the authenticator."preferred"
: Prefers a client-side discoverable credential if possible."required"
: Requires a client-side discoverable credential. The operation fails if one cannot be created.
3. requireResidentKey
:
- Values:
true
orfalse
(default isfalse
). - Influence: This option is deprecated in favor of using
residentKey
. It has the same effect as settingresidentKey
to"required"
whentrue
.
4. userVerification
:
- Values:
"required"
,"preferred"
,"discouraged"
, or absent. - Influence: Specifies the Relying Party’s preference for user verification, which adds an additional layer of security.
"required"
: User verification (e.g., fingerprint, PIN, facial scan) MUST be performed. The operation fails if the authenticator doesn’t support it."preferred"
: User verification is preferred but not mandatory."discouraged"
: User verification is not desired.
Example:
Let’s say a Relying Party wants to create a credential that is:
- Stored on the user’s device (
authenticatorAttachment: "platform"
) - Client-side discoverable (
residentKey: "preferred"
) - Requires user verification (
userVerification: "required"
)
Their authenticatorSelection
might look like this:
authenticatorSelection: {
authenticatorAttachment: "platform",
residentKey: "preferred",
userVerification: "required"
}
How the Client Uses the Information:
The WebAuthn client within the browser or operating system considers the authenticatorSelection
criteria when discovering and selecting an appropriate authenticator:
- Filtering: The client filters out authenticators that don’t meet the specified requirements.
- Prioritization: For preferences (
"preferred"
or"discouraged"
), the client prioritizes authenticators that align with those preferences.
Key Points:
- All fields in
authenticatorSelection
are optional. - The client makes a best-effort to fulfill preferences but will proceed even if they can’t be fully met.
- Requirements must be satisfied; otherwise, the operation will fail.
By understanding the nuances of the authenticatorSelection
parameter, Relying Parties can control the user experience and security characteristics of credential creation within the WebAuthn framework.
Question: Describe the process of selecting an effective user verification requirement for credential creation.
The process of selecting an effective user verification requirement for credential creation is described in step 27 of the [[Create]](origin, options, sameOriginWithAncestors)
method in section 5.1.3 of the WebAuthn Level 3 spec. Here’s a breakdown:
Goal: Determine whether user verification should be performed during credential creation. This depends on both the Relying Party’s preference (expressed in authenticatorSelection.userVerification
) and the authenticator’s capabilities.
Inputs:
- pkOptions.authenticatorSelection.userVerification: The Relying Party’s preference for user verification, which can be one of:
"required"
: The Relying Party insists on user verification."preferred"
: The Relying Party prefers user verification if the authenticator supports it."discouraged"
: The Relying Party does not want user verification.
- Authenticator Capabilities: The client determines whether the selected authenticator is capable of performing user verification.
Algorithm:
- If pkOptions.authenticatorSelection.userVerification is set to “required”:
userVerification
(effective requirement) is set totrue
, meaning user verification MUST be performed.
- If pkOptions.authenticatorSelection.userVerification is set to “preferred”:
- If the authenticator is capable of user verification:
userVerification
is set totrue
.
- If the authenticator is not capable of user verification:
userVerification
is set tofalse
.
- If pkOptions.authenticatorSelection.userVerification is set to “discouraged”:
userVerification
is set tofalse
.
Output:
- userVerification: A boolean value indicating the effective user verification requirement.
In Summary:
- If the Relying Party requires user verification (
"required"
), it will always be performed. - If the Relying Party prefers user verification (
"preferred"
), it will only be performed if the authenticator supports it. - If the Relying Party discourages user verification (
"discouraged"
), it will never be performed.
This process ensures that user verification is performed according to the Relying Party’s preference, while also respecting the limitations of the chosen authenticator.
Question: How does the client handle different attestation conveyance preferences specified by the Relying Party?
Let’s break down how the WebAuthn client handles attestation conveyance preferences, as per the WebAuthn Level 3 spec.
Attestation Conveyance Preference: The Basics
Attestation is a process where an authenticator (like a security key or your phone’s built-in security features) cryptographically vouches for its own authenticity and characteristics. It helps the Relying Party (the website or service you’re logging into) assess the trustworthiness of the authenticator.
The Relying Party can express its preference for how much attestation information it wants to receive using the attestation
field in PublicKeyCredentialCreationOptions
during registration. The possible values (from the AttestationConveyancePreference
enum) are:
- “none” (Default): The Relying Party doesn’t want attestation data.
- “indirect”: The Relying Party wants attestation, but the client can choose how to provide it (potentially using anonymization techniques).
- “direct”: The Relying Party wants the raw attestation statement directly from the authenticator.
- “enterprise”: The Relying Party wants attestation that might include uniquely identifying information (for controlled environments).
Client’s Actions Based on Preference
Here’s how the client, often implemented within a web browser, handles each preference:
- “none”:
- If the authenticator creates an attestation statement:
- Non-Self Attestation: The client replaces the original attestation statement with a “None” attestation statement, stripping away any identifiable information.
- Self Attestation: No action is needed, as self attestation already lacks provenance details.
- “indirect”:
- The client has flexibility here. It can:
- Pass through the authenticator’s attestation statement directly.
- Replace it with a more privacy-friendly or easily verifiable version, perhaps using an Anonymization CA (certificate authority).
- “direct”:
- The client MUST convey the authenticator’s original attestation statement without modification to the Relying Party.
- “enterprise”:
- The client is highly restricted:
- It MUST NOT provide identifying attestation unless specifically permitted by user agent or authenticator configuration for the given Relying Party.
- If permitted, it SHOULD signal to the authenticator that enterprise attestation is desired and convey the received attestation data without changes.
Key Takeaways
- The client acts as a gatekeeper for attestation information, mediating between the authenticator and the Relying Party.
- “none” prioritizes user privacy by minimizing the disclosure of potentially identifiable data.
- “indirect” provides a balance between security (verifiable attestation) and privacy (anonymization options).
- “direct” gives the Relying Party maximum control over attestation evaluation but might expose more user-specific information.
- “enterprise” is for highly controlled scenarios and requires explicit configuration to allow uniquely identifying attestation.
References
Web Authentication: An API for accessing Public Key Credentials – Level 3 (w3.org)
Continue Reading
WebAuthn FAQs – Part 1 – Penfluence
WebAuthn FAQs – Part 2 – Penfluence