Table of Contents
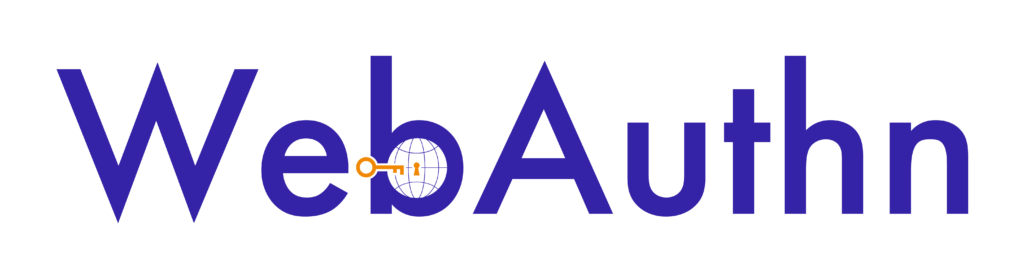
Question: How does the client handle the situation where none of the listed pubKeyCredParams types can be created by the authenticator?
This scenario is handled in step 20 of the [[Create]](origin, options, sameOriginWithAncestors) method, specifically within the “If an authenticator becomes available on this client device” section. Here’s a breakdown of how the client handles it:
- Iterating through
pubKeyCredParams
: The client loops through each item in thepubKeyCredParams
list provided by the Relying Party in thePublicKeyCredentialCreationOptions
. - Authenticator Support Check: For each
PublicKeyCredentialParameters
item, the client checks if thecurrent.type
(credential type, typically “public-key”) andalg
(algorithm identifier, e.g., -7 for ES256) combination is supported by the available authenticator. - Building
credTypesAndPubKeyAlgs
: If atype
andalg
pair is supported by the authenticator, it’s added to a list calledcredTypesAndPubKeyAlgs
. - Empty
credTypesAndPubKeyAlgs
: After checking all items inpubKeyCredParams
, if thecredTypesAndPubKeyAlgs
list remains empty, it means that the authenticator does not support any of the requested credential types and algorithms. - Throw “NotSupportedError”: In this case, the client throws a “NotSupportedError” DOMException, signaling to the Relying Party script that the operation failed because the authenticator does not support the requested parameters.
In Summary: The client proactively checks if the available authenticator supports at least one of the requested pubKeyCredParams
types and algorithms. If none are supported, the credential creation process is terminated with a NotSupportedError
, and the Relying Party is informed that it needs to provide different parameters, use a different authenticator, or handle the error accordingly.
Question: Describe the process of selecting an effective user verification requirement for assertion generation.
The process of selecting an effective user verification requirement for assertion generation in WebAuthn is defined in step 2 of the “Issuing a Credential Request to an Authenticator” algorithm and in step 4 of the authenticatorGetAssertion
operation. It’s a multi-step process that considers both the Relying Party’s preference and the authenticator’s capabilities. Here’s a breakdown:
1. Relying Party’s Preference:
The Relying Party expresses its preference for user verification using the userVerification
option in PublicKeyCredentialRequestOptions
. This option can have three values:
- “required”: The Relying Party strongly requires user verification for the authentication ceremony.
- “preferred”: The Relying Party prefers user verification but will accept an assertion without it if the authenticator doesn’t support it.
- “discouraged”: The Relying Party prefers to avoid user verification if possible.
2. Authenticator’s Capabilities:
The client platform queries the authenticator to determine whether it’s capable of performing user verification. This capability depends on the authenticator’s design and may involve checking for hardware support (e.g., fingerprint sensor) or software features (e.g., PIN entry).
3. Determining the Effective Requirement:
Based on the Relying Party’s preference and the authenticator’s capabilities, the effective user verification requirement is determined as follows:
- If
userVerification
is “required”:- The effective user verification requirement is true (user verification is required).
- If the authenticator is NOT capable of user verification: The client MUST return an error, and the ceremony fails.
- If
userVerification
is “preferred”:- If the authenticator IS capable of user verification: The effective user verification requirement is true (user verification is required).
- If the authenticator is NOT capable of user verification: The effective user verification requirement is false (user verification is not required).
- If
userVerification
is “discouraged”:- The effective user verification requirement is false (user verification is not required).
4. User Interaction:
Once the effective user verification requirement is determined, the authenticator prompts the user for an authorization gesture:
- If user verification is required: The authorization gesture MUST include a user verification method (e.g., fingerprint scan, PIN entry).
- If user verification is not required: The authorization gesture will be a simple test of user presence (e.g., touching a button).
5. Signaling to the Relying Party:
The authenticator sets the UV (User Verified) flag in the authenticatorData
of the assertion response:
- If user verification was performed: The UV flag is set to 1.
- If user verification was NOT performed: The UV flag is set to 0.
Example Scenarios:
- Scenario 1: The Relying Party sets
userVerification
to “required,” and the authenticator supports fingerprint verification. The effective requirement is true, and the user must scan their fingerprint to generate the assertion. The UV flag in the response will be 1. - Scenario 2: The Relying Party sets
userVerification
to “preferred,” and the authenticator only supports a test of user presence. The effective requirement is false. The user touches a button to generate the assertion, and the UV flag will be 0. - Scenario 3: The Relying Party sets
userVerification
to “discouraged,” and the authenticator supports both PIN entry and fingerprint scanning. The effective requirement is false. The user only needs to touch a button (test of user presence) to generate the assertion, and the UV flag will be 0.
In summary, the process aims to balance the Relying Party’s security requirements with the user’s experience by considering both the requested user verification level and the authenticator’s capabilities.
Question: What are the conditions under which the client might substitute an arbitrary sequence for the transports member?
The WebAuthn spec outlines a specific condition where the client might substitute an arbitrary sequence for the transports
member of the AuthenticatorAttestationResponse
. This happens when:
- The user agent does not wish to divulge the authenticator’s transport information. This could be due to privacy concerns, where revealing the exact transport mechanisms supported by an authenticator might be considered sensitive user data.
The specification emphasizes that, even in this case, the substituted sequence MUST still be valid. This means:
- Lexicographically sorted: The transport strings in the sequence must be in alphabetical order.
- Free of duplicates: No transport string should appear more than once in the sequence.
The user agent takes a risk when substituting an arbitrary sequence:
- Relying Party behavior may be suboptimal: The Relying Party might be unable to optimize the user experience based on the available transports. For example, if the actual transport is USB but the arbitrary sequence doesn’t include “usb”, the Relying Party might fail to provide specific instructions for using a USB authenticator.
In essence, the client can substitute the transports
sequence when it prioritizes user privacy over potentially optimal interaction with the Relying Party. This substitution, however, must adhere to the validity constraints to avoid causing errors or unexpected behavior.
Question: What steps should user agents take to ensure correct string handling when interacting with authenticators?
The WebAuthn specification outlines several considerations for user agents regarding string handling during interactions with authenticators. This is crucial because authenticators might have limited resources and may not handle arbitrary strings gracefully. Here’s a breakdown of the steps user agents should take:
1. Understand String Truncation Limits
- The spec defines minimum supported lengths for various strings.
- User agents MUST be aware of these limits for each authenticator they interact with.
- If an authenticator cannot handle a string exceeding its limit, the user agent MUST perform the truncation before sending the data to the authenticator.
2. Truncate Strings Correctly
- When truncating, user agents SHOULD respect UTF-8 sequence boundaries and, ideally, grapheme cluster boundaries [UAX29].
- Truncation at byte boundaries alone can lead to rendering issues or invalid UTF-8 sequences, especially when the authenticator uses CBOR.
3. Validate String Encoding
- User agents SHOULD ensure that all strings sent to authenticators are validly UTF-8 encoded.
- When receiving truncated strings back from authenticators, user agents SHOULD handle potentially invalid encodings, for example by replacing partial code points with U+FFFD (REPLACEMENT CHARACTER).
4. Handle Language and Direction Metadata
- Strings in the WebAuthn API might need to carry language and direction information for correct rendering, especially when displayed on different platforms than where they were created.
- The spec defines a specific method for encoding this metadata directly within the string itself, using code points in the Private Use Area.
- User agents SHOULD be able to encode and decode this metadata, ensuring it’s preserved during truncation.
5. Be Aware of CBOR Limitations
- When dealing with authenticators that use CBOR, user agents MUST be mindful of how truncation affects CBOR string encoding.
- Truncated UTF-8 sequences can result in invalid CBOR data.
Summary
The overarching goal is to maintain a consistent and secure experience for Relying Parties, shielding them from the potential inconsistencies of authenticator string handling. By performing these steps, user agents act as a bridge, ensuring reliable data transfer and preventing errors that could compromise security or functionality.
Question: Explain the purpose and functionality of the isConditionalMediationAvailable() method.
The isConditionalMediationAvailable()
method in the Web Authentication API plays a crucial role in streamlining user experience during WebAuthn authentication ceremonies. It allows Relying Parties (websites or applications requesting authentication) to determine whether the user’s browser supports “conditional user mediation.”
Purpose:
Conditional user mediation aims to minimize user interaction during authentication. Instead of always presenting a prominent modal dialog requiring user action (like choosing an authenticator or providing a biometric scan), the browser can delay this prompt. It waits for a specific trigger, like a user interacting with a designated form field, before requesting the user’s explicit involvement. This reduces unnecessary interruptions when credentials are readily available or can be silently discovered.
Functionality:
The isConditionalMediationAvailable()
method is a static method of the PublicKeyCredential
interface. When invoked, it returns a Promise that resolves to:
true
– if the browser supports conditional user mediation.false
– if conditional user mediation is not supported.
How Relying Parties Use It:
- Checking Availability: Before attempting to use conditional mediation, a Relying Party should call
isConditionalMediationAvailable()
. This ensures they don’t trigger unexpected errors or degraded user experience in browsers that lack support. - Setting
mediation
toconditional
: If the method returnstrue
, the Relying Party can safely set themediation
property of thePublicKeyCredentialRequestOptions
object to'conditional'
when callingnavigator.credentials.get()
. This instructs the browser to use conditional mediation.
Example:
if (!window.PublicKeyCredential) {
/* Client not capable. Handle error. */
}
PublicKeyCredential.isConditionalMediationAvailable()
.then(function (available) {
if (available) {
// Conditional mediation is supported!
var options = {
// ... other authentication options ...,
mediation: 'conditional'
};
navigator.credentials.get({ publicKey: options })
.then(function (assertion) {
// ... handle assertion ...
})
.catch(function (err) {
// ... handle error ...
});
} else {
// Conditional mediation not available, use regular mediation
// ... authentication logic without 'conditional' ...
}
});
In summary:
The isConditionalMediationAvailable()
method is a simple yet powerful tool that enables Relying Parties to create smoother and less intrusive WebAuthn authentication flows, enhancing user satisfaction and convenience.
Question: How do user agents determine the transports supported by a given authenticator?
The WebAuthn spec doesn’t explicitly define how user agents should determine the transports supported by a given authenticator. It leaves this aspect open for client platform-specific implementations, recognizing that different platforms and authenticators will have their own mechanisms for communicating this information.
The spec does offer some suggestions for how user agents could discover this information, including:
- Information from an Attestation Certificate: Some attestation certificates might include extensions indicating the supported transports. For instance, the [FIDO-Transports-Ext] standard allows encoding transport information in attestation certificates.
- Metadata Communicated in an Authenticator Protocol: Protocols like CTAP2 ([FIDO-CTAP]), commonly used for communicating with authenticators, might contain metadata about supported transports. This metadata can be exchanged during the initial handshake or other protocol interactions.
- Special-Case Knowledge about a Platform Authenticator: The user agent might have built-in knowledge about platform authenticators and their capabilities, especially if the user agent and platform authenticator are developed by the same entity (e.g., a browser and platform authenticator developed by the same company).
In essence, the method for determining supported transports is left flexible to accommodate various implementation choices and future advancements in authenticator technology. The key takeaway is that user agents are expected to use some mechanism to gather this information, allowing Relying Parties to receive accurate transport hints via the getTransports()
method, ultimately improving the user experience.
However, the spec also acknowledges that the user agent might not always have access to reliable transport information. In such cases, the spec allows the user agent to:
- Substitute an arbitrary sequence: The user agent can choose to return a sequence of DOMStrings that doesn’t accurately reflect the authenticator’s supported transports. This sequence must still be valid (lexicographically sorted and free of duplicates), but it can be designed to preserve privacy, even if it might lead to suboptimal Relying Party behavior.
- Set the field to the empty sequence: This indicates that the user agent has no transport information available.
Relying Parties are expected to handle both situations gracefully, recognizing that transport information might not always be accurate or available.
Question: What happens if the user exercises a user agent UI option to cancel the registration or authentication process?
Let’s break down what happens when a user cancels a WebAuthn registration or authentication process through the user agent’s UI.
The User Agent’s Role
The user agent (your web browser) acts as a mediator between the website (Relying Party) and the authenticator (e.g., security key, fingerprint reader). It provides the UI that guides the user through the process.
Canceling the Operation
- Cancel Signal: When the user clicks “cancel” or otherwise indicates a desire to stop the process, the user agent sends a cancel signal.
- Authenticator Notification: This signal is sent to the authenticator, instructing it to immediately halt whatever operation it was performing (generating a new key pair or creating an assertion).
- Stopping User Input: The authenticator stops prompting the user for any further input (like a fingerprint scan or PIN) related to the canceled operation.
- Promise Rejection: The user agent’s promise that represented the WebAuthn operation (either
navigator.credentials.create()
for registration ornavigator.credentials.get()
for authentication) is rejected. The rejection typically includes aDOMException
with the name “NotAllowedError”.
Impact on the Website
- Error Handling: The website’s JavaScript code that initiated the WebAuthn process will receive this error. It’s the responsibility of the website developer to handle this gracefully, for example, by:
- Displaying a user-friendly message indicating the operation was canceled.
- Offering alternative authentication methods if appropriate.
- Logging the error for debugging purposes.
Security Implications
- Preventing Information Leaks: The WebAuthn specification is carefully designed to prevent information leaks even when a user cancels. For instance, a malicious website shouldn’t be able to tell the difference between a user canceling and no authenticator being present.
- Maintaining User Control: The cancellation mechanism ensures the user always has the final say in whether a credential is created or used, reinforcing user privacy and security.
Key Points
- User cancellation is a fundamental part of the WebAuthn user experience.
- The specification ensures that cancelation is handled in a way that protects the user’s privacy and prevents security vulnerabilities.
- Website developers should implement robust error handling to manage canceled operations gracefully.
Question: What are the implications of the crossOrigin member of the client data for Relying Parties?
The crossOrigin
member of the client data in WebAuthn plays a crucial role in informing Relying Parties about the context in which a registration or authentication ceremony took place. Specifically, it tells the Relying Party whether the WebAuthn API call originated from within a cross-origin iframe.
Here’s a breakdown of the implications for Relying Parties:
Security Implications:
- UI Redressing/Clickjacking: A key security concern for Relying Parties is the potential for UI redressing (clickjacking) attacks when WebAuthn is used within an iframe. If a malicious website embeds a Relying Party’s WebAuthn-enabled page in an iframe and overlays it with their own deceptive UI, they could trick users into performing unintended actions (like making a purchase or transferring funds). The
crossOrigin
flag helps the Relying Party detect this situation. IfcrossOrigin
is true, the Relying Party knows the request came from an iframe and can take appropriate measures to mitigate the risk of UI redressing. - Subdomain Code Injection: Malicious code running on a subdomain of the Relying Party’s main domain (e.g.,
evil.example.com
on a site with RP IDexample.com
) can potentially exercise WebAuthn credentials scoped to the main domain. If a Relying Party is not expecting cross-origin requests, thecrossOrigin
flag can signal a potential code injection attack.
Mitigations and Policy Decisions:
- Strict Origin Validation: Based on the value of
crossOrigin
, Relying Parties should implement strict origin validation. This means checking both theorigin
andtopOrigin
fields of the client data to ensure they match the expected origins for the ceremony.- If the RP expects only same-origin requests, it should reject any requests with
crossOrigin
set totrue
. - If the RP allows embedding in iframes, it should have a well-defined list of permitted embedding origins and should validate
topOrigin
against this list.
- If the RP expects only same-origin requests, it should reject any requests with
- UI Redressing Prevention: Relying Parties can employ various techniques to protect against UI redressing, such as:
- Using the
X-Frame-Options
HTTP header to prevent embedding in iframes entirely. - Employing the experimental
Intersection Observer v2
API’sisVisible
attribute to detect if their embedded content is fully visible to the user. If not, they could, for example, redirect the user to a full-page version of the content.
- Using the
- Subdomain Security: Relying Parties should carefully consider the security implications of allowing WebAuthn requests from subdomains. If allowing subdomains, they should:
- Never serve untrusted code on allowed subdomains within the scope of their WebAuthn credentials.
- Implement strong Content Security Policy (CSP) rules on subdomains to restrict the execution of potentially malicious scripts.
In Summary: The crossOrigin
member is a valuable piece of information that enables Relying Parties to make informed security and policy decisions related to WebAuthn ceremonies. It highlights potential security risks associated with embedded contexts and guides the implementation of appropriate mitigations.
Question: What are the limitations of using the getPublicKey() operation for accessing credential data?
The getPublicKey()
operation, introduced in WebAuthn Level 2, offers a convenient way for Relying Parties to access the credential public key without parsing the entire CBOR-encoded attestation object. However, it has a significant limitation:
- Limited Algorithm Support: The
getPublicKey()
method can only return a non-null value if the user agent understands the algorithm used for the credential public key. If the Relying Party uses thepubKeyCredParams
option to negotiate a public key algorithm that the user agent doesn’t understand,getPublicKey()
will returnnull
. In such cases, the Relying Party must parse theattestationObject
orauthenticatorData
directly to extract the credential public key.
Here’s a breakdown:
- User agents MUST support the following algorithms:
-7 (ES256)
: Elliptic Curve Digital Signature Algorithm (ECDSA) with SHA-256.-257 (RS256)
: RSA Signature with SHA-256.-8 (EdDSA)
: Edwards-curve Digital Signature Algorithm (EdDSA).
- If a Relying Party chooses an algorithm outside this list:
- The user agent cannot translate the public key into a SubjectPublicKeyInfo format.
getPublicKey()
will returnnull
.- The Relying Party must parse the
attestationObject
orauthenticatorData
themselves.
In summary: While getPublicKey()
simplifies the process for common algorithms, Relying Parties should be aware of its limitations and be prepared to handle cases where direct parsing is required.
Question: Why does the AuthenticatorAssertionResponse structure not include an authData key within the attestationObject?
The AuthenticatorAssertionResponse
structure in WebAuthn does include the authData
key within the attestationObject
, unlike what’s mentioned in the provided spec excerpt. The excerpt seems to contain an error.
Here’s why:
- Purpose of authData: The
authData
(authenticator data) is crucial for verifying the assertion’s authenticity. It includes:- RP ID hash: Ensures the assertion is meant for the correct Relying Party.
- Flags: Indicate user presence, user verification, etc.
- Signature counter: Helps detect cloned authenticators.
- Attested credential data (if attestation is present): Includes AAGUID, credential ID, and credential public key.
- Attestation in Assertions: Even though attestation is primarily associated with credential creation, it can optionally be included in assertions as well. This helps the Relying Party get updated information about the authenticator and the credential.
- Necessity of authData: To properly validate the attestation statement within the
attestationObject
of an assertion, the Relying Party needs the correspondingauthData
.
In summary: The attestationObject
within an AuthenticatorAssertionResponse
should indeed include the authData
key. The statement in the provided spec excerpt appears to be an oversight.
Question: How does the client handle situations where authenticators return unsolicited extensions?
The WebAuthn spec acknowledges that client platforms (browsers, operating systems) might have their own policies regarding extensions. This means they could add extra authenticator extensions or client extensions, even if the Relying Party didn’t explicitly request them in the options.extensions
object.
Here’s how the client handles this, and what the Relying Party needs to do:
Client-side:
- The client platform is free to add its own extensions. The motivation for this might be:
- Enhancing security or privacy.
- Fulfilling platform-specific requirements.
Relying Party-side:
- Be prepared: The Relying Party MUST be able to handle situations where the
clientExtensionResults
or theextensions
inauthData
contain entries for extensions that weren’t in the originaloptions.extensions
. - Policy decision: The Relying Party has two choices:
- Ignore: It can choose to safely ignore the unsolicited extensions. This is often appropriate for extensions that don’t affect core functionality.
- Reject: If the unsolicited extension raises concerns or violates the Relying Party’s security policy, it can choose to reject the attestation or assertion.
Example:
Imagine a client platform always adds a hypothetical “clientLocation” extension to provide coarse geolocation data, even if the Relying Party didn’t ask for it.
- Ignore: If the Relying Party doesn’t use location data, it can simply ignore this extra information.
- Reject: If the Relying Party has a strict policy against using location during authentication, it might choose to reject the entire response because of this unsolicited extension.
In Summary:
The WebAuthn spec allows flexibility for client platforms to add their own extensions. Relying Parties are responsible for having a policy in place to handle these unsolicited extensions, either by ignoring them or rejecting the response based on their security requirements.
Question: What should user agents do when encountering unknown values in enumerations?
According to the WebAuthn Level 3 spec, user agents should generally ignore unknown values in enumerations. The spec explicitly states that user agents MUST ignore unknown values in the following enumerations:
- PublicKeyCredentialType: This enumeration defines the valid credential types.
- AuthenticatorAttachment: This enumeration describes authenticators’ attachment modalities.
- ResidentKeyRequirement: This enumeration describes the Relying Party’s requirements for client-side discoverable credentials.
- AttestationConveyancePreference: This enumeration specifies the Relying Party’s preference regarding attestation conveyance during credential generation.
- AuthenticatorTransport: This enumeration defines hints as to how clients might communicate with a particular authenticator.
- UserVerificationRequirement: This enumeration specifies the Relying Party’s requirements regarding user verification.
- TokenBindingStatus: This enumeration defines the status of the Token Binding protocol.
The spec states that enumerations are defined for documentation and registry purposes. While they provide a standardized set of values, the specification acknowledges that new values may be added in the future.
Therefore, user agents MUST be prepared to encounter unknown values and are instructed to ignore them, ensuring backward compatibility with future versions of the spec. This approach allows for flexibility and extensibility without breaking existing implementations.
In summary, user agents encountering unknown enumeration values should treat the situation as if the corresponding parameter or member was not present. This ensures that the WebAuthn API continues to function correctly and avoids unexpected errors or security vulnerabilities.
Question: What are some examples of error codes that authenticators might return, and how should clients interpret them?
The WebAuthn spec doesn’t define specific error codes for authenticators to return. Instead, it focuses on how the client should interpret the variety of errors that an authenticator might return, grouping them into categories based on the security and privacy implications for the Relying Party.
Here’s a breakdown of error handling and interpretation from the client’s perspective, along with examples of situations that might trigger them:
1. “UnknownError”
- Client Interpretation: A generic error occurred within the authenticator. The client can’t provide specific details to the Relying Party.
- Examples:
- Internal authenticator malfunction.
- An unexpected or unsupported command received from the client.
- Resource exhaustion within the authenticator.
2. “NotSupportedError”
- Client Interpretation: The authenticator does not support the requested operation or capability.
- Examples:
- Requesting an algorithm that the authenticator doesn’t implement.
- Requesting a resident key on an authenticator that can’t store credentials.
- Requesting user verification on an authenticator that doesn’t support it.
3. “NotAllowedError”
- Client Interpretation: The user declined to authorize the operation, or the request violated the authenticator’s security policy. This is the most common error, usually indicating the user canceled the process.
- Examples:
- User refused to provide an authorization gesture (fingerprint, PIN, etc.).
- The authenticator’s internal security policy blocked the operation.
- The request timeout expired before the user interacted with the authenticator.
4. “InvalidStateError”
- Client Interpretation: The requested operation is not possible in the authenticator’s current state, but the user has consented to the operation. The client should let the Relying Party know about this specific error, so it can potentially guide the user to a different authenticator.
- Examples:
- The
excludeCredentials
list in acreate()
operation matches a credential already on the authenticator.
- The
5. Other Errors
- The authenticator might return errors specific to its underlying implementation or protocol.
- Client Interpretation: The client SHOULD NOT expose details of these errors to the Relying Party to avoid potential privacy leaks. These errors SHOULD be treated like “NotAllowedError”.
Important Security Considerations for Clients
- Privacy: To protect the user’s privacy, clients should avoid revealing specific details about authenticator errors to the Relying Party, especially in cases where the user hasn’t explicitly consented.
- Information Leakage: The way a client handles errors should not allow a malicious Relying Party to probe for the presence of specific credentials or identify the authenticator type.
In summary, while the WebAuthn spec doesn’t mandate specific authenticator error codes, it guides clients on how to interpret and handle those errors to ensure user privacy and Relying Party security.
Question: How do authenticators maintain isolation between different authenticator sessions?
The WebAuthn specification dictates that authenticators MUST maintain isolation between different authenticator sessions. This is critical for security, ensuring that one session cannot access or influence the operations of another.
The specification, however, intentionally remains flexible about the how, leaving the specific implementation details to the authenticator developers. They can achieve this isolation through various methods:
- Single Session at a Time: The simplest approach is to allow only one active session at any given time. Any new session request would be blocked until the existing session is terminated. This might be suitable for simpler authenticators with limited resources.
- Session Management: More sophisticated authenticators can employ robust session management mechanisms. This could involve assigning unique identifiers to each session, tracking their state, and enforcing access control based on these identifiers. Resources and operations would be strictly partitioned based on the session they belong to.
Key Points:
- Regardless of the implementation, the end result MUST be that sessions are effectively isolated. One session should not be able to access data, resources, or influence the operations of another.
- The isolation is crucial for security, preventing attacks where a malicious entity attempts to gain access to data or manipulate operations in another user’s session.
Example:
Imagine a user has two browser tabs open, both interacting with the same authenticator. Each tab initiates an authentication ceremony (a session). The authenticator must ensure that the operations in one tab (e.g., selecting a credential, entering a PIN) do not interfere with the other tab’s ceremony. This isolation is essential for preventing cross-session attacks.
Question: What security considerations should authenticators take into account when storing personally identifying information?
The WebAuthn specification outlines some crucial security considerations for authenticators handling personally identifying information (PII):
1. Minimize PII Storage:
- Only What’s Necessary: Authenticators should store the absolute minimum PII required for their functionality. The user handle provided during registration is NOT considered PII and its storage is fine. Additional information like usernames, email addresses, or display names should only be stored if absolutely essential for the user experience and even then, only after successful user verification (see below).
2. User Verification as Gatekeeper:
- No PII without Verification: If an authenticator supports user verification (e.g., PIN, biometrics), it SHOULD NOT expose ANY PII unless the user has successfully verified their identity.
- No Verification, No PII: Authenticators without user verification capabilities SHOULD NOT store ANY PII at all.
3. Protect Against Unauthorized Access:
- Secure Storage: PII, if stored, MUST be protected from unauthorized access using strong cryptographic mechanisms. This means using secure storage elements, tamper-resistant hardware, or robust software encryption.
- Compartmentalization: If an authenticator supports multiple user accounts, PII belonging to different users MUST be isolated and protected from each other. A verified user should never have access to another user’s information.
4. Adhere to User Consent:
- Transparent UI: Any user interface that exposes PII should be clear and unambiguous about what information is being accessed and why.
- User Control: Users should have the ability to control what PII is stored on the authenticator and how it is used. They should be able to review, modify, or delete their data.
Reasoning behind these precautions:
- Data Breaches: Authenticators are potential targets for attackers. Minimizing PII storage reduces the impact of a successful breach.
- Physical Access: A lost or stolen authenticator could be accessed by an adversary. User verification and strong protection mechanisms act as barriers against PII extraction.
- Privacy Abuse: Even legitimate applications could misuse PII. User control and transparent UI empower users to make informed decisions about their data.
Important Note: WebAuthn is designed to minimize the need for authenticators to store PII. Relying Parties should prioritize using user handles for identification and avoid placing sensitive information on the authenticator if possible.
Question: How should authenticators handle situations where they are presented with a credential ID that they cannot decrypt?
The WebAuthn spec allows for two forms of credential IDs:
- Randomly generated IDs: These are at least 16 bytes long with at least 100 bits of entropy.
- Encrypted credential sources: These are the public key credential source (excluding ID and mutable items) encrypted so only the generating authenticator can decrypt it. This allows for near stateless authenticators.
When presented with a credential ID, the authenticator first attempts to decrypt it:
Step 1: Decryption Attempt:
- The authenticator uses its internal decryption mechanisms to see if the credential ID is a validly encrypted credential source. This might involve checking for specific formatting or signatures associated with its encryption scheme.
Step 2: Decryption Success:
- Success: If decryption is successful, the authenticator has recovered the public key credential source. It can proceed with the authentication ceremony using the retrieved information.
Step 3: Decryption Failure:
- Failure: If decryption fails, the authenticator assumes the credential ID is a randomly generated one. It then proceeds to:
- Check its internal database: It searches for a credential source matching the provided ID.
- Proceed if found: If a match is found, the authenticator can proceed with the ceremony.
- Return an error if not found: If no match is found, the authenticator returns an error indicating that it doesn’t recognize the credential ID.
Important Considerations:
- Privacy: The authenticator shouldn’t reveal whether the decryption failed due to an invalid encrypted ID or a non-existent ID. This helps protect user privacy and prevents attackers from probing for credential existence.
- Error Handling: The authenticator’s response should be consistent whether it can’t find a matching credential ID or simply doesn’t recognize the provided ID. This avoids leaking information about the authenticator’s internal workings.
In summary: When presented with a credential ID, the authenticator first attempts decryption. If successful, it uses the recovered information. If decryption fails, it treats the ID as a randomly generated one and searches its database for a match. The key is to handle both success and failure discreetly to maintain user privacy and security.
Question: What are the implications of an authenticator supporting both client-side and server-side credential storage modalities?
You’re right to hone in on that detail! Here’s a breakdown of the implications when an authenticator supports both client-side (discoverable credentials) and server-side credentials:
From the Authenticator’s Perspective:
- Flexibility: The authenticator offers greater versatility, catering to both Relying Parties who prioritize discoverable credentials (for streamlined UX) and those who prefer server-side storage (for potentially enhanced security or control).
- Complexity: The authenticator’s internal logic and storage mechanisms become more intricate. It needs to manage two distinct credential types, each with its own set of requirements.
- Resource Management: If the authenticator has limited storage, it needs to carefully allocate space, potentially prioritizing one credential type over the other based on user preference, Relying Party requirements, or other factors.
From the Relying Party’s Perspective:
- Choice and Control: The Relying Party gains the freedom to choose the most suitable credential storage modality for its specific use cases and security model. They can use
authenticatorSelection.residentKey
during registration to express their preference. - Attestation Considerations: The Relying Party needs to be aware that a single authenticator model may generate credentials with different levels of attestation trust. A discoverable credential generated by the same authenticator might have weaker attestation than a server-side credential, especially if the authenticator is relying on “self attestation” for discoverable credentials.
- Registration Flow Logic: The Relying Party’s registration process may need to become more complex to accommodate both credential types. For example, they might need to handle different error cases if a discoverable credential cannot be created due to lack of user verification.
From the User’s Perspective:
- Potential for Confusion: If an authenticator can create both discoverable and server-side credentials, the user might need to make choices during registration that they don’t fully understand. Clear, user-friendly explanations from the client platform and the Relying Party are crucial.
- Impact on Backup and Recovery: The user needs to be aware that backing up and recovering credentials may work differently for discoverable and server-side credentials, depending on the mechanisms employed by the authenticator and the Relying Party.
In Summary:
Support for both credential storage modalities empowers both authenticator manufacturers and Relying Parties, giving them more options to tailor WebAuthn to specific needs. However, it also introduces some complexities that need to be carefully addressed to maintain security, user privacy, and a smooth user experience.
Question: How should authenticators handle the situation where the user does not consent to the creation of a new credential?
According to the WebAuthn spec, when the user does not consent to the creation of a new credential, the authenticator MUST:
- Abort the Operation: Immediately stop any ongoing processes related to credential creation.
- Return an Error: Return an error code equivalent to “NotAllowedError” to the client platform.
This ensures that:
- User Control is Maintained: The user has ultimate authority over whether a new credential is generated on their authenticator.
- Privacy is Preserved: The Relying Party doesn’t get any information about why the operation failed, preventing potential information leaks that could identify the user.
- Consistent Client Behavior: The client platform can consistently handle user rejections across different authenticator implementations.
Example:
Imagine a user visits example.com
and the website wants to register a new security key. The browser initiates the registration ceremony and prompts the user for authorization on their security key.
If the user decides not to register with the site, they would decline consent on their authenticator. The authenticator would then abort the process and signal the “NotAllowedError” to the browser. The browser would then relay that error to the website, which would need to handle it appropriately (e.g., by displaying a message to the user).
Important Notes:
- The “NotAllowedError” is deliberately generic. It doesn’t reveal whether the user explicitly denied consent or if there was another issue (e.g., a timeout).
- This behavior ensures the user isn’t unknowingly enrolled with a Relying Party.
- Relying Parties should provide clear and understandable prompts to users so they can make informed decisions about consent.
Question: Explain the process of incrementing signature counters within the authenticatorGetAssertion operation.
Let’s break down how signature counters work within the authenticatorGetAssertion
operation of WebAuthn.
Purpose of Signature Counters
Signature counters are a crucial security mechanism in WebAuthn designed to detect cloned or compromised authenticators. Think of it as a tamper-evident seal. Each time a credential is successfully used for authentication, its associated counter increases. This allows the Relying Party (website/service) to check if the counter value in an assertion (authentication response) is logically consistent with previous uses.
Counter Incrementing During authenticatorGetAssertion
- Operation Triggered: When a user tries to authenticate with a WebAuthn credential, the Relying Party’s server initiates the
authenticatorGetAssertion
operation on the user’s authenticator. - Counter Increment: Upon successful user verification and authentication, the authenticator will:
- Per-Credential Counters (Recommended): If the authenticator supports individual signature counters for each credential, it finds the counter associated with the credential being used and increments it by a positive value (not necessarily 1, but always increasing).
- Global Counter (Less Privacy-Friendly): If the authenticator uses a single counter for all credentials, it increments this global counter.
- Authenticator Data: The incremented counter value is included in the ‘authenticatorData’ structure within the authenticator’s response.
Relying Party Verification
- Stored Value: The Relying Party’s server stores the signature counter from the last successful authentication attempt for that credential. If this is the first authentication, it might store the initial counter value provided during registration.
- Comparison: The server receives the new
authenticatorData
with the updated counter. It compares the received counter value with its stored value. - Validation:
- Consistent: If the new counter is greater than the stored counter, everything checks out, and the authentication proceeds.
- Cloning Suspected: If the new counter is less than or equal to the stored value, this raises a red flag. It indicates that a cloned authenticator might be in use or that the authenticator is malfunctioning. The Relying Party needs to make a risk-based decision on how to handle this (e.g., block the authentication, require additional verification).
Important Notes
- No Decrement: Authenticators should ensure counters never decrease in value to prevent attackers from manipulating them.
- Not Foolproof: Counter-based clone detection is not a silver bullet. Sophisticated attackers might manipulate counter values or exploit vulnerabilities in an authenticator’s implementation.
- FIDO U2F Compatibility: WebAuthn’s counter scheme is designed to be compatible with FIDO U2F, which typically uses a global counter.
In Summary
The signature counter incrementing process in authenticatorGetAssertion
is a vital part of WebAuthn’s security, adding an extra layer of defense against attacks targeting authenticators. While it’s not perfect, it significantly enhances the security of user authentication.
Question: What steps should authenticators take to ensure that signature counter values do not accidentally decrease?
The WebAuthn specification strongly recommends that authenticators implement signature counters to help Relying Parties detect cloned authenticators. It also states that authenticators SHOULD ensure that the signature counter value does not accidentally decrease (e.g., due to hardware failures).
Here’s a breakdown of steps authenticators should take to ensure signature counters don’t decrease:
1. Secure Storage:
- Dedicated Storage: The signature counter should be stored in a dedicated, secure area of the authenticator. This could be within a Secure Element, Trusted Platform Module (TPM), or a protected memory region with appropriate access controls.
- Redundancy: Employing redundant storage mechanisms can help mitigate the risk of data loss due to single-point failures. For instance, mirroring the counter value in multiple non-volatile memory locations.
- Error Correction: Use error correction techniques (like checksums or ECC) to detect and potentially correct corruption in the stored counter value.
2. Atomic Updates:
- Transaction Mechanisms: Implement atomic update operations for the signature counter. This ensures that if an update is interrupted (e.g., power loss), the counter is either incremented fully or not at all, preventing an invalid intermediate value from being stored.
- Journaling: Consider using journaling techniques where changes to the counter are first written to a log. This log can be used to recover the counter value to a consistent state if an update operation is incomplete.
3. Power Loss Protection:
- Power-Fail Detection: Implement mechanisms to detect power failures.
- Graceful Shutdown: Upon detecting a power failure, the authenticator should attempt a graceful shutdown, writing the current counter value to secure storage before power is fully lost.
- Supercapacitors/Battery Backup: Consider using supercapacitors or small battery backups to provide enough power for the authenticator to complete the counter write operation during a power failure.
4. Monotonic Counter Implementation:
- Hardware-based Counters: Some hardware platforms offer specialized hardware counters designed to be monotonic (always increasing). Utilize these if available.
- Software Monotonicity: If using a software counter, ensure its implementation enforces strict monotonicity. This means even in the event of unexpected errors or rollbacks, the counter should never be set to a value lower than its current value.
5. Testing and Validation:
- Rigorous Testing: Conduct extensive testing of the counter implementation under various failure scenarios (power loss, memory corruption, software bugs, etc.).
- Formal Verification: For high-security authenticators, consider formal verification methods to mathematically prove the correctness of the counter implementation.
Important Considerations:
- Authenticator Type: The complexity of counter protection mechanisms might vary based on the type of authenticator. For instance, a high-security hardware token will have stronger safeguards compared to a software-based authenticator on a mobile phone.
- Attestation: Attestation mechanisms should be used to inform Relying Parties about the specific counter protection measures employed by the authenticator, enabling them to make informed trust decisions.
By following these steps, authenticators can significantly reduce the risk of signature counter values decreasing, strengthening the security of WebAuthn deployments.
Question: How do authenticators handle the situation where they are presented with a requireResidentKey value of true but are incapable of client-side credential storage?
This scenario is addressed in step 3 of the authenticatorMakeCredential operation, as defined in section 6.3.2 of the WebAuthn spec.
Here’s the breakdown:
- Context: The Relying Party has requested a client-side discoverable credential (formerly known as a resident key) by setting
authenticatorSelection.residentKey
torequired
in thePublicKeyCredentialCreationOptions
. This means the RP wants a credential that can be discovered and used without the RP providing a list of potential credential IDs. - Authenticator Limitation: The authenticator receiving this request is incapable of storing credentials client-side. This could be due to various reasons, like limited storage or a design that relies on server-side credential management.
- Authenticator Action: The spec mandates that in this situation, the authenticator MUST return an error code equivalent to
"ConstraintError"
. This signals to the client (browser) that the authenticator cannot fulfill the specific requirements of the request. - Client Response: The client, upon receiving the
"ConstraintError"
, will understand that this specific authenticator is not suitable for the operation. The client may then either:- Try another authenticator: If other authenticators are available on the client platform, the client may attempt to use one that is capable of client-side credential storage.
- Inform the user and fail: If no suitable authenticator is found, the client will typically inform the user that the requested operation cannot be completed and abort the registration process.
In summary, authenticators gracefully handle the mismatch between the requireResidentKey
requirement and their own capabilities by returning a specific error, allowing the client to take appropriate action. This ensures a smooth user experience and prevents unexpected behavior or security vulnerabilities.
Question: How should authenticators determine which attestation statement format to use for a given operation?
The WebAuthn specification outlines how authenticators should determine the appropriate attestation statement format to use. Here’s a breakdown of the process, combining information from various sections of the spec:
1. Relying Party Preference (Advisory):
- The Relying Party can express its preference for attestation statement formats using the
attestationFormats
parameter in bothPublicKeyCredentialCreationOptions
(for registration) andPublicKeyCredentialRequestOptions
(for authentication). - This parameter is an array of strings, listing desired formats in order from most to least preferred.
- Important: This is merely a suggestion. Authenticators are not obligated to strictly follow this preference.
2. Authenticator Capabilities:
- Authenticators have a limited set of attestation statement formats they support.
- The specific formats available depend on the authenticator’s hardware, software, and security features.
3. Enterprise Attestation:
- If the Relying Party sets the
attestation
parameter to"enterprise"
, the authenticator should prioritize formats that may include uniquely identifying information, provided the user agent or authenticator configuration permits this for the RP ID.
4. Authenticator’s Choice:
- Ultimately, the authenticator makes the final decision on the format, considering:
- The Relying Party’s preferences (if any).
- The authenticator’s supported formats.
- Whether enterprise attestation is permitted.
- If the Relying Party’s
attestationFormats
doesn’t contain any format the authenticator supports, it will default to its most preferred format.
5. No Attestation Case:
- If the Relying Party sets
attestation
to"none"
, the authenticator MUST NOT return an attestation statement. The client will replace any authenticator-generated statement with a"none"
attestation statement.
Example:
- A Relying Party requests
"tpm"
and"packed"
formats (in that order). - The authenticator supports
"packed"
,"fido-u2f"
, and"android-key"
formats. - The authenticator would likely choose
"packed"
, as it’s the most preferred format it supports.
Key Points:
- The authenticator’s choice is influenced by a combination of factors.
- Relying Parties should be prepared to handle any supported attestation format, even if it wasn’t their top preference.
- Attestation is primarily a mechanism for the Relying Party to assess the trustworthiness of the authenticator and the credential, not a strict identification process.
Question: What considerations should authenticators take into account when selecting a randomness generation mechanism for the randomNonce in the “devicePubKey” extension?
The randomNonce
in the devicePubKey
extension plays a crucial role in enhancing the security of attestation signatures. Its primary purpose is to introduce randomness into the signature value, mitigating potential side-channel attacks targeting the authenticator’s attestation private key.
Here’s a breakdown of considerations for authenticator developers when choosing a randomness generation mechanism for the randomNonce
:
1. True Randomness vs. Pseudorandomness:
- True Random Number Generators (TRNGs): These rely on physical processes to generate unpredictable data. TRNGs are generally considered more secure but can be slower and more resource-intensive. If available in the authenticator’s hardware, a TRNG is the ideal source for the
randomNonce
. - Pseudorandom Number Generators (PRNGs): PRNGs use algorithms to produce sequences of numbers that appear random but are ultimately deterministic. Their security relies on the quality of the initial seed and the algorithm used.
2. Seed Quality for PRNGs:
- If using a PRNG, ensure the initial seed is derived from a truly random source. This could be:
- Output from a hardware TRNG.
- Timestamps combined with other unpredictable system parameters (e.g., CPU temperature, system load).
- Dedicated hardware entropy sources available on some platforms.
- Avoid using easily predictable values like the current time alone.
3. Algorithm Strength for PRNGs:
- Use a cryptographically secure PRNG algorithm with well-established properties. Common examples include:
- HMAC-DRBG: Based on a cryptographic hash function.
- CTR-DRBG: Uses a block cipher in counter mode.
- Avoid weak PRNGs that might produce predictable sequences.
4. Timing Attacks:
- Be aware of potential timing side channels. The time taken to generate the
randomNonce
should not leak information about the underlying random values or the authenticator’s internal state.- Use constant-time operations if possible.
- Implement appropriate countermeasures to minimize timing variations.
5. Standards Compliance:
- If using a PRNG, consider algorithms recommended by relevant security standards (e.g., NIST SP 800-90A). This helps ensure a certain level of security assurance.
6. Authenticator Capabilities:
- Choose a mechanism that aligns with the authenticator’s hardware and software capabilities. TRNGs might not be available on all devices, and PRNGs may vary in efficiency depending on the platform.
7. Entropy Pool Management (if applicable):
- If the authenticator maintains an entropy pool, ensure it is properly managed to prevent depletion and maintain a sufficient level of randomness.
8. Attacker Model:
- Consider the capabilities of potential attackers. If the authenticator is susceptible to physical tampering or sophisticated side-channel analysis, choose a mechanism with a higher level of security.
9. Security Reviews:
- Subject the chosen randomness generation mechanism to thorough security reviews to identify potential vulnerabilities.
In Summary:
The key is to generate a randomNonce
that is genuinely unpredictable by an attacker, even if they have knowledge of previous nonces. Prioritize true randomness from a hardware source whenever possible. If using a PRNG, ensure strong seed generation and algorithm selection. Consult cryptography experts and security standards for guidance when making these critical decisions.
Question: What are the different scopes for device-bound keys, and how do they impact their usage?
The WebAuthn specification defines two scopes for device-bound keys, created via the devicePubKey
extension:
- “Entire Device” scope (value 0x00): This is the broader scope, indicating the device-bound key is tied to the entire device. It’s expected to be consistent across different applications (e.g., a browser and a native app) on the same device.
- Impact: Relying Parties can use this scope to gain a stronger device continuity signal. For example, if a user registers with a web application in a browser and later authenticates using a native app on the same device, the same device-bound key will be used, signaling continued usage of the same device.
- “Per-app” scope (value 0x01): This is a narrower scope, indicating the device-bound key is tied to a specific application. It’s expected to be different for different applications on the same device.
- Impact: Relying Parties can use this scope for cases where device continuity is desired only within the context of a specific application. For example, a native app might use this scope to ensure that the same device is used for all interactions with that specific app, but this wouldn’t impact authentication on other apps or websites.
Choosing the Right Scope:
The choice between these scopes depends on the Relying Party’s security and user experience goals:
- “Entire device” scope is preferred when the Relying Party wants a stronger device continuity signal across all applications on the user’s device. This can be useful for risk assessment and fraud prevention.
- “Per-app” scope is preferred when the Relying Party is concerned about potential privacy implications of tying a key to the entire device and only needs device continuity within a specific application context.
Important Considerations:
- Attestation: Regardless of the scope, the device-bound key’s attestation statement provides information about the authenticator’s security properties and can help the Relying Party assess the trustworthiness of the device continuity signal.
- Privacy: Relying Parties should be mindful of the privacy implications of using device-bound keys, especially those with “entire device” scope. These keys can potentially be used to track users across different applications and websites, so their usage should be transparent and justified.
- User Experience: The scope can impact the user experience. For example, if a user has multiple applications that use device-bound keys with “per-app” scope, they might be prompted for user verification multiple times on the same device.
By carefully considering these factors, Relying Parties can choose the appropriate scope for device-bound keys to enhance their security posture while maintaining user privacy and providing a seamless user experience.
Question: How do authenticators handle the situation where they are requested to provide “enterprise” attestation but are not configured to do so?
According to the WebAuthn Level 3 spec, when an authenticator receives a request for “enterprise” attestation but is not configured to provide it, it MUST NOT return an attestation statement that includes uniquely identifying information.
Here’s a breakdown of the behavior:
- Request received: The authenticator receives the
authenticatorMakeCredential
orauthenticatorGetAssertion
operation with the “enterprise” attestation preference signaled. - Configuration check: The authenticator checks its internal configuration or relies on a signal from the user agent to determine if it is allowed to provide uniquely identifying attestation for the requested RP ID.
- Not permitted: If enterprise attestation is not permitted:
- Attestation format: The authenticator sets the attestation format to “none”.
- AAGUID: The AAGUID is set to 16 zero bytes. This ensures no specific hardware identification is revealed.
- Result: The authenticator returns a standard response without any uniquely identifying information in the attestation object.
Key takeaway: The spec emphasizes user privacy, especially in enterprise scenarios. Even when explicitly requested, an authenticator will not provide identifying information unless explicitly configured to do so for the specific Relying Party. This approach prevents accidental leakage of sensitive data.
Practical Implications:
- Relying Parties: Relying Parties requesting “enterprise” attestation should be aware that it might not always be available. They should design their systems to handle scenarios where the authenticator returns a non-identifying attestation.
- Authenticator Developers: When designing authenticators for enterprise use, ensure clear and secure mechanisms are in place to configure which Relying Parties are allowed to receive identifying attestations.
This mechanism balances the need for stronger identity assurance in enterprise settings with the paramount importance of user privacy.
Question: What happens if an error occurs during the generation of a new credential object within the authenticatorMakeCredential operation?
Let’s break down what happens when an error occurs during credential object generation within the authenticatorMakeCredential
operation, as described in the WebAuthn spec.
The authenticatorMakeCredential
operation
This is the core operation for registering a new WebAuthn credential with an authenticator. The client (e.g., your web browser) initiates this operation on a chosen authenticator (like a security key or built-in platform authenticator) based on a Relying Party’s request.
The Critical Step Where Errors Can Occur
After the user consents to create the credential, the authenticator begins creating a new credential object. This process involves:
- Key Pair Generation: Generating a new public-private key pair (
publicKey
,privateKey
). - Credential Source Creation: Constructing a
credentialSource
structure holding essential data like:type
: “public-key”privateKey
: The newly generated private keyrpId
: The Relying Party IDuserHandle
: User identifier provided by the Relying PartyotherUI
: Any additional UI-related info the authenticator wishes to include
- Credential ID and Storage:
- If a client-side discoverable credential is requested or the authenticator chooses to create one:
- A new, random
credentialId
is generated. - The
credentialId
is assigned tocredentialSource.id
. - The
credentialSource
is stored in the authenticator’s credentials map, keyed by (rpId
,userHandle
).
- A new, random
- Otherwise (server-side credential):
- The
credentialSource
is serialized and encrypted, with the resulting ciphertext becoming thecredentialId
. This allows for server-side storage of the credential.
- The
- If a client-side discoverable credential is requested or the authenticator chooses to create one:
What Happens If an Error Occurs
If any error happens during the above steps, the authenticator:
- Returns an error code: Specifically, an error code equivalent to “UnknownError” is returned to the client.
- Terminates the operation: The
authenticatorMakeCredential
operation is immediately stopped. No new credential is created or registered.
Example Errors
- Key generation failure: The cryptographic module within the authenticator might be unable to generate a key pair.
- Storage errors: The authenticator might encounter problems storing the new
credentialSource
data (e.g., insufficient storage space, internal data corruption). - Encryption issues: If creating a server-side credential, errors during serialization or encryption of the
credentialSource
would lead to this error.
Client’s Response to “UnknownError”
When the client receives the “UnknownError” signal from the authenticator:
- Ceremony Aborting: The client will likely abort the registration ceremony.
- User Feedback: The client may display a generic error message to the user, indicating that credential creation failed.
- Error Handling: The Relying Party’s script can catch this error and implement appropriate error handling (e.g., retrying, prompting the user to try a different authenticator).
Key Points
- Credential object generation is a crucial step in
authenticatorMakeCredential
. - Errors at this stage indicate a serious problem within the authenticator.
- The “UnknownError” code hides the specific cause from the Relying Party to prevent potential security leaks.
- Relying Parties should be prepared to handle this error gracefully.
Question: How do authenticators handle the situation where they cannot find any matching credentials during the authenticatorGetAssertion operation?
This question is addressed directly in the last paragraph of section 6.3.3 of the WebAuthn Level 3 spec:
If the authenticator cannot find any credential corresponding to the specified Relying Party that matches the specified criteria, it terminates the operation and returns an error.
Let’s break down what this means in practical terms:
- No Matching Credentials: The authenticator has received a
getAssertion
request, but after examining its internal store, it can’t find any credentials that match all the criteria provided:
- RP ID: The Relying Party identifier must match.
- Credential ID List (Optional): If the
allowCredentials
list is provided, the credential ID must be included in it. - User Verification: The authenticator must be capable of fulfilling the
userVerification
requirement (discouraged, preferred, or required).
- Termination & Error: The authenticator immediately stops the
getAssertion
operation and signals back to the client (browser or OS) that the request failed. It does not proceed to prompt the user for authorization. - Error Handling: The WebAuthn API specification intentionally avoids defining specific error codes. This is left up to the individual authenticator implementation and its underlying protocols (e.g., CTAP). The important point is that the client platform will receive an indication of failure.
- Client’s Response: The client, in turn, will reject the
navigator.credentials.get()
promise. This allows the Relying Party’s JavaScript code to handle the authentication failure. The browser typically will not give specific reasons for the failure to the website to prevent information leaks.
In essence, the authenticator fails gracefully without giving away any details about its credential store to an unauthorized caller. This helps maintain user privacy.
Question: What are the implications of using a global signature counter instead of a per-credential signature counter?
You’re right to ask about the implications of using a global signature counter! It’s a privacy consideration that often gets overlooked. Here’s a breakdown of why per-credential counters are preferred and what using a global counter could mean:
Global Signature Counter
- How it works: The authenticator maintains a single counter that increments with every successful assertion, regardless of which credential was used.
- The problem: The counter value is shared across all credentials on that authenticator. This means that multiple Relying Parties observing assertions from the same authenticator can potentially correlate the user’s activity across those different services.
Per-Credential Signature Counter
- How it works: Each credential stored on the authenticator has its own independent counter that only increments when that specific credential is used.
- The benefit: The counter value is unique to each credential and thus to each Relying Party. This prevents cross-site correlation based on counter values.
Implications of Global Counters
- User Tracking: A malicious Relying Party could potentially track a user’s activity across multiple websites by observing the incrementing global counter value. Even if usernames are different, the counter can link activity together.
- Fingerprinting: The global counter value, combined with other information like the authenticator’s AAGUID, can contribute to a more unique browser fingerprint. This fingerprint can be used to track users even if they clear cookies or use privacy-enhancing browser features.
Why Per-Credential is Preferred
- Enhanced Privacy: By isolating the counter to individual credentials, user activity is compartmentalized, making it much harder to track a user across different websites.
- Alignment with Privacy Principles: Per-credential counters are more aligned with the privacy goals of WebAuthn and the FIDO Alliance, which prioritize user control and data minimization.
Important Notes:
- Authenticator Choice: The choice of using a global or per-credential counter is up to the authenticator manufacturer. The WebAuthn specification recommends per-credential counters, but does not mandate them.
- Relying Party Awareness: Relying Parties should be aware of this difference and factor it into their risk assessments and user privacy policies.
In summary: While global counters might simplify some aspects of authenticator implementation, they pose a privacy risk by enabling cross-site user correlation. Per-credential counters offer a more privacy-preserving approach, making it harder for malicious actors to track users.
Question: What is the purpose of the WebAuthn WebDriver extension capability?
The WebAuthn WebDriver extension capability serves two main purposes:
- Advertisement: It allows WebDriver endpoints (like browsers or browser drivers) to advertise their support for the WebAuthn extension commands. This way, testing frameworks and tools know whether they can leverage these commands for automating WebAuthn interactions.
- Capability Matching: When a testing framework or tool requests a WebDriver session with specific capabilities, including the WebAuthn extension capability, the endpoint uses this capability to determine if it can fulfill that request. If the endpoint doesn’t support the necessary commands, the session request will be rejected, preventing tests from running on incompatible environments.
Essentially, the WebAuthn WebDriver extension capability acts as a flag that signals the presence of features designed for testing WebAuthn implementations. It ensures that tests are executed only on platforms that can properly simulate and interact with WebAuthn authenticators.
Question: Describe the properties of a Virtual Authenticator in the context of user agent automation.
Virtual Authenticators, used in user agent automation for testing WebAuthn implementations, mirror the behavior of real authenticators but exist only in software. Here’s a breakdown of their key properties:
1. Identification & Protocol:
- authenticatorId: A unique string identifier for the virtual authenticator. This allows WebDriver to reference and control specific virtual authenticators.
- protocol: Defines the version of the Client to Authenticator Protocol (CTAP) that the virtual authenticator emulates. Options include:
"ctap1/u2f"
: For legacy FIDO U2F compatibility."ctap2"
: The standard WebAuthn/FIDO2 protocol."ctap2_1"
: A newer version of CTAP with additional features.
2. Physical Characteristics (Simulation):
- transport: Simulates the physical connection method of the authenticator:
"internal"
: Mimics a platform authenticator (built into the device)."usb"
,"nfc"
,"ble"
,"hybrid"
,"smart-card"
: Simulate various types of roaming authenticators.
3. Capabilities:
- hasResidentKey:
true
if the virtual authenticator supports client-side discoverable credentials (also known as resident keys or passkeys),false
otherwise. - hasUserVerification:
true
if the virtual authenticator can perform user verification (e.g., via PIN or biometrics),false
otherwise.
4. User Interaction (Simulated):
- isUserConsenting: Controls whether the virtual authenticator will automatically grant or deny user consent for operations.
true
: Consent is always granted.false
: Consent is always denied.- isUserVerified: Determines the outcome of user verification attempts.
true
: User verification always succeeds.false
: User verification always fails.- Note: This only applies if
hasUserVerification
istrue
.
5. Extensions:
- extensions: A list of WebAuthn extension identifiers that the virtual authenticator supports. This allows for testing of specific extension behaviors.
- uvm: If the “User Verification Method” (
uvm
) extension is supported, this property stores a set of pre-defined user verification methods that the virtual authenticator will return.
Important Notes:
- Database: Virtual Authenticators are managed within a Virtual Authenticator Database controlled by the WebDriver implementation.
- Testing Flexibility: These properties provide granular control over the behavior of the virtual authenticator, enabling developers to simulate various scenarios and edge cases in their testing.
- Realism: While Virtual Authenticators provide a powerful testing tool, they cannot fully replicate the security characteristics of real-world authenticators.
Question: Explain the steps involved in the “Add Virtual Authenticator” WebDriver extension command.
The “Add Virtual Authenticator” command is part of the WebAuthn WebDriver extension, enabling automated testing of web applications using virtualized authenticators. Here’s a breakdown of the steps involved:
1. HTTP Request:
- Method:
POST
- URI Template:
/session/{session id}/webauthn/authenticator
- Payload: The payload of the request is a JSON object called
Authenticator Configuration
. It defines the characteristics of the virtual authenticator being created.
2. Authenticator Configuration:
The Authenticator Configuration
object has the following keys, allowing you to customize the virtual authenticator:
protocol
: (string, required) Specifies the version of the Client to Authenticator Protocol (CTAP) the virtual authenticator will use. Valid values are"ctap1/u2f"
,"ctap2"
, or"ctap2_1"
.transport
: (string, required) Simulates the authenticator’s communication method. Use values from theAuthenticatorTransport
enumeration:"usb"
,"nfc"
,"ble"
,"smart-card"
,"hybrid"
, or"internal"
."internal"
simulates a platform authenticator.hasResidentKey
: (boolean, default:false
) Determines if the virtual authenticator supports discoverable credentials (also known as resident keys).hasUserVerification
: (boolean, default:false
) Determines if the virtual authenticator has a mechanism for user verification (e.g., PIN, biometrics).isUserConsenting
: (boolean, default:true
) Sets the default outcome for user consent prompts. Iftrue
, prompts will always succeed.isUserVerified
: (boolean, default:false
) Sets the default outcome for user verification. Iftrue
, user verification will always succeed. This is only relevant ifhasUserVerification
is set totrue
.extensions
: (string array, default: empty array) An array of strings listing the extension identifiers supported by the virtual authenticator.uvm
: (UvmEntries, default: empty array) Configures the User Verification Method extension output. Only has an effect if the “uvm” extension is included in theextensions
array.
3. Remote End Steps (WebDriver Server):
- Parameter Validation: The WebDriver server receives the
Authenticator Configuration
object and validates its structure and contents.- If
parameters
isn’t a JSON object, return a “WebDriver error” with code “invalid argument”. - For each key in
parameters
:- If the key is unrecognized, return “invalid argument” error.
- If the value associated with the key is invalid (wrong type, outside allowed range, etc.), return “invalid argument” error.
- If
- Virtual Authenticator Creation:
- A new
Virtual Authenticator
object is created. - Properties from the
Authenticator Configuration
are assigned to theVirtual Authenticator
. - For any missing properties in the
Authenticator Configuration
, defaults are applied. - The server checks that all required properties are now set on the
Virtual Authenticator
. If any are missing, return an “invalid argument” error.
- A new
- Extension Support Validation:
- The server iterates through the
extensions
array of the virtual authenticator. - If an extension identifier is not supported by the server’s WebAuthn WebDriver implementation, it returns a “WebDriver error” with code “unsupported operation”.
- The server iterates through the
- Authenticator ID Generation:
- A unique
authenticatorId
string is generated for the new virtual authenticator. This ID must adhere to rules specified in [RFC3986], Appendix A (unreserved characters).
- A unique
- Storing the Virtual Authenticator:
- The
Virtual Authenticator
, along with its ID, is stored in theVirtual Authenticator Database
, which manages all virtual authenticators for the current testing session.
- The
4. Response:
- Success: If all the steps are successful, the server returns a “success” response with the
authenticatorId
as data.
Summary:
The “Add Virtual Authenticator” command provides fine-grained control over the behavior of virtual authenticators during testing. This allows you to test your web application’s WebAuthn integration under various scenarios, simulating different authenticator types, capabilities, and potential user interactions.
Question: How does the “Remove Virtual Authenticator” command work?
The “Remove Virtual Authenticator” command is part of the WebAuthn WebDriver extension. It’s used for testing WebAuthn implementations in automated browser environments.
Here’s a breakdown of how it works:
- Command Structure:
- HTTP Method: DELETE
- URI Template:
/session/{session id}/webauthn/authenticator/{authenticatorId}
- Parameters:
{authenticatorId}
: This is a unique identifier for the virtual authenticator that you want to remove. It was previously generated and returned when you created the virtual authenticator using the “Add Virtual Authenticator” command.
- What it Does:
- Location Check: The command first checks if the provided
{authenticatorId}
exists within the Virtual Authenticator Database. This database is a temporary storage mechanism managed by the WebDriver implementation where the virtual authenticators are kept. - Removal: If the
authenticatorId
is found, the command removes the corresponding Virtual Authenticator from the database. This means any credentials and settings associated with that virtual authenticator are also deleted. - Success: Upon successful removal, the command returns a success message.
- Error Handling:
- If the provided
{authenticatorId}
does not match any existing Virtual Authenticator, the command returns a WebDriver error with the error codeinvalid argument
.
In Summary: The “Remove Virtual Authenticator” command provides a way to clean up test environments by deleting virtual authenticators that are no longer needed. It ensures your test cases start with a clean slate and prevents potential conflicts between tests that might use the same authenticatorId
.
Question: Describe the functionality of the “Add Credential” and “Get Credentials” commands.
Let’s break down the functionality of the “Add Credential” and “Get Credentials” WebDriver extension commands, used for testing WebAuthn implementations.
1. Add Credential Command
- Purpose: This command injects a simulated public key credential source directly into an existing Virtual Authenticator. This is vital for setting up controlled test scenarios without relying on real physical authenticators.
- Mechanism:
- You provide a JSON object called “Credential Parameters” with details about the credential you want to add:
credentialId
: The unique ID for the credential.isResidentCredential
: Determines if it’s a client-side discoverable credential (like a passkey) or a server-side credential.rpId
: The Relying Party ID (website domain) this credential is associated with.privateKey
: The credential’s private key.userHandle
: An optional identifier linking the credential to a user account.signCount
: A counter that tracks how many times the credential has been used.largeBlob
: Optional data to be stored with the credential.
- The WebDriver implementation validates the provided parameters, ensures they are compatible with the Virtual Authenticator’s capabilities, and then stores the new credential in the Virtual Authenticator’s database.
- You provide a JSON object called “Credential Parameters” with details about the credential you want to add:
2. Get Credentials Command
- Purpose: This command retrieves information about all public key credential sources stored within a specific Virtual Authenticator. This is useful for inspecting the state of the Virtual Authenticator after test interactions.
- Mechanism:
- You specify the
authenticatorId
of the Virtual Authenticator you want to inspect. - The WebDriver implementation retrieves all credentials from that Virtual Authenticator.
- It encodes each credential’s details into a “Credential Parameters” JSON object (the same format as used for “Add Credential”).
- It returns an array of these “Credential Parameters” objects, representing all credentials in the Virtual Authenticator.
- You specify the
In Essence
- “Add Credential” lets you populate a Virtual Authenticator with test credentials.
- “Get Credentials” lets you inspect the contents of a Virtual Authenticator.
Why These Commands Are Important
- Control and Reproducibility: Virtual Authenticators and these commands give you precise control over test conditions, ensuring consistent and repeatable WebAuthn tests.
- Isolation: Tests are isolated from the user’s real authenticators, preventing interference with actual credentials.
- Simulation: You can simulate various authenticator types, capabilities, and user behaviors (e.g., consent, verification success/failure).
Question: How do the “Remove Credential” and “Remove All Credentials” commands differ?
The Remove Credential and Remove All Credentials commands are both WebDriver extension commands used to manage Public Key Credential Sources stored on a Virtual Authenticator within the WebAuthn WebDriver implementation. Here’s how they differ:
Remove Credential:
- Target: Deletes a specific Public Key Credential Source from the Virtual Authenticator.
- Parameters: Requires the
credentialId
of the credential to be removed. - Effect: Only the credential matching the provided
credentialId
is removed. All other credentials on the Virtual Authenticator remain untouched.
Remove All Credentials:
- Target: Deletes all Public Key Credential Sources stored on the Virtual Authenticator.
- Parameters: Does not require any specific
credentialId
as it targets all credentials. - Effect: The Virtual Authenticator’s credential database is completely cleared.
In summary:
Use “Remove Credential” when you want to delete a particular credential from a Virtual Authenticator, identified by its unique credentialId
. Use “Remove All Credentials” when you need to clear the entire credential database of a Virtual Authenticator.
Question: What is the purpose of the “Set User Verified” command?
The “Set User Verified” command in the WebAuthn WebDriver extension is designed for testing purposes. It allows developers to control the outcome of user verification for virtual authenticators. Here’s why this is important:
- Simulating User Verification: WebAuthn relies on user verification (e.g., fingerprint scan, PIN, facial recognition) to confirm the user’s presence and consent. In real-world scenarios, you can’t directly force a user to pass or fail this check. However, during automated tests, you need to precisely control the user verification result to test different authentication flows.
- Testing Different Scenarios: Imagine you are testing a website’s login system that uses WebAuthn. You’ll want to test:
- Success: Simulate a user successfully verifying themselves.
- Failure: Simulate a scenario where user verification fails.
- Improving Test Coverage: By being able to force both success and failure of user verification, you can ensure your tests cover all the possible paths within your authentication logic.
How it Works:
- Virtual Authenticators: The WebDriver extension lets you create “virtual authenticators” – software representations of real authenticators.
isUserVerified
Property: These virtual authenticators have a property calledisUserVerified
. This property determines whether user verification will succeed (if set totrue
) or fail (if set tofalse
).- “Set User Verified” Command: The “Set User Verified” command directly manipulates this
isUserVerified
property. When you call this command, you provide theauthenticatorId
of the virtual authenticator and the desiredisUserVerified
value.
In essence, the “Set User Verified” command is a testing tool that gives you fine-grained control over the simulated user verification process, enabling more comprehensive and reliable automated testing for WebAuthn implementations.
Question: How do authenticator extension capabilities enable testing of specific extension features?
Authenticator extension capabilities are crucial for robust testing of WebAuthn extensions in a WebDriver environment. Here’s how they make it possible:
1. Precise Capability Matching:
- WebDriver’s matching capabilities process ensures that the testing environment (the browser or node under test) actually supports the specific extension you want to test. For example, if your test requires the
largeBlob
extension, the capability"webauthn:extension:largeBlob": true
will only match with browsers/nodes that have implemented that extension.
2. Focused Testing:
- By declaring the needed authenticator extension capabilities, you avoid running tests that are bound to fail due to missing extension support. This allows for more targeted testing, focusing on the specific features and behaviors of the extensions you care about.
3. Virtual Authenticator Configuration:
- When you create a Virtual Authenticator using the “Add Virtual Authenticator” command, you can specify which extensions it supports. The WebDriver implementation will cross-reference this with the declared capabilities. If you try to add an extension the environment doesn’t support, you’ll get an “unsupported operation” error, preventing invalid test configurations.
Example:
Let’s say you want to test the prf
extension’s ability to generate outputs from a credential’s pseudo-random function.
- Declare Capability: Your test setup would include
"webauthn:extension:prf": true
in the desired capabilities. - Create Virtual Authenticator: You’d use the “Add Virtual Authenticator” command, specifying
"prf"
within theextensions
array of the authenticator configuration. - Run Focused Test: You can now proceed with confidence, knowing that the environment supports the
prf
extension and the virtual authenticator is properly configured to simulate its behavior. Your test can focus on interacting with theprf
extension’s client input and verifying the expected client output.
In essence, authenticator extension capabilities provide a mechanism for verifying and controlling the extension features available during WebAuthn testing. This ensures accurate, efficient, and reliable testing of specific extension functionality.
Question: What is the main purpose of the “appid” extension and how does it enable backward compatibility with FIDO U2F credentials?
The “appid” extension is primarily designed to bridge the gap between WebAuthn (specifically, the newer FIDO2 standard) and the older FIDO U2F authentication system. Its main purpose is to allow websites (Relying Parties) that previously used FIDO U2F to authenticate users with their existing U2F credentials, without requiring those users to re-register their security keys.
Here’s how it works:
The Problem:
- Different Scopes: FIDO U2F credentials are scoped to a specific AppID, a unique identifier tied to the website. WebAuthn credentials, on the other hand, are scoped to an RP ID (Relying Party ID), which is usually the website’s domain.
- Incompatibility: This difference in scoping meant that websites transitioning to WebAuthn couldn’t directly use existing U2F credentials, forcing users to register new credentials with their security keys.
The “appid” Extension Solution:
- Signaling: The Relying Party includes the “appid” extension in the
navigator.credentials.get()
call during authentication, specifying the U2F AppID it used previously. - Client Handling: The client (browser or operating system), upon encountering the “appid” extension and a U2F authenticator:
- Tries to use the provided AppID instead of the RP ID when communicating with the U2F authenticator.
- If the authenticator recognizes the AppID, it allows the user to authenticate using their U2F credential.
- Server Verification: The Relying Party server, when verifying the authentication response, needs to be aware that the
rpIdHash
in the authenticator data might correspond to the AppID instead of the RP ID.
In essence, the “appid” extension acts as a translation layer, allowing the newer WebAuthn system to understand and utilize the scope of older FIDO U2F credentials. This enables a smooth transition for websites adopting WebAuthn while retaining support for their existing user base with U2F security keys.
Question: Explain the client-side processing steps involved in using the “appid” extension.
Let’s break down the client-side processing of the “appid” extension in WebAuthn. This extension is crucial for backwards compatibility, allowing Relying Parties to transition from older FIDO U2F authentication to the more robust WebAuthn while still supporting users who registered credentials using the legacy system.
Here’s a step-by-step explanation of the client-side processing:
- Determine the Facet ID:
- The client starts by figuring out the “Facet ID” of the calling web application. This ID, defined in the FIDO AppID and Facet Specification, essentially represents the application’s unique identity in the context of WebAuthn. It’s derived from the application’s origin (protocol, domain, and port).
- Authorize the AppID:
- Next, the client needs to ensure the supplied AppID (from the extension input) is indeed authorized for the application identified by the Facet ID. This involves a comparison process:
- Ideal: The client should ideally run the full FIDO AppID authorization algorithm (FIDO AppID and Facet Specification, section 4 onwards) which involves checking the AppID’s origin against the Facet ID and, if necessary, a whitelist provided by the AppID.
- Practical: In practice, many browser implementations skip the full algorithm and use a simpler check based on same-site origins. This means they accept any AppID that shares the same site (e.g.,
example.com
) as the calling application.
- If the AppID is not authorized, a “SecurityError” DOMException is thrown, and the process stops.
- Identify U2F Credentials:
- The client scans for potential U2F authenticators available to the user.
- For each U2F authenticator, the client attempts to determine if any of the credentials listed in the
allowCredentials
option were created using the legacy FIDO U2F system. - This is done by sending a special “check-only” message to the U2F authenticator, using the SHA-256 hash of the provided AppID instead of the relying party’s RP ID.
- Handle U2F Authentication:
- If a U2F authenticator responds positively to the “check-only” message, it indicates that the credential was created using the legacy FIDO U2F system.
- The client then includes this credential in the list of
allowCredentialDescriptorList
. - When issuing the
authenticatorGetAssertion
request to the U2F authenticator, the AppID replaces the WebAuthn RP ID (since U2F authenticators work with AppIDs, not RP IDs).
- Signal AppID Usage:
- The client records whether the AppID was ultimately used to authenticate the user. This information is crucial for the Relying Party during server-side validation.
- Generate Output:
- Finally, the client extension output is set to
true
if the AppID was used,false
otherwise.
In essence, the “appid” extension instructs the client to:
- Treat certain credentials from
allowCredentials
as potentially being U2F credentials. - Issue requests to U2F authenticators using the AppID, not the RP ID.
- Inform the Relying Party whether the AppID was used, so the server can validate the response correctly.
This process ensures smooth backwards compatibility, making user authentication seamless even during a transition from legacy FIDO U2F to WebAuthn.
Question: How does the “appid” extension impact the rpIdHash verification during assertion validation by the Relying Party?
The “appid” extension is a crucial mechanism for backward compatibility with legacy FIDO U2F credentials. Here’s how it affects the rpIdHash
verification process:
Normal WebAuthn Assertion Verification:
- Calculate Expected
rpIdHash
: The Relying Party (RP) calculates the SHA-256 hash of its RP ID (options.rpId
provided during assertion generation). - Extract
rpIdHash
fromauthData
: The RP extracts therpIdHash
from theauthenticatorData
received in the assertion response. - Compare: The RP compares the calculated and extracted
rpIdHash
values. They MUST match for the assertion to be valid.
WebAuthn Assertion Verification with “appid” Extension:
- Possible AppID Hash: Because the
appid
extension allows the use of legacy FIDO U2F credentials, which are scoped to an AppID rather than an RP ID, the RP must consider an additional possibility during verification. - Calculate AppID Hash: The RP calculates the SHA-256 hash of the AppID provided in the
appid
extension input during assertion generation (options.extensions.appid
). - Compare Against Both: The RP compares the extracted
rpIdHash
fromauthData
against BOTH the calculated RP ID hash AND the calculated AppID hash. If either match, the assertion is considered valid.
Important Considerations:
- Credential Registration: The
appid
extension ONLY affects assertion verification. New credentials created with WebAuthn are always scoped to the RP ID and are NOT backward compatible with FIDO U2F. - Mixed Credentials: The RP can support both WebAuthn and legacy FIDO U2F credentials simultaneously. The
allowCredentials
list can include both WebAuthn credential IDs and FIDO U2F key handles. - Client-Side Logic: The client (browser) plays a key role in handling the
appid
extension:- Scope Check: It verifies if the caller’s origin is authorized for the specified AppID.
- Authenticator Interaction: It attempts to use the AppID hash if the RP ID hash fails during U2F authenticator interaction.
- Output Flag: The client sets the
appid
output flag totrue
if the assertion was created using the AppID hash. This signals to the RP which hash was used for verification.
In essence, the appid
extension introduces flexibility in the rpIdHash
verification by allowing a match against either the RP ID hash or the AppID hash, enabling seamless integration of legacy FIDO U2F credentials within a WebAuthn authentication flow.
Question: What is the difference between the “appid” and “appidExclude” extensions?
Both the appid
and appidExclude
extensions are designed to bridge the gap between the legacy FIDO U2F authentication system and the newer WebAuthn system during a transition period. They deal with AppIDs, which are Relying Party identifiers used in FIDO U2F, while WebAuthn uses RP IDs. Here’s a breakdown of their differences:
appid
Extension:
- Purpose: Enables authentication with existing FIDO U2F credentials during a WebAuthn authentication ceremony.
- How it works:
- Tells the client platform to treat credentials scoped to the provided AppID as if they were scoped to the current RP ID.
- Allows the client to issue U2F authentication requests to U2F authenticators using the provided AppID instead of the current RP ID.
- Informs the Relying Party server that the
rpIdHash
in the authenticator data might be the hash of the AppID instead of the RP ID.
- When to use:
- During authentication ceremonies.
- When you want users with existing FIDO U2F credentials to be able to authenticate without re-registering.
appidExclude
Extension:
- Purpose: Prevents the creation of new WebAuthn credentials on authenticators that already contain FIDO U2F credentials scoped to a specific AppID.
- How it works:
- Tells the client platform to consider both WebAuthn and FIDO U2F credentials when checking for duplicates during registration.
- Prevents registration on an authenticator that contains a FIDO U2F credential matching the provided AppID and the credential descriptors listed in the
excludeCredentials
option.
- When to use:
- During registration ceremonies.
- When you want to prevent users from accidentally creating duplicate credentials on authenticators that already have FIDO U2F credentials for the same Relying Party.
In summary:
appid
: Uses existing U2F credentials in a WebAuthn context.appidExclude
: Excludes authenticators with existing U2F credentials during WebAuthn registration.
Both extensions are useful tools for migrating from FIDO U2F to WebAuthn. appid
helps maintain a smooth user experience during authentication, while appidExclude
helps avoid confusion and potential security issues by preventing the creation of duplicate credentials.
Question: Explain the client-side processing steps involved in using the “appidExclude” extension during credential creation.
The appidExclude
extension is used during credential registration (navigator.credentials.create()
) to prevent the creation of a WebAuthn credential on an authenticator that already has a credential registered via the legacy FIDO U2F API. Here’s how the client-side processing works:
1. AppID Validation
- Facet ID Determination: The client determines the Facet ID of the calling web application using the origin. Facet ID is a concept from the FIDO AppID specification that helps determine the scope of an application.
- Authorization Check: The client checks if the provided
appidExclude
value is authorized for the determined Facet ID. This step ensures that the calling application is allowed to exclude credentials associated with the provided AppID. A “SecurityError” DOMException is thrown if the authorization fails. - Relaxed Host Comparison (Implementation Detail): In practice, many implementations simplify this authorization check. Instead of strictly adhering to the FIDO AppID algorithm, they often perform a relaxed comparison of the hosts, allowing hosts on the same site to be considered authorized.
2. Legacy Credential Check (U2F Authenticators)
- U2F Authenticator Detection: This step only applies if the authenticator in question supports the U2F protocol.
- Iterate Through
excludeCredentials
: The client iterates through each credential descriptor (C
) provided in theexcludeCredentials
list. - U2F_AUTHENTICATE Message: For each descriptor, the client sends a
U2F_AUTHENTICATE
message to the authenticator with specific parameters to check for the presence of the corresponding U2F credential:control byte
: Set to0x07
(“check-only”) to avoid triggering user interaction.challenge parameter
: Filled with 32 random bytes.application parameter
: Set to the SHA-256 hash of theappIdExclude
value.key handle length
: The length (in bytes) ofC.id
.key handle
: The value ofC.id
.
- Success Response Handling: If the authenticator responds with a success message (
message:error:test-of-user-presence-required
), it indicates the presence of the excluded credential. The client marks the authenticator as inapplicable and stops its processing. This could be done by displaying a UI message to the user or even triggering a user consent request from the authenticator (by sending anotherU2F_AUTHENTICATE
message withcontrol byte
set to0x03
). The purpose is to prevent registration on this authenticator.
3. Normal Processing Resumption
After these checks, if the authenticator is not deemed inapplicable, the client resumes the standard credential creation process (authenticatorMakeCredential
operation).
Client Extension Output
- The client extension output for
appidExclude
is alwaystrue
, indicating that the extension was processed.
Important Notes:
- User Agent Responsibility: The user agent is responsible for ensuring the smooth execution of these steps, including any necessary UI interactions.
- Relying Party Considerations: The Relying Party must understand that this extension is optional for both clients and authenticators. If the extension is not supported, the
excludeCredentials
list will only prevent the registration of new WebAuthn credentials and not legacy FIDO U2F credentials.
In essence, the appidExclude
extension allows for a more robust transition from legacy FIDO U2F to WebAuthn by ensuring that users do not end up with duplicate credentials on the same authenticator, even if those credentials were registered using different APIs.
Question: How does the client determine if a credential in excludeCredentialDescriptorList was created using U2F and should be excluded based on the “appidExclude” extension?
You’re asking about a specific step within the FIDO AppID Exclusion Extension (appidExclude
) during the credential registration process. Here’s a breakdown of how the client determines if a credential should be excluded based on this extension:
Context
- Transitioning from U2F: Websites often migrate from older FIDO U2F to the newer WebAuthn standard. Users might already have U2F credentials registered.
excludeCredentials
List: During WebAuthn registration, theexcludeCredentials
list helps prevent registering a new credential on an authenticator that already has a credential for that user.- U2F/WebAuthn Incompatibility: The
excludeCredentials
list in WebAuthn is designed for WebAuthn credentials, not U2F credentials. This is whereappidExclude
comes in.
Steps for appidExclude
Processing
- Identify U2F Authenticators: The client first identifies if the candidate authenticator supports the U2F protocol.
- Iterate through
excludeCredentials
: The client then iterates through eachPublicKeyCredentialDescriptor
(C) in theexcludeCredentials
list provided by the Relying Party. - U2F Check: For each
C
, the client sends a specialU2F_AUTHENTICATE
message to the authenticator with the following structure:
- control byte:
0x07
(“check-only”). This indicates to the authenticator that the client is only checking for the presence of a credential, not requesting a signature. - challenge parameter: 32 random bytes. This is a standard part of U2F communication.
- application parameter: SHA-256 hash of the
appId
provided in theappidExclude
extension. This is how the client checks if the credential is associated with the specifiedappId
. - key handle length: The length (in bytes) of
C.id
(the credential ID from theexcludeCredentials
list). - key handle: The actual value of
C.id
.
- Authenticator Response: The authenticator responds to the
U2F_AUTHENTICATE
message. There are two possible outcomes:
- Success (
message:error:test-of-user-presence-required
): The authenticator has a matching U2F credential. The client stops processing this authenticator and marks it as unusable for this registration, preventing the creation of a duplicate credential. The client might show UI to the user indicating this, or might silently request user presence from the authenticator as a privacy measure before marking it unusable. - Failure: The authenticator does not have a matching U2F credential. The client continues processing this authenticator as usual.
Key Points
- Purpose of
appidExclude
: TheappidExclude
extension bridges the gap between U2F and WebAuthn during registration by allowing Relying Parties to exclude authenticators based on their legacy U2F credentials. - Specific U2F Message: The client uses a specific
U2F_AUTHENTICATE
message with the “check-only” control byte to simply check for the credential’s existence without triggering any user interaction or signature generation. - Privacy Implications: The client might request user presence even when a matching U2F credential is found to avoid revealing to the Relying Party which specific credential is present on the authenticator.
Question: What are the different values for the “resident key credential property” in the “credProps” extension output, and what do they signify?
The “resident key credential property” (rk) within the “credProps” extension output is a boolean value that tells you whether the newly created credential is a client-side discoverable credential (historically known as a “resident key” or “resident credential”). Here’s a breakdown:
rk: true
: This signifies that the credential is a client-side discoverable credential, meaning it can be discovered and used for authentication without the Relying Party explicitly providing its credential ID. The authenticator will store the necessary information to allow for this discovery process.rk: false
: This indicates that the credential is a server-side credential. It cannot be discovered automatically and its credential ID must be provided by the Relying Party during authentication.rk
absent: In this case, the client platform was unable to definitively determine whether the created credential is client-side discoverable or server-side. This can happen due to certain authenticators’ behavior (more details below).
Important Nuance:
The rk
property may be omitted even when the client supports the “credProps” extension. Here’s why:
- Authenticator Behavior: Some authenticators always create client-side discoverable credentials, even if the Relying Party or client platform requests a server-side credential. In such situations, the client platform cannot confidently set
rk
tofalse
, leading to its omission.
Relying Party Interpretation:
When rk
is absent, the Relying Party should assume that the created credential is most likely a server-side credential. This is because compliant client platforms will generally strive to populate rk
if they can be certain about the credential type.
Question: Why might a client platform be forced to omit the “rk” property in the “credProps” extension output?
The rk
(resident key) property in the credProps
extension output indicates whether a newly created credential is client-side discoverable (a passkey). Ideally, the client platform should always be able to determine this and accurately report it to the Relying Party.
However, there’s a specific scenario where the client platform might be forced to omit the rk
property:
When the authenticator creates a discoverable credential even if it wasn’t explicitly requested.
Here’s why:
- Authenticator Autonomy: Some authenticators, especially older models, are designed to always create discoverable credentials. They might lack the capability to create server-side (non-discoverable) credentials.
- Client Platform Limitation: The client platform acts as an intermediary. It sends the Relying Party’s preferences (like
residentKey: "discouraged"
) to the authenticator. But if the authenticator ignores this preference and makes a discoverable credential anyway, the client platform is caught in a bind. - Uncertainty and Security: The client platform cannot definitively say
rk: false
because it knows the credential might be discoverable. But settingrk: true
would be inaccurate if the Relying Party specifically wanted a server-side credential. - Omission as a Safeguard: To avoid providing misleading information, the client platform is forced to omit the
rk
property entirely. This signals to the Relying Party that there’s uncertainty about the credential’s discoverability.
Implications for Relying Parties:
- Assume Non-Discoverable: When
rk
is missing, Relying Parties should generally assume the credential is not discoverable. This is the safer assumption, as it’s more likely the authenticator would have explicitly indicated discoverability if it was intended. - Handle Missing Data: Relying Parties must be prepared to handle cases where
rk
is missing. They might need to adjust their user experience flows or fall back to other mechanisms to determine credential discoverability.
In summary, the client platform omits rk
when it cannot guarantee the credential’s discoverability due to limitations in the authenticator’s capabilities.
Question: How can Relying Parties use the information provided by the “credProps” extension to tailor their user experience?
The credProps
extension, specifically the rk
(resident key) property within its output, is a powerful tool for Relying Parties to tailor their WebAuthn user experience. It allows them to understand the nature of the credential created and adjust their flows accordingly. Here’s how:
1. Understanding rk
:
rk: true
: The created credential is a discoverable credential, also known as a passkey. This means the user can authenticate without providing a username, as the authenticator can identify the relevant credential based on the RP ID alone.rk: false
: The created credential is a server-side credential. The user needs to be identified first (e.g., by providing a username), and the Relying Party must provide the credential ID to the authenticator.rk
absent: The client couldn’t determine whether the credential is discoverable or server-side. This usually happens with older authenticators that don’t explicitly signal this property.
2. Tailoring User Experience:
- Promoting Passwordless Flows: If
rk: true
, the Relying Party can confidently offer passwordless authentication options in the future. They can prioritize this flow for users with passkeys, leading to a more streamlined and convenient experience. - Simplifying Registration: During registration, if
rk: true
is expected, the Relying Party can guide the user towards authenticators that support discoverable credentials (e.g., platform authenticators, security keys with FIDO2 support). Ifrk: false
, they might prioritize instructions for authenticators that are primarily server-side (e.g., older security keys). - Account Recovery: When a user sets up account recovery, Relying Parties can suggest a different type of credential based on the
rk
value of the initial credential. For instance, if the user primarily uses discoverable credentials, suggesting a server-side credential as a recovery option might be beneficial. - Managing Multiple Credentials: The
rk
property helps manage multiple credentials for a single user account. Knowing which credentials are discoverable enables the Relying Party to handle potential conflicts or duplicates efficiently. - Conditional UI: Relying Parties can customize their UI based on the credential type. If
rk: true
, they might hide username fields in login forms, assuming passwordless authentication. Ifrk: false
or absent, the username field would be necessary.
3. Handling Missing rk
:
When the rk
property is absent, Relying Parties need to employ careful strategies:
- Conservative Approach: Assume the credential is server-side and proceed with flows that require username input and credential ID management. This ensures compatibility with older authenticators but might miss opportunities for streamlined experiences.
- Progressive Enhancement: Use feature detection to check for
credProps
support. If available, use therk
value to tailor the experience. If not, fall back to the conservative approach. - User Hints: Rely on user hints (e.g., “security key”, “client device”) provided in
PublicKeyCredentialCreationOptions
and adjust the UI accordingly, even ifrk
is absent.
In essence, the credProps
extension, through its rk
property, empowers Relying Parties to provide more intelligent and user-centric WebAuthn flows. By understanding the type of credential created, they can optimize registration, authentication, and account recovery processes for a smoother and more secure user journey.
Question: What steps must a Relying Party take when the promise returned by navigator.credentials.create() is rejected?
The WebAuthn specification dictates that if the promise returned by navigator.credentials.create()
is rejected, the Relying Party (RP) must abort the registration ceremony. It should also provide appropriate feedback to the user, guiding them through the issue.
Here’s a breakdown of the steps and considerations:
- Abort the Ceremony: The registration ceremony should be immediately stopped to prevent any further processing with the potentially incomplete or erroneous data.
- Handle the Rejection: The
.catch()
block of the promise should capture the rejection and its associated error. The error object will provide information about the reason for the rejection. - User Feedback: The RP should display a user-friendly error message explaining the problem. The specific message should be tailored to the type of error returned.
- General Error: A generic error message like “Registration failed. Please try again.” can be displayed if the specific reason is unclear.
- Specific Errors: If the error object provides more detailed information, the error message should reflect it. For example:
"NotAllowedError"
: Might indicate the user declined consent or there’s a permissions issue. The message could be: “You need to authorize registration on your authenticator.”"InvalidStateError"
: This typically signifies theexcludeCredentials
list identified a credential bound to the authenticator. Instruct the user to try a different authenticator: “Please use a different authenticator for registration.”"ConstraintError"
: This suggests the chosen authenticator doesn’t meet the RP’s requirements (like lacking resident key support or user verification capability). The message could be: “The chosen authenticator doesn’t meet our security requirements. Please use a different one.”
- Guidance for User Action: The error message should also guide the user on how to resolve the issue. For instance:
- Suggest checking their authenticator and trying again.
- Advise using a different authenticator if the current one is incompatible or has issues.
- Provide contact information for support if the user encounters persistent problems.
Example Code:
navigator.credentials.create({ publicKey: options })
.then(function (newCredentialInfo) {
// Send new credential info to server...
})
.catch(function (err) {
console.error("Registration failed:", err);
if (err.name === "NotAllowedError") {
alert("You need to authorize registration on your authenticator.");
} else if (err.name === "InvalidStateError") {
alert("Please use a different authenticator for registration.")
} else if (err.name === "ConstraintError") {
alert("The chosen authenticator doesn't meet security requirements. Please use a different one.")
} else {
alert("Registration failed. Please try again.");
}
});
Important Note: The exact handling of the rejection and the specific error messages should be determined based on the RP’s security policies, risk tolerance, and the overall user experience they aim to provide.
Question: Explain the process of verifying the client data during credential registration.
Let’s break down how client data is verified during WebAuthn credential registration. Think of this as a safety check to ensure the registration request is legitimate and hasn’t been tampered with.
What is Client Data?
Client data is a JSON object that captures important contextual information about the registration attempt. It’s created by the user’s browser (the WebAuthn Client) and includes:
- type: Always set to “webauthn.create” for registration.
- challenge: A randomly generated value provided by the Relying Party (your website) that acts like a unique puzzle for this registration.
- origin: The exact web address (including protocol and port) of the page requesting registration.
- crossOrigin: A boolean flag indicating if the request originated from an iframe with a different origin than the main page.
- topOrigin: If
crossOrigin
is true, this field contains the origin of the top-level page.
Verification Steps
The Relying Party server receives this client data (alongside other information) and performs these checks:
- JSON Parsing and Structure:
- The server parses the
clientDataJSON
(which is a byte array) into a usable JSON object. - It checks if the object has the expected fields (
type
,challenge
,origin
,crossOrigin
,topOrigin
). Any missing fields or unexpected data structures are a red flag.
- Type Validation:
- The
type
field MUST be “webauthn.create”. This ensures the client data is indeed for registration, not for a different WebAuthn operation (like authentication).
- Challenge Matching:
- The
challenge
value within the client data MUST exactly match the challenge the Relying Party server originally generated. This proves that the client data is associated with this specific registration attempt.
- Origin Verification:
- The
origin
MUST match the expected web address from which the registration request was supposed to come. This prevents malicious websites from attempting to register credentials on behalf of legitimate Relying Parties. The strictness of the origin check depends on the Relying Party’s security policies (e.g., exact match, allowing subdomains). - If
topOrigin
is present, it’s also verified against expected values based on the Relying Party’s policies for embedded content.
Why is this important?
- Prevents Replay Attacks: The challenge ensures the client data can’t be reused for a different registration attempt.
- Verifies Request Source: Origin verification confirms the registration is happening on the legitimate website.
- Protects Against Tampering: Matching the challenge and validating the structure means the client data hasn’t been altered in transit.
In essence, client data verification acts as a crucial safeguard against various attacks, making the WebAuthn registration process more secure.
Question: How does the Relying Party handle situations where the topOrigin member is present in the client data during registration?
OK, here’s a breakdown of how a Relying Party (RP) handles the topOrigin
member during WebAuthn registration, along with security implications:
What is topOrigin
?
topOrigin
appears in theclientDataJSON
ONLY when a WebAuthn registration is initiated from within an iframe that’s cross-origin to the main website.- It reflects the origin of the top-most browsing context – the website the user sees in their address bar.
- If the registration happens directly on the website (not in a cross-origin iframe),
topOrigin
will be absent.
Relying Party Actions
- Expectation Check: The RP MUST first determine if it expects to be embedded in a cross-origin iframe.
- If the RP is designed for standalone use, the mere presence of
topOrigin
is suspicious and likely an attack attempt. The ceremony should be aborted. - If embedding is allowed, proceed to the next steps.
- Origin Verification: The RP MUST verify that the value of
topOrigin
matches a legitimate embedding context.
- Whitelisting: If the RP knows the specific sites that may embed it, the
topOrigin
should be compared against a whitelist of allowed origins. Any mismatch means a potentially malicious actor is involved. - Dynamic Validation: For more flexible embedding, the RP may use a dynamic procedure (e.g., consulting a database or policy engine) to determine if the
topOrigin
is acceptable at that moment. - Subdomain Considerations: Be very cautious about broadly allowing subdomains of the RP’s domain as
topOrigin
(e.g., if your RP ID isexample.com
, be wary of accepting anything from*.example.com
). This is because a malicious subdomain could host code to intercept WebAuthn operations.
- Risk Assessment: Even with a matching
topOrigin
, consider this a potential risk factor in your overall security evaluation. Embedding, by its nature, introduces complexities that could be exploited.
Security Implications
- UI Redressing/Clickjacking: The primary risk is that an attacker could create a website that embeds your RP in an iframe, hiding or manipulating the RP’s UI while tricking the user into registering a credential that the attacker can then control.
- Code Injection: Malicious code on the embedding site (
topOrigin
) might be able to tamper with the registration process, possibly substituting a different public key or manipulating other parameters.
Example:
Let’s say your RP is hosted at https://login.example.com
, and you allow embedding only from https://partner.com
.
- Valid Case: A user visits
https://partner.com
, which has an iframe loading your login page. During registration, theclientDataJSON
will include:origin
:https://login.example.com
topOrigin
:https://partner.com
- This is expected and should pass verification.
- Malicious Case: An attacker creates
https://evil.com
, embedding your login page. TheclientDataJSON
might have:origin
:https://login.example.com
topOrigin
:https://evil.com
- This should be rejected because the
topOrigin
doesn’t match your whitelist.
Key Points:
topOrigin
presence signals embedding.- Verification is crucial to prevent attacks.
- Embedding always increases complexity and potential risk.
Let me know if you have any further questions!
Question: How does the Relying Party assess the trustworthiness of an attestation statement?
The Relying Party assesses the trustworthiness of an attestation statement in a multi-step process, combining cryptographic verification with policy decisions and trust anchor lookups. Here’s a breakdown:
1. Cryptographic Verification
- Decoding and Format Matching: The attestation object received from the authenticator is decoded using CBOR. The Relying Party then determines the attestation statement format (e.g., “packed”, “tpm”, “android-key”) by matching the
fmt
value against registered formats. - Verification Procedure: Each attestation statement format defines its own verification procedure. This procedure involves:
- Verifying the attestation signature using the provided public key.
- Checking the validity of the attestation certificate (if present).
- Verifying that data within the attestation statement (like the AAGUID) matches the authenticator data.
2. Trust Anchor Lookup
- Acceptable Trust Anchors: Based on the successfully verified attestation type and format, the Relying Party consults a trusted source to obtain a list of acceptable trust anchors. These trust anchors are typically root certificates or similar entities that the Relying Party trusts.
- Trust Path Validation: The Relying Party uses the attestation trust path (usually a chain of X.509 certificates provided in the attestation statement) to check if the attestation public key chains up to one of the acceptable trust anchors. This confirms that the attestation originates from a trusted source.
3. Policy Decisions
- Attestation Type Acceptance: The Relying Party’s policy dictates whether certain attestation types (e.g., Basic, Self, AttCA) are acceptable. For instance, a Relying Party might reject self attestation as it doesn’t provide strong assurances about the authenticator’s origin.
- Authenticator Model Evaluation: If the attestation provides information about the authenticator model, the Relying Party might have policies based on the model’s known security characteristics. They may accept or reject the attestation based on the model.
- Risk Assessment: Relying Parties can incorporate attestation information into their risk engines. Factors like the authenticator’s security level, certification status, and other details can influence the overall risk score associated with a registration or authentication.
Important Considerations:
- Trust Anchor Source: The source of acceptable trust anchors must be trustworthy. The FIDO Metadata Service is an example of such a source, providing information about certified authenticators and their corresponding root certificates.
- Certificate Revocation: Relying Parties must have mechanisms to check for revoked attestation certificates. This can be done by consulting Certificate Revocation Lists (CRLs) or using the Online Certificate Status Protocol (OCSP).
- Policy Enforcement: The Relying Party’s security policies should clearly define which attestation types, formats, and authenticator properties are acceptable.
In essence, the Relying Party’s assessment of an attestation statement relies on a combination of cryptographic proof, trust in the attestation source, and adherence to its internal security policies.
Question: What actions should a Relying Party take if the attestation statement is found to be untrustworthy?
The WebAuthn spec outlines the following recommendations for Relying Parties if an attestation statement is deemed untrustworthy during a registration ceremony:
Primary Recommendation: Fail the Registration Ceremony
The spec strongly advises that the Relying Party SHOULD fail the registration if the attestation statement, although successfully verified, doesn’t meet the trust requirements. This is the most secure course of action, as it prevents potentially compromised or uncertified authenticators from being used.
Alternative: Register with “Self Attestation” Trust Level
As an alternative, the spec acknowledges that Relying Parties MAY choose to register the credential ID and public key even with an untrustworthy attestation. However, they should treat this credential as if it were self-attested.
Implications of Treating a Credential as Self-Attested:
- Reduced Trust: By accepting an untrustworthy attestation, the Relying Party essentially acknowledges there’s no cryptographic proof the credential was generated by a specific authenticator model.
- Potential Risks: This opens the door to vulnerabilities, as the authenticator’s claimed security properties haven’t been independently validated.
Factors to Consider Before Accepting Untrustworthy Attestations:
- Relying Party Policy: The decision hinges on the Relying Party’s internal policies and risk tolerance. Some organizations may have strict security mandates, while others might prioritize user convenience over absolute assurance.
- Authenticator Characteristics: Understanding the nature of the authenticator is crucial. If it’s a platform authenticator tied to a user’s device, the risks might be lower than with an unknown roaming authenticator.
- Context of Use: The sensitivity of the protected data and the consequences of a potential breach should factor into the decision.
Key Takeaways:
- Failing registration with an untrustworthy attestation is the safest approach.
- Accepting untrustworthy attestations (treating them as self-attested) introduces security risks and should only be done after careful policy consideration.
Remember: Attestation is a valuable tool for assessing authenticator security, but it’s just one piece of the puzzle. Relying Parties should employ comprehensive security practices, including robust risk analysis and user education.
Question: Why is it recommended that Relying Parties reject duplicate credential IDs during registration?
The WebAuthn specification recommends Relying Parties reject duplicate credential IDs during registration for a critical security reason: the potential for credential ID reuse attacks.
Here’s a breakdown of the problem:
- Credential IDs are probabilistically unique. This means they are generated with enough entropy to make accidental duplication highly unlikely.
- Attestation doesn’t always prove private key possession. Attestation types, except for Self Attestation, do not include a self-signature by the credential private key. This means the Relying Party can’t be absolutely sure the authenticator presenting the attestation actually controls the private key associated with the credential ID.
The Attack Scenario:
- Compromised Credentials: An attacker manages to obtain a user’s credential ID and public key for a target website. This could happen through phishing, malware, or a data breach.
- Registration Attempt: The attacker then attempts to register the victim’s credential as their own on the target website. They present the stolen credential ID and public key during registration.
- Relying Party Accepts: If the Relying Party accepts this registration and replaces the victim’s existing registration, the attacker now controls the victim’s account.
Impact:
- Account Takeover: If the credentials are discoverable (e.g., resident keys/passkeys), the victim will be automatically logged into the attacker’s account the next time they try to access the website.
- Data Theft: The attacker gains access to the victim’s data stored on the website.
Mitigation:
By rejecting duplicate credential IDs, the Relying Party effectively prevents this attack. If the attacker tries to register the stolen credential ID, the registration will be denied because the ID is already associated with the victim’s account.
Additional Notes:
- While Self Attestation does include a self-signature, rejecting duplicate IDs is still good practice for consistency and to handle situations where a Relying Party might accept multiple attestation types.
- Even with duplicate ID rejection, it’s important for Relying Parties to encourage users to register multiple credentials per account. This provides redundancy and helps with account recovery.
In conclusion, rejecting duplicate credential IDs is a simple but crucial security measure that helps protect users from credential ID reuse attacks.
Question: What information should be stored in a credential record by the Relying Party?
The WebAuthn spec recommends storing the following information in a credential record:
REQUIRED:
type
: The type of the public key credential source. This will always be “public-key” for WebAuthn.id
: The credential ID of the public key credential source. This is a unique identifier generated by the authenticator for this credential.publicKey
: The credential public key of the public key credential source. The Relying Party will use this to verify assertions from the authenticator.signCount
: The latest value of the signature counter from the authenticator data. This counter is incremented each time the credential is used, and helps detect cloned authenticators.transports
: The transports that the authenticator is believed to support, as provided bygetTransports()
during registration. This helps the client understand how to reach the authenticator for future authentication ceremonies.uvInitialized
: A Boolean value indicating if a credential from this public key credential source has ever been used with user verification. This can help the Relying Party make decisions about user verification requirements in the future.backupEligible
: The value of the backup eligibility (BE) flag from the authenticator data. This indicates whether the credential can be backed up to another device.backupState
: The latest value of the backup state (BS) flag from the authenticator data. This indicates whether the credential is currently backed up.
OPTIONAL:
attestationObject
: The attestation object returned by the authenticator during registration. This provides evidence about the authenticator’s origin and security properties, but is not strictly necessary for WebAuthn to function.attestationClientDataJSON
: The client data JSON from the registration ceremony. This can be used in conjunction withattestationObject
to re-verify the attestation signature at a later date.
WebAuthn Extensions
Extensions may define additional fields that need to be stored in the credential record. For example, the devicePubKey
extension requires storing a set of device-bound key records.
Relying Party Discretion
Relying Parties may store any other information that they deem necessary for their implementation. They may also choose to omit any optional fields that they do not need.
Importance of Secure Storage
Credential records contain sensitive information that should be protected from unauthorized access. Relying Parties should implement appropriate security measures, such as encryption and access control, to ensure the confidentiality and integrity of credential records.
Question: What are the optional contents that a credential record may include?
According to the WebAuthn Level 3 spec, a credential record MAY include these optional contents:
- attestationObject: This is the full
attestationObject
returned by the authenticator during registration. Storing this allows the Relying Party to reference the credential’s attestation statement at a later time. This can be useful for auditing purposes or for re-evaluating the trustworthiness of a credential in the future. - attestationClientDataJSON: This is the
clientDataJSON
from the registration response. Storing it alongsideattestationObject
allows the Relying Party to re-verify the attestation signature at any point in the future. This can be valuable if concerns about attestation validity arise later on.
Note: WebAuthn extensions may define additional optional fields for the credential record based on their specific needs.
Relying Parties have the flexibility to include these optional contents based on their specific security and auditing requirements. They are not strictly required for basic WebAuthn functionality but offer valuable capabilities for more advanced use cases.
Question: How does the Relying Party handle the userHandle attribute during assertion verification?
The userHandle
attribute’s role in assertion verification depends on whether the Relying Party provided a list of allowed credentials (allowCredentials
) during the authentication ceremony:
Scenario 1: allowCredentials
is NOT empty:
- The Relying Party has already identified the user (e.g., through a username or cookie).
- The
userHandle
returned in theAuthenticatorAssertionResponse
is optional. - If present, the Relying Party MUST verify that the returned
userHandle
matches the user handle associated with the identified user account. This acts as an additional check to ensure the correct user is authenticated. - If absent, the Relying Party does not need to perform any specific action related to the
userHandle
.
Scenario 2: allowCredentials
is empty:
- The Relying Party has not identified the user beforehand.
- The
userHandle
returned in theAuthenticatorAssertionResponse
is mandatory. - The Relying Party MUST verify that the user account identified by the returned
userHandle
contains a credential record matching thecredential.rawId
. This allows the Relying Party to identify the user based on theuserHandle
associated with the used discoverable credential.
In Summary:
userHandle
is used for user identification whenallowCredentials
is empty (discoverable credentials).- It acts as an additional verification step when
allowCredentials
is not empty. - Relying Parties should handle both cases (
userHandle
present or absent) appropriately in their authentication logic.
Question: What steps are involved in verifying the authenticator data during assertion verification?
You’re asking about a crucial part of WebAuthn’s security: validating data sent by the authenticator to ensure the user is genuinely who they claim to be. Here’s a breakdown of the steps involved, referencing the relevant sections of the WebAuthn spec you provided:
Steps for Verifying Authenticator Data in an Assertion
These steps occur within the broader “Verifying an Authentication Assertion” process outlined in section 7.2 of the WebAuthn spec.
- Obtain the
authenticatorData
:
- The
authenticatorData
is a byte array included in theAuthenticatorAssertionResponse
object sent by the authenticator. (See §5.2.2)
- Compute the hash of the Client Data:
- You’ve already validated and parsed the
clientDataJSON
(steps 6-11 in §7.2). - Compute the SHA-256 hash of the raw
clientDataJSON
bytes and store the result ashash
. (Step 18 in §7.2)
- Validate the Relying Party ID (RP ID) Hash:
- Extract the
rpIdHash
from the beginning ofauthenticatorData
. (See §6.1, Table 1) - Compute the SHA-256 hash of the Relying Party’s expected RP ID.
- Verify that these two hashes match. This confirms that the response is intended for the correct website. (Step 13 in §7.2)
- Special Case: AppID Extension: If the
appid
extension (§10.1.1) is used, you might need to compare therpIdHash
against the hash of the AppID instead of the RP ID.
- Check the User Present (UP) Flag:
- Extract the
flags
byte fromauthenticatorData
. (See §6.1, Table 1) - Verify that the User Present (UP) bit is set (bit 0). This confirms the user physically interacted with the authenticator. (Step 14 in §7.2)
- Conditional User Verification (UV) Flag Check:
- Determine if your policy requires user verification for this assertion (based on
options.userVerification
). - If user verification is required, verify that the User Verified (UV) bit in the
flags
is set (bit 2). (Step 15 in §7.2) - If user verification is not required, you can ignore the UV flag.
- Check Backup Eligibility (BE) and Backup State (BS) Flags:
- Extract the BE and BS bits from the
flags
byte (bits 3 and 4 respectively). - If the BE bit is not set (single-device credential), verify that the BS bit is also not set. (Step 16 in §7.2)
- If you use backup state in your logic:
- Retrieve the stored
backupEligible
andbackupState
values from the user’scredentialRecord
. - Compare the current BE and BS flags with the stored values.
- Enforce your policy based on these values (e.g., require additional authentication factors if the backup state has changed unexpectedly). (Step 17 in §7.2)
- Retrieve the stored
- Extract the BE and BS bits from the
- Validate Extension Outputs (If applicable):
- If you used any authenticator extensions, verify their output values as defined by each extension’s specification. (Step 18 in §7.2)
- Verify the Signature:
- Use the credential public key stored in the user’s
credentialRecord
to verify that thesignature
provided in the response is a valid signature over the concatenation ofauthData
andhash
. (Step 20 in §7.2) - This signature confirms that the response came from the authenticator holding the corresponding private key.
- Signature Counter Verification (If applicable):
- If either the
authData.signCount
or the storedcredentialRecord.signCount
is non-zero:- If
authData.signCount
is greater thancredentialRecord.signCount
, the signature counter is valid. - If
authData.signCount
is less than or equal tocredentialRecord.signCount
, it indicates a potential cloned authenticator. Consider your risk policies and actions (e.g., rejecting the authentication or requiring additional verification). (Step 21 in §7.2)
- If
Key Points:
- These checks protect against various attacks, including replay attacks, man-in-the-middle attacks, and attempts to use cloned authenticators.
- The specific actions you take if verification fails (e.g., rejecting the authentication, requiring additional factors) depend on your security policy and risk tolerance.
Let me know if you’d like to explore any of these steps in more detail or have further questions about WebAuthn security.
Question: Explain the process of verifying the assertion signature.
The process of verifying an assertion signature is a crucial part of the WebAuthn authentication ceremony. It ensures that the user possesses the private key corresponding to the registered public key and that the authentication response hasn’t been tampered with. Here’s a breakdown of the process, as described in section 7.2 “Verifying an Authentication Assertion” of the WebAuthn Level 3 specification:
1. Data Retrieval and Preparation
- Obtain necessary data: The Relying Party server receives the following from the client:
credential.response
: AnAuthenticatorAssertionResponse
object containing the authenticator’s response.credential.id
: The credential ID of the public key credential used.clientExtensionResults
: The results of any client extensions.
- Identify the user: The Relying Party matches
credential.id
with a storedcredentialRecord
associated with the user. This is done either based on a pre-identified user (e.g., through a username) or by usingresponse.userHandle
if the user wasn’t identified beforehand. - Extract data from the response: Retrieve the following from the
AuthenticatorAssertionResponse
:cData
: TheclientDataJSON
byte array.authData
: TheauthenticatorData
byte array.sig
: Thesignature
byte array.
2. Client Data Validation
- Decode and parse
clientDataJSON
: DecodecData
using UTF-8 and parse it as a JSON object (C
). - Verify client data fields:
C.type
MUST be “webauthn.get”.C.challenge
MUST match the base64url encoding of the challenge sent by the Relying Party.C.origin
MUST be an expected origin. IfC.topOrigin
is present, it also needs to be validated.
3. Authenticator Data Validation
- Verify RP ID hash: Ensure the
rpIdHash
inauthData
matches the SHA-256 hash of the RP ID. - Check user presence (
UP
flag): TheUP
flag inauthData
MUST be set, indicating user presence. - Verify user verification (
UV
flag): If the Relying Party requires user verification, ensure theUV
flag inauthData
is set. - Validate backup eligibility and state: Check the
BE
andBS
flags against thecredentialRecord
. - Check client and authenticator extension outputs: Validate that the extension outputs match the expected values based on the inputs and Relying Party policy.
4. Signature Verification
- Hash client data: Compute the SHA-256 hash of
cData
and store it inhash
. - Concatenate data: Create a byte string by concatenating
authData
andhash
. - Verify signature: Use the credential public key from
credentialRecord.publicKey
and the signature algorithm indicated by the credential to verify thatsig
is a valid signature over the concatenated data.
5. Post-Verification Steps
- Update signature counter: Update
credentialRecord.signCount
with the value fromauthData.signCount
, if it’s greater. If it’s not, handle potential cloning. - Process attestation (optional): If
response.attestationObject
is present, the Relying Party can optionally verify the attestation statement within it. - Update credential record: Update the
credentialRecord
with the newsignCount
,backupState
, and potentiallyuvInitialized
.
Security Implications
- Critical for security: Signature verification ensures the authenticity and integrity of the authentication response, preventing unauthorized access.
- Challenge uniqueness: Using a unique challenge for each authentication prevents replay attacks.
- Relying Party responsibility: It’s crucial for the Relying Party to implement all the verification steps correctly to maintain the security of the system.
Let me know if you want a deeper dive into a particular aspect of the process, such as handling potential cloning scenarios or validating attestation statements.
Question: What actions should a Relying Party take if a signature counter mismatch is detected during assertion verification?
The WebAuthn specification is intentionally vague about the specific actions a Relying Party (RP) should take when encountering a signature counter mismatch. This is because the appropriate response is highly dependent on the RP’s specific threat model, risk tolerance, and business context.
Here’s a breakdown of the situation and considerations for possible RP responses:
What a signature counter mismatch means:
A signature counter mismatch occurs when the signCount
value received in an assertion is less than or equal to the signCount
value previously stored for that credential. This signals a potential problem, as the counter is supposed to monotonically increase with each successful authentication. The mismatch could indicate:
- Cloned Authenticator: An attacker might have created a copy of the authenticator and is using it to generate assertions.
- Authenticator Malfunction: The authenticator might have experienced a software or hardware error causing the counter to reset or decrement.
- RP Error: The RP might have incorrectly stored or retrieved the previous
signCount
value.
Possible RP Actions:
- Do Nothing: This is generally NOT recommended, especially if the RP has reason to believe the account might be targeted. Ignoring the mismatch could leave the account vulnerable to attacks from the potentially cloned authenticator.
- Log the Event: At a minimum, the RP should log the mismatch event, recording relevant information like the credential ID, the user account, the observed
signCount
values, timestamps, and any other available contextual information. This data can be invaluable for later analysis if suspicious activity occurs. - Increase Monitoring: The RP could increase monitoring of the user account for suspicious activities, such as login attempts from unusual locations or changes to sensitive account information.
- Require Additional Authentication: The RP could challenge the user with additional authentication factors, such as a one-time password sent to a registered email address or phone number. This helps to confirm the user’s identity and legitimacy of the authentication attempt.
- Trigger Account Recovery: In high-risk situations or if the RP suspects malicious activity, initiating an account recovery process is appropriate. This might involve disabling the affected credential, requiring the user to verify their identity through an out-of-band channel, and potentially resetting security credentials.
- Investigate: Depending on the RP’s resources and capabilities, further investigation into the mismatch may be warranted. This could include contacting the user, analyzing logs for other anomalies, or even collaborating with authenticator manufacturers to analyze the potential for a cloned device.
Choosing the right action:
The appropriate action for the RP depends on a careful assessment of the situation. Some factors to consider include:
- Sensitivity of the account: Accounts with access to sensitive personal or financial information require stronger security measures.
- Likelihood of a targeted attack: If the RP has reason to believe the account is targeted, taking more aggressive actions is warranted.
- Authenticator’s attestation: The attestation statement provided during credential registration can offer insights into the authenticator’s security posture and potential vulnerability to cloning.
- User behavior: Analyzing the user’s typical login patterns and any recent changes in behavior can help to assess the legitimacy of the authentication attempt.
- RP’s risk tolerance: Some RPs are more risk-averse than others and might opt for more conservative measures, even for minor discrepancies.
In Conclusion:
A signature counter mismatch is a serious security signal that RPs should not ignore. The appropriate response varies depending on the specific circumstances and a well-defined policy for handling such events is crucial. Implement a graduated response system, starting with logging and monitoring, and escalating to stronger measures like additional authentication or account recovery based on the assessed risk.
Question: What are the implications of the “devicePubKey” extension for Relying Party risk analysis systems?
The devicePubKey
extension in WebAuthn offers Relying Parties a valuable “device continuity” signal, enhancing their risk analysis systems for multi-device credentials (aka passkeys). Here’s how it works and its implications:
How it Works:
- Key Generation: When a user registers a passkey with the
devicePubKey
extension enabled, the authenticator generates a unique, hardware-bound device key pair tied to that specific user credential. This key pair remains on the initial authenticator. - Signature: Subsequent authentication attempts on the same device, using the same passkey and the
devicePubKey
extension, produce a signature using the corresponding device private key. This signature, along with the device public key, is sent to the Relying Party. - Backup & New Device: If the passkey is backed up and used on a new device, that device generates its own device key pair tied to the user credential, and a new signature is produced using that new device private key.
Implications for Risk Analysis:
- Device Recognition: By tracking the device public keys and verifying the accompanying signatures, Relying Parties can identify specific devices previously used with a particular passkey. This allows the system to recognize familiar devices and associate them with lower risk.
- Suspicious Activity Detection: Conversely, if a login request comes from an unrecognized device (new device public key), coupled with other suspicious signals (unusual geolocation, time of day, etc.), the risk score increases. This enables the Relying Party to challenge the authentication more rigorously, perhaps requiring additional verification steps.
- Enhanced Security for Passkeys: Passkeys, being multi-device credentials, inherently face a slightly higher risk of account takeover if a single device is compromised. The
devicePubKey
extension mitigates this risk by providing a device-specific continuity signal, making it harder for an attacker to impersonate a legitimate user on a new device. - Attestation & Trust Levels: The
devicePubKey
extension also supports attestation, allowing Relying Parties to assess the trustworthiness of the device hardware protecting the device private key. This further informs the risk analysis process.
In Summary:
The devicePubKey
extension strengthens the security of passkeys by providing a device-level layer of authentication, enabling Relying Parties to:
- Distinguish between known and unknown devices.
- Flag potentially fraudulent activity from unfamiliar devices.
- Implement more nuanced risk-based authentication policies for passkey-based logins.
However, Relying Parties should carefully consider the privacy implications of tracking user devices and use this data responsibly.
Question: How does the Relying Party verify the device-bound key signature before storing the extension output?
You’re asking about the devicePubKey extension, which is designed to help Relying Parties track device continuity for multi-device credentials (like passkeys). This helps mitigate risks when a user logs in from a new or unusual device. Here’s a breakdown of how a Relying Party verifies the device-bound key signature:
Steps for Verification
- Requesting the Extension: The Relying Party includes the
devicePubKey
extension in thePublicKeyCredentialRequestOptions
when callingnavigator.credentials.get()
. - Receiving the Response: The authenticator returns an
AuthenticatorAssertionResponse
which includes:- The standard assertion signature (signed by the credential private key)
- Authenticator data containing the devicePubKey extension output
- An unsigned extension output containing a signature (
dpkSig
) generated with the device private key
- Parsing the Extension Output: The Relying Party parses the
devicePubKey
extension output from the authenticator data. It extracts:aaguid
: The AAGUID of the authenticator managing the device-bound keydpk
: The device public keyscope
: Indicates device-wide or per-app scopenonce
: Random value generated by the authenticator (can be empty)fmt
: Attestation statement format for the device-bound keyattStmt
: Attestation statement for the device-bound keysignature
: ThedpkSig
value from the unsigned extension output
- Signature Verification: The Relying Party MUST verify the
dpkSig
signature:- The signed data is the same data that was signed for the standard assertion: the concatenation of
authData
and the hash of the client data. - The signature verification uses the extracted
dpk
(device public key) and the signature algorithm of the user credential.
- The signed data is the same data that was signed for the standard assertion: the concatenation of
- Optional Attestation Verification: If attestation was requested, the Relying Party can optionally verify the
attStmt
following the standard attestation verification procedure for the givenfmt
. TheattStmt
signature covers the AAGUID, device public key, and nonce (if present). See Section 10.2.2.2.2 of the WebAuthn spec for details on attestation calculation.
After Verification
- If the
dpkSig
verification fails: The Relying Party should treat this as a potential security issue and may choose to reject the authentication attempt. - If the
dpkSig
verification succeeds: The Relying Party can proceed with its risk assessment. A successful verification provides a strong signal that the current authentication attempt is coming from the same device that was used in previous authentications with this credential.
Important Considerations:
- Relying Party Policy: The Relying Party defines its own policies regarding:
- Whether a devicePubKey response is required.
- Which attestation types are acceptable for device-bound keys.
- How to incorporate device continuity signals into risk analysis.
- Storage: Upon successful verification, the Relying Party typically stores the device-bound key information (
aaguid
,dpk
,scope
,fmt
,attStmt
) associated with the user credential. This allows for comparison in future authentication attempts.
In Essence:
The devicePubKey extension offers a powerful mechanism for tracking device continuity, adding an extra layer of security to authentication with multi-device credentials. The signature verification process is crucial for ensuring the authenticity and integrity of this signal.
Question: What security considerations should Relying Parties take into account regarding cryptographic challenges?
The WebAuthn specification outlines crucial security considerations for Relying Parties concerning cryptographic challenges. These challenges play a vital role in thwarting replay attacks, a common threat in authentication systems. Here’s a breakdown of the key points:
Challenge Generation:
- Server-Side Generation: Challenges MUST be generated by the Relying Party server in a trusted environment, NOT on the client-side. This prevents attackers from manipulating or predicting the challenge.
- Randomness: Challenges MUST be truly random and contain enough entropy to make guessing them impractical. At least 16 bytes of random data is RECOMMENDED.
- Storage: The Relying Party SHOULD store the generated challenge temporarily until the authentication ceremony is complete. This is essential for verifying that the challenge received back from the authenticator matches the one originally sent.
Challenge Verification:
- Challenge Comparison: The Relying Party MUST verify that the
challenge
value returned in the authenticator’s response matches the challenge originally sent. This ensures that the response is not a replay of a previous authentication attempt.
Rationale and Importance:
- Replay Attacks: A replay attack occurs when an attacker captures a valid authentication response and attempts to reuse it to gain unauthorized access. Random, unpredictable challenges prevent this because each authentication attempt uses a unique challenge.
- Client-Side Compromise: If challenges were generated on the client-side, an attacker who has compromised the user’s browser or device could easily manipulate the challenge, rendering WebAuthn authentication ineffective.
Insecure Practices to Avoid:
- Predictable Challenges: Using predictable challenges, such as timestamps or sequential numbers, makes it easier for attackers to guess or forge them.
- Reusing Challenges: Reusing the same challenge across multiple authentication ceremonies creates a vulnerability to replay attacks.
Additional Considerations:
- Challenge Length: The length of the challenge impacts its security. While 16 bytes is generally considered secure, some Relying Parties may opt for longer challenges for extra protection.
- Challenge Storage: The mechanism for storing challenges on the server-side should be secure and prevent unauthorized access or modification.
By adhering to these security considerations, Relying Parties can significantly enhance the security of their WebAuthn implementations and protect against common attacks.
Question: Describe the limitations of attestation statements in providing complete security guarantees.
You’re right to be cautious! Attestation statements, while useful, don’t offer complete security guarantees. Here’s a breakdown of their limitations:
1. Man-in-the-Middle (MitM) Attacks During Registration:
- The Vulnerability: The biggest weakness lies in the initial registration ceremony. A MitM attacker could intercept the communication between the user’s authenticator and the Relying Party server. This allows them to:
- Replace the authenticator’s genuine attestation statement with a fake one.
- Substitute the user’s actual public key with their own.
- The Impact: The Relying Party would unknowingly register the attacker’s key, thinking it belongs to the user. The attacker could then impersonate the user in subsequent authentication attempts.
- Mitigation: Relying Parties MUST secure the registration ceremony using robust transport security like TLS to prevent tampering.
2. Limited Scope of Protection:
- The Issue: Attestation primarily focuses on the authenticator itself. It provides assurance about:
- The authenticator’s model/origin.
- How it stores and protects keys.
- What it Doesn’t Cover: Attestation DOESN’T guarantee the user’s identity or their intent. It doesn’t stop:
- Malware on the user’s device from manipulating WebAuthn interactions.
- A user from being tricked into authorizing a malicious request (e.g., through UI redressing).
- Mitigation: Relying Parties should implement additional security measures beyond WebAuthn:
- Strong account recovery mechanisms.
- Risk-based authentication to detect unusual activity.
- User education about phishing and social engineering.
3. No Proof of Private Key Possession at Registration:
- The Challenge: Most attestation types (except Self Attestation) don’t include a signature using the credential’s private key during registration.
- The Risk: An attacker who learns a user’s credential ID and public key could try to register them on a different account. If successful, the attacker might gain control if the credentials are discoverable.
- Mitigation: Relying Parties should:
- Reject duplicate credential IDs.
- Consider using Self Attestation for higher assurance, as it signs with the private key.
4. Reliance on External Trust Anchors:
- The Dependency: Attestation verification relies on trusted root certificates or metadata services (like the FIDO Metadata Service).
- The Weakness: If these trust anchors are compromised, attestation becomes unreliable.
- Mitigation: Relying Parties must carefully manage their trust anchors:
- Obtain them from reputable sources.
- Stay updated on any compromises or revocations.
In Summary:
Attestation statements are a valuable tool for enhancing WebAuthn security, but they are not a silver bullet. They provide assurance about the authenticator but have limitations in guaranteeing the user’s identity or preventing all forms of attacks. Relying Parties need a layered security approach to fully protect user accounts.
Question: What actions are recommended for Relying Parties when dealing with revoked attestation certificates?
According to the WebAuthn spec, when an attestation certificate validation fails because an intermediate attestation CA certificate is revoked, Relying Parties should take the following actions:
- Reject the Current Request: If the Relying Party’s policy dictates rejecting registration or authentication requests in such scenarios, the current request should be immediately denied.
- Un-register or Mark Existing Credentials: It’s highly RECOMMENDED that the Relying Party also un-register or mark as untrusted (equivalent to “self attestation”) any public key credentials registered after the CA compromise date. This applies to credentials using an attestation certificate chain linked to the revoked intermediate CA.
Key Points:
- Record Intermediate CAs: Relying Parties should store the intermediate attestation CA certificates during the registration process. This allows them to identify and take action on credentials associated with compromised CAs later.
- Policy Enforcement: The specific actions taken will depend on the Relying Party’s security policies and risk tolerance.
- User Experience: Consider the user experience when un-registering credentials. You might want to inform the affected users and provide guidance on registering new, trusted credentials.
Rationale:
This practice is essential to maintain the integrity of the WebAuthn system. Revoked attestation certificates indicate a potential security breach at the CA level. By rejecting requests and handling existing credentials appropriately, Relying Parties limit the risk of accepting compromised authenticators and improve overall security.
Question: How can Relying Parties mitigate the risk of credential loss and lack of key mobility?
The WebAuthn specification doesn’t inherently allow for credential private key backup or sharing between authenticators. This is a deliberate design choice to enhance security: keeping the private key locked to the authenticator that generated it greatly reduces the risk of compromise.
However, this design leads to a challenge: credential loss. If a user loses their authenticator, they lose all the credentials bound to it. This can lock them out of their accounts, especially if they only registered a single credential with each Relying Party.
To mitigate this risk, Relying Parties are strongly advised to:
- Encourage Multiple Credential Registration:
- During registration, Relying Parties SHOULD explicitly prompt users to register multiple credentials.
- This can involve explaining the benefits (e.g., account recovery, device continuity) and guiding users through the process.
- Support Diverse Authenticator Types:
- Relying Parties SHOULD support a range of authenticators, including:
- Platform Authenticators: Integrated into devices like laptops or phones (e.g., fingerprint sensors, Windows Hello).
- Roaming Authenticators: Separate devices like security keys or phones used via Bluetooth or NFC.
- This enables users to choose credentials based on their preferences and risk tolerance.
- Leverage
excludeCredentials
:
- During registration, utilize the
excludeCredentials
option innavigator.credentials.create()
. - This option allows Relying Parties to prevent the creation of a new credential on an authenticator that already holds a credential for the same user account.
- Effectively, it helps ensure that users register their multiple credentials on distinct authenticators, increasing resilience against loss.
- Utilize
user.id
Effectively:
- Employ the
user.id
parameter consistently to identify the user account. This helps authenticators understand when to replace existing credentials with new ones during registration. - By combining a unique
user.id
withexcludeCredentials
, Relying Parties can effectively manage multiple credentials per user without creating duplicates on the same authenticator.
- Guide Users on Credential Management:
- Provide clear instructions on how to manage registered credentials, including:
- Adding new authenticators.
- Removing lost or compromised authenticators.
- Recovering accounts in case of credential loss.
By following these best practices, Relying Parties can help their users:
- Create a more resilient authentication setup.
- Minimize the impact of credential loss.
- Maintain a strong security posture while offering flexibility and user convenience.
Question: What are the privacy risks associated with username enumeration, and how can Relying Parties address them?
Username enumeration is a privacy risk in WebAuthn (and in many authentication systems) where an attacker can gain information about which usernames are valid (i.e., have registered accounts) and which are not. This can happen even if the attacker can’t successfully log in.
Here’s a breakdown of the risks and how to address them:
Privacy Risks of Username Enumeration:
- Targeted Attacks: Attackers can identify valid usernames and focus their efforts on compromising those specific accounts. This makes attacks more efficient than random guessing.
- Data Correlation: By correlating enumerated usernames across multiple services, attackers might be able to build profiles of individuals and their online activities.
- Social Engineering: Knowledge of valid usernames can be used in social engineering attacks (e.g., phishing emails that appear more personalized).
- Reputation Damage: Leaking information about registered users could damage a Relying Party’s reputation, particularly if sensitive user data is involved.
How Relying Parties Can Address Username Enumeration:
The WebAuthn specification and general good security practices offer several mitigation techniques:
- Limit Information Disclosure:
- Consistent Error Messages: Provide generic error messages (e.g., “Invalid username or password”) for both incorrect usernames and incorrect passwords. Don’t distinguish between them.
- Delayed Responses: Introduce artificial delays in responses to authentication requests to make timing attacks more difficult.
- Verification Flow Changes:
- Multi-Step Authentication: Implement a multi-step process where the username is verified before proceeding to the WebAuthn challenge. This could involve:
- An initial password challenge (even a fake one if not actually used for authentication)
- Verifying a session cookie
- Sending a one-time code to an associated email address
- Plausible Imaginary Values:
- Fake Credentials: If no matching username is found, continue the authentication ceremony with imaginary but plausible WebAuthn credential options. This makes it harder for attackers to distinguish between real and fake accounts.
- Strong Username Policies:
- Restrict Email Addresses: Discourage or prevent users from registering with email addresses as usernames. This reduces the effectiveness of enumeration across multiple services.
- Long and Complex Usernames: Enforce longer and more complex usernames to make brute-force enumeration attempts less feasible.
- Rate Limiting:
- Throttle Requests: Limit the number of authentication attempts from a single IP address or user agent to slow down enumeration attacks.
Additional Recommendations:
- Monitoring and Logging: Monitor authentication logs for suspicious activity patterns that could indicate enumeration attempts.
- Security Audits: Regularly audit your authentication system for vulnerabilities and weaknesses that could facilitate username enumeration.
- User Education: Educate users about the risks of username enumeration and encourage them to choose strong and unique usernames.
Important Considerations:
- Trade-offs: Some mitigations might introduce usability challenges. Balance security with a smooth user experience.
- Context-Specific Solutions: The best combination of techniques will depend on your specific application, user base, and threat model.
By understanding the risks of username enumeration and implementing appropriate mitigations, Relying Parties can strengthen the privacy of their users’ authentication data.
Question: How can Relying Parties prevent information leakage via the allowCredentials argument?
The allowCredentials
argument in the WebAuthn API can pose a privacy risk, as it exposes a user’s credential IDs to the Relying Party before the user is authenticated. This can potentially leak information about the user’s other online accounts if they reuse authenticators and usernames.
Here’s how Relying Parties can prevent information leakage via the allowCredentials
argument:
1. Utilize Client-Side Discoverable Credentials:
- How it Works: Client-side discoverable credentials (also known as passkeys) eliminate the need for
allowCredentials
entirely. The authenticator can select the appropriate credential based on the RP ID, without the Relying Party needing to provide a list. - Benefits: This offers the strongest privacy protection as no credential IDs are exposed.
- Considerations: Requires user agent and authenticator support for discoverable credentials.
2. Employ a Multi-Step Authentication Process:
- How it Works: Instead of directly invoking WebAuthn as the first step, perform a preliminary authentication using a less sensitive method like:
- Username and password
- Session cookie
- Benefits: The user’s credential IDs are only exposed after they have been authenticated via the initial step.
- Considerations: Adds an extra authentication step, potentially impacting user experience.
3. Return “Imaginary” Credential IDs:
- How it Works: If forced to use
allowCredentials
as the first step, populate it with plausible, but fake, credential IDs derived deterministically from the username. - Benefits: An attacker observing
allowCredentials
won’t be able to distinguish between real and fake credentials. - Considerations: Attackers could potentially discern patterns in the fake IDs and use them to probe for real accounts. Ensure the imaginary values are sufficiently random and unpredictable.
4. Mask Authentication Failure Reasons:
- How it Works: When verifying an
AuthenticatorAssertionResponse
, make the error message indistinguishable regardless of whether the signature is invalid or the credential is unregistered. - Benefits: Prevents attackers from learning whether a specific credential ID is valid.
- Considerations: This can make troubleshooting authentication failures more difficult.
In Summary:
The best approach is to use client-side discoverable credentials whenever possible. If that’s not feasible, a multi-step authentication process provides a good balance between privacy and user experience. The other mitigation techniques can be useful when those two options are unavailable, but require careful implementation to be effective.
Question: What are the privacy implications of including personally identifying information within the user handle?
Including personally identifying information (PII) in the user handle has severe privacy implications for WebAuthn users. Here’s why:
What is a User Handle?
The user handle is an identifier for a user account, chosen by the Relying Party and passed to the authenticator during registration. While not displayed directly to the user, it’s stored by the authenticator and potentially returned to the Relying Party during specific authentication ceremonies (those with an empty allowCredentials
list, relying on discoverable credentials).
Privacy Risks of PII in User Handles
- Unnecessary Exposure: The user handle is not designed for user identification in most authentication scenarios. Using PII like email or username is unnecessary, as the credential ID already uniquely links the credential to the user account.
- Cross-Site Tracking: Exposing PII in the user handle allows malicious actors to potentially correlate a user’s activity across different websites. If two websites use the same PII (e.g., email address) as the user handle, and the user authenticates using a discoverable credential, the sites could link the user’s accounts.
- Authenticator Vulnerability: The WebAuthn specification explicitly states that authenticators MAY reveal user handles without requiring user verification. This means an attacker with access to the authenticator could potentially extract PII without the user’s knowledge or consent.
- Compromised Security: Storing PII in the user handle creates an additional data point that attackers can target. If an attacker compromises an authenticator or a Relying Party’s database, the exposed PII can be used for identity theft or targeted attacks.
Best Practices
- Randomize User Handles: The best practice is to generate a random, unique user handle for each account. This prevents cross-site tracking and minimizes the risk of PII exposure.
- Limit User Handle Exposure: Relying Parties should avoid authentication scenarios that require the authenticator to return the user handle.
- Educate Users: Users should be informed about the potential privacy risks of using authenticators that store PII in user handles, allowing them to make informed choices.
In conclusion, including personally identifying information in the user handle is a significant privacy risk that should be avoided at all costs. Relying Parties should prioritize user privacy by using random, unique user handles and limiting their exposure.
Question: What are the IANA registries related to WebAuthn, and what information do they contain?
WebAuthn, as a specification, relies on several registries maintained by IANA (Internet Assigned Numbers Authority) to ensure global interoperability and a structured approach to extensions. Here’s a breakdown of the IANA registries related to WebAuthn and their contents:
1. WebAuthn Attestation Statement Format Identifiers
- Purpose: This registry houses identifiers for various attestation statement formats supported by WebAuthn. These formats dictate how an authenticator cryptographically proves its origin and characteristics during credential creation.
- Information: Each entry in the registry contains:
- Attestation Statement Format Identifier: A unique string (e.g., “packed”, “tpm”, “android-key”) identifying the format.
- Description: A brief explanation of the format and its purpose.
- Specification Document: Reference to the section within the WebAuthn specification defining the format.
- Examples:
- “packed”: A compact and flexible format suitable for authenticators with limited resources.
- “tpm”: A format typically used by authenticators leveraging a Trusted Platform Module (TPM).
- “android-key”: Specific to Android platform authenticators using a hardware-backed attestation.
- “fido-u2f”: For compatibility with legacy FIDO U2F authenticators.
- “none”: Indicates no attestation is provided.
- “apple”: Proprietary format used by Apple devices for anonymous attestation.
2. WebAuthn Extension Identifiers
- Purpose: This registry lists identifiers for WebAuthn extensions. Extensions provide mechanisms to enhance or modify WebAuthn’s core functionality to suit specific use cases.
- Information: Each entry has:
- WebAuthn Extension Identifier: A unique string (e.g., “appid”, “uvm”, “largeBlob”) denoting the extension.
- Description: A concise overview of the extension’s functionality and usage.
- Specification Document: A reference to the section of the WebAuthn specification or external document that defines the extension.
- Examples:
- “appid”: Enables compatibility with legacy FIDO U2F credentials during authentication.
- “appidExclude”: Allows excluding legacy FIDO U2F credentials during registration.
- “credProps”: Facilitates communicating properties of a newly created credential to the Relying Party.
- “uvm”: Returns detailed information about the user verification method(s) employed by the authenticator.
- “largeBlob”: Enables storage and retrieval of larger opaque data blobs associated with a credential.
- “devicePubKey”: Provides a “device continuity” signal to Relying Parties for credentials that can be backed up.
Importance:
These IANA registries are crucial for the following reasons:
- Standardization: They ensure a consistent and globally recognized naming convention for attestation formats and extensions.
- Interoperability: Developers can rely on these identifiers to create authenticators and relying party implementations that work seamlessly across different platforms and browsers.
- Extensibility: The structured registration process allows for future innovations and the addition of new features to WebAuthn without breaking existing deployments.
You can find the most up-to-date information on these registries on the IANA website: https://www.iana.org/assignments/webauthn/
Question: How are attestation statement format identifiers registered and maintained by IANA?
You’re asking about the process for registering and maintaining Attestation Statement Format Identifiers within the IANA registry dedicated to WebAuthn. Here’s a breakdown:
The Registry
The IANA “WebAuthn Attestation Statement Format Identifiers” registry is the official repository for these identifiers. You can find it at https://www.iana.org/assignments/webauthn/. This registry is crucial for ensuring that different authenticators can communicate their attestation information in a standardized, interoperable way.
Registration Process
- Proposal: Someone, typically an authenticator manufacturer or a standards body, proposes a new attestation format. This proposal needs to include:
- A unique identifier string for the format (e.g., “packed”, “tpm”, “android-key”).
- A detailed specification of the format itself.
- Information about which attestation types the format supports.
- IETF Review: The proposal is submitted to the Internet Engineering Task Force (IETF). The IETF reviews the proposal to make sure it’s well-defined, technically sound, and meets interoperability requirements. The Web Authentication Working Group plays a key role in this evaluation.
- IANA Registration: Once the IETF approves the proposal, it’s passed on to IANA. IANA then adds the new format identifier and its associated information to the “WebAuthn Attestation Statement Format Identifiers” registry.
Maintenance
- Updates: If an existing attestation statement format needs to be revised or updated, a new proposal is submitted to the IETF for review, similar to the initial registration process.
- Deprecation: If a format becomes obsolete or insecure, it may be marked as deprecated in the registry.
Importance of Registration
- Interoperability: Registering attestation statement format identifiers with IANA is essential for ensuring that Relying Parties (websites or services using WebAuthn) can understand and verify the attestation information provided by different authenticators.
- Security: IANA registration provides a level of standardization and scrutiny that helps to enhance the security of WebAuthn.
Key Points
- IANA’s Role: IANA acts as the administrative body that maintains the registry but relies on the IETF’s technical expertise for evaluating new format proposals.
- Community-Driven: The registration and maintenance process is collaborative, involving authenticator manufacturers, standards bodies, and the broader security community.
Let me know if you have any more questions!
Question: Explain how Content Security Policy can enhance the security of WebAuthn implementations.
How Content Security Policy Enhances WebAuthn Security
Content Security Policy (CSP) is a powerful tool that can significantly bolster the security of WebAuthn implementations. Here’s a detailed explanation of how CSP helps:
1. Preventing Malicious Code Injection:
- Scope of WebAuthn Credentials: WebAuthn credentials are scoped to a specific origin, but malicious code running within that origin can potentially compromise them. This could happen through various means like:
- Third-party Scripts: Vulnerable third-party scripts included by the Relying Party could be exploited to inject malicious code.
- Subdomain Vulnerabilities: Malicious code hosted on a subdomain of the RP ID could exploit the credential scope rules to launch attacks.
- CSP’s Role: CSP helps mitigate this risk by allowing Relying Parties to define a strict whitelist of sources allowed to load and execute code on their origins. This limits the risk of malicious code injection by:
- Restricting Script Sources: You can specify exactly which domains are allowed to serve scripts using the
script-src
directive. - Blocking Inline Scripts: The
script-src
directive also allows you to block inline scripts, forcing all script execution to come from trusted sources. - Controlling Other Resources: CSP can also be used to control the loading of other resources like images, stylesheets, and fonts, further reducing the attack surface.
- Restricting Script Sources: You can specify exactly which domains are allowed to serve scripts using the
2. Protecting Against Cross-Site Scripting (XSS):
- XSS Threat: XSS attacks inject malicious scripts into otherwise trusted websites. If an attacker successfully launches an XSS attack on a Relying Party’s website, they could potentially:
- Steal user credentials.
- Hijack authentication sessions.
- Redirect users to phishing sites.
- CSP’s Role: While not a complete solution for XSS, CSP can significantly reduce the impact of such attacks.
- Restricting Script Execution: The
script-src
directive, along withobject-src
andframe-ancestors
, can help prevent malicious scripts from running within the WebAuthn context. - Enabling Reporting: CSP’s reporting mechanisms can help identify and address XSS vulnerabilities, allowing Relying Parties to react swiftly and patch their websites.
- Restricting Script Execution: The
3. Enforcing Subdomain Isolation:
- Subdomain Risk: As mentioned before, subdomains can pose a risk because malicious code on a subdomain can access credentials scoped to the parent domain.
- CSP’s Role: CSP provides granular control over subdomain interactions:
- Restricting Frames: The
frame-ancestors
directive allows you to prevent your website from being framed by other domains, including subdomains. - Isolating Subdomains: You can apply different CSP policies to specific subdomains, further isolating them from each other and the main domain.
- Restricting Frames: The
Best Practices for Using CSP with WebAuthn:
- Strong Script Whitelisting: Only allow scripts from highly trusted sources.
- Block Inline Scripts: Enforce external script loading to eliminate inline script injection vectors.
- Secure Communication: Ensure all communication with Relying Party servers happens over HTTPS.
- Robust Error Handling: Implement proper error handling mechanisms to deal with security policy violations.
- Regular Policy Review: Regularly review and update your CSP policies to adapt to evolving threats.
In conclusion, CSP is an essential layer of defense for WebAuthn implementations. It helps protect against code injection attacks, mitigates the impact of XSS vulnerabilities, and enforces strong origin isolation, significantly enhancing the overall security of WebAuthn authentication.
Question: What precautions should Relying Parties take when allowing subdomains in their WebAuthn origin validation?
The WebAuthn specification, for flexibility, allows Relying Parties to define the scope of their credentials using the RP ID. This means that a credential can be used on any origin whose effective domain is equal to the RP ID, or a registrable domain suffix of the RP ID.
This flexibility, however, introduces a significant security risk when combined with subdomains. If a Relying Party allows subdomain origins in its origin validation, a malicious actor could potentially:
- Inject malicious code: If the Relying Party hosts user-generated content or third-party scripts on a subdomain within the RP ID’s scope, an attacker could exploit vulnerabilities to inject code. This malicious code, running on a trusted origin, could then initiate WebAuthn requests and potentially steal user credentials.
- Launch man-in-the-middle attacks: By hosting a malicious website on a subdomain, an attacker can intercept WebAuthn requests meant for the Relying Party’s main domain. This allows them to potentially steal user credentials or manipulate the authentication process.
Therefore, Relying Parties should exercise extreme caution when allowing subdomains during WebAuthn origin validation. Here’s a breakdown of the precautions to take:
General Best Practices:
- Minimize Third-party Scripts: Limit the use of third-party scripts, especially on subdomains within the RP ID’s scope, as they can introduce vulnerabilities.
- Strong Content Security Policy (CSP): Implement a strict CSP to restrict the sources of scripts, objects, and other resources loaded on the Relying Party’s origins. This can help prevent malicious code injection.
- Regular Security Audits: Conduct regular security audits of all code and infrastructure, paying close attention to subdomains within the RP ID’s scope.
Subdomain-specific Precautions:
- Default to Disallowing Subdomains: Relying Parties should avoid allowing subdomains in their origin validation by default. Only deviate from this if absolutely necessary for specific functionality.
- Isolate Untrusted Code: If subdomains must be allowed, ensure they are strictly isolated and do not host any untrusted code, especially user-generated content.
- Separate Authentication Subdomains: Consider using dedicated subdomains specifically for authentication purposes. Implement rigorous security measures on these subdomains, treating them as highly sensitive environments.
Additional Considerations:
- User Education: Educate users about the potential risks associated with using WebAuthn on subdomains. Advise them to be cautious and verify the authenticity of the website before proceeding with authentication.
- Monitoring and Logging: Implement robust monitoring and logging mechanisms to detect suspicious activity related to WebAuthn requests, especially those originating from subdomains.
By taking these precautions, Relying Parties can mitigate the risks associated with allowing subdomains in WebAuthn origin validation while retaining some flexibility. However, it’s crucial to remember that the most secure approach is to generally disallow subdomains and only make exceptions after careful consideration and implementation of robust security measures.
Question: How can Relying Parties ensure they are validating the topOrigin member of the client data correctly?
The topOrigin
member in client data is crucial for Relying Parties that embed WebAuthn functionality within iframes, especially cross-origin ones. Validating it incorrectly exposes your application to potential security risks. Here’s a detailed breakdown of how to approach topOrigin
validation, tailored for different embedding scenarios:
Understanding topOrigin
- Purpose: The
topOrigin
field indicates the origin of the top-level browsing context where the WebAuthn ceremony was initiated. It’s ONLY present when the request originates from an iframe that’s cross-origin to the main page. - Security Implication: Without
topOrigin
, a malicious actor could embed your WebAuthn flow in their iframe, potentially tricking users into authenticating with your site while they believe they’re interacting with something else (UI Redressing or Clickjacking).
Validation Strategies
- No Embedding (Most Secure):
- Policy: You don’t allow your WebAuthn flows to be embedded at all.
- Validation:
- Require
topOrigin
to be absent, as your content shouldn’t be in an iframe. - Enforce that
origin
(the iframe’s origin) exactly matches your expected origin (e.g.,https://yourdomain.com
).
- Require
- Limited Embedding (Moderately Secure):
- Policy: You permit embedding on a specific, pre-defined list of trusted partner domains.
- Validation:
- If
topOrigin
is absent: Validateorigin
as in the “No Embedding” scenario. - If
topOrigin
is present: It MUST exactly match one of your allowed partner origins (e.g.,https://trustedpartner.com
).
- If
- Dynamic Embedding (Least Secure, More Complex):
- Policy: You allow embedding from a large number of domains or have a dynamic process to decide who can embed.
- Validation:
- If
topOrigin
is absent: Validateorigin
as before. - If
topOrigin
is present: This is the tricky part. You’ll need a robust mechanism to:- Parse the
topOrigin
string, extracting its scheme, host, and port. - Consult a dynamic policy system (e.g., a database lookup or an allowlisting service) to determine if this
topOrigin
is permitted at the time of the request.
- Parse the
- If
Crucial Security Considerations
- Subdomain Risks: Be extremely cautious about automatically trusting subdomains of your RP ID or your allowed partners. If you must allow subdomains, ensure they NEVER host untrusted user-generated content that could hijack your WebAuthn flow.
- Dynamic Policies: If you use dynamic embedding policies, design your systems to be secure against unauthorized modification or bypass. Regularly review and update your allowed origins.
- UI Redressing Mitigation: Even with correct
topOrigin
validation, UI Redressing remains a concern. Consider using techniques like the Intersection Observer API or pre-emptive popup windows to make your embedded UI harder to obscure.
Example Validation Code (Illustrative)
function validateTopOrigin(clientData, expectedOrigin, allowedPartners) {
if (clientData.topOrigin) {
if (allowedPartners.includes(clientData.topOrigin)) {
return true;
} else {
throw new Error("Unauthorized topOrigin");
}
} else {
if (clientData.origin === expectedOrigin) {
return true;
} else {
throw new Error("Invalid origin");
}
}
}
Key Points
- Don’t underestimate
topOrigin
— it’s vital for embedded scenarios. - Choose a validation strategy aligned with your embedding policy.
- Implement validation carefully, as errors can undermine security.
Let me know if you’d like to dive deeper into a specific embedding scenario, and I can provide more tailored guidance and code examples.
Question: How can a multi-step authentication process improve the overall security of WebAuthn ceremonies?
You’re absolutely right to ask about how multi-step authentication enhances WebAuthn security. Here’s a breakdown of how it works and the benefits:
Understanding the Vulnerability
WebAuthn, while incredibly robust, isn’t completely immune to certain attacks, especially in scenarios where an attacker has gained access to the user’s device or can intercept communications. Let’s consider these potential weaknesses:
- Device Compromise: If an attacker has physical access to a user’s device, they might be able to bypass WebAuthn if it’s the sole authentication factor.
- Phishing Attacks: Sophisticated phishing schemes can trick users into authenticating to a fake website, unknowingly handing over their WebAuthn assertion.
- Malware: Malware on a user’s device could potentially capture WebAuthn assertions or manipulate the authentication process.
Multi-Step Authentication as a Solution
Multi-step authentication (also known as multi-factor authentication or MFA) introduces additional layers of security by requiring users to prove their identity through multiple independent channels. Here’s how it strengthens WebAuthn:
- Defense in Depth: By combining WebAuthn (something you have) with other factors like a password (something you know) or a one-time code sent to a separate device (something you have), you significantly increase the difficulty for attackers. Even if one factor is compromised, the others remain as barriers.
- Phishing Mitigation: If a user is tricked into authenticating with WebAuthn on a phishing site, the additional factors (like a one-time code sent via SMS or email) won’t match the attacker’s system, thwarting the attack.
- Malware Resistance: While malware might be able to capture a WebAuthn assertion, it’s unlikely to also have access to the user’s password or a separate device to intercept a one-time code.
Practical Examples of Multi-Step WebAuthn
- WebAuthn + Password: The user first enters their username and password, and then uses their WebAuthn authenticator as a second step.
- WebAuthn + One-Time Code: After a user initiates a WebAuthn authentication, a unique code is sent to their registered email address or phone number. They must enter this code to complete the login.
- WebAuthn + Biometrics: Some devices allow for a combination of WebAuthn with biometric verification (fingerprint, face scan) built directly into the platform.
Key Considerations for Implementation
- User Experience: While multi-step authentication adds security, it can also add friction to the user experience. Strike a balance between security and convenience.
- Factor Independence: Ensure that the different authentication factors are truly independent. For instance, don’t send a one-time code to an email account that’s accessible from the same device as the WebAuthn authenticator.
- Risk-Based Authentication: Consider implementing risk-based authentication. This means evaluating user behavior and context to determine when to require additional authentication steps.
In Conclusion
Multi-step authentication is an essential practice to maximize the security of WebAuthn. By layering different authentication methods, you create a significantly more robust system that’s resilient to common attack vectors.
Question: Explain why guessing the challenge value in WebAuthn would compromise security.
Here’s why guessing the challenge value in WebAuthn would be disastrous for security:
Understanding the WebAuthn Challenge
- The Heart of Proof: The challenge is a unique, unpredictable value generated by the relying party (the website or service you’re trying to log into) for each authentication attempt.
- Proof of Possession: The authenticator (your security key, phone, or built-in biometric sensor) uses the challenge, along with its stored private key, to create a cryptographic signature. This signature proves that the authenticator possesses the private key that matches the public key registered with the relying party.
Why Guessing the Challenge Breaks Everything
- Replay Attacks: If an attacker could guess or predict the challenge, they could capture a legitimate authentication response (containing the signature) and then replay it later. This would allow them to impersonate the legitimate user without actually having the private key.
- Forged Assertions: A predictable challenge makes it possible for an attacker to forge a valid-looking signature even without the private key. This completely undermines the authentication process.
Real-World Analogy
Imagine a bank using a simple, predictable stamp to authorize transactions. If you knew the stamp’s pattern, you could easily forge a stamp and authorize fake transactions! The WebAuthn challenge is like a unique, one-time stamp, making forgery practically impossible.
How WebAuthn Prevents Challenge Guessing
- Randomness: WebAuthn mandates that challenges be generated using a cryptographically secure random number generator. This makes guessing virtually impossible.
- One-Time Use: Each challenge is valid for a single authentication attempt. A reused challenge would immediately raise red flags.
In Conclusion
The challenge’s unpredictability is the cornerstone of WebAuthn’s security. Without it, the entire system collapses, leaving users vulnerable to impersonation and account takeover.
Question: What minimum length is recommended for the challenge value to ensure adequate entropy?
The WebAuthn specification recommends that the challenge value should be at least 16 bytes long to ensure enough entropy and make guessing it infeasible, preventing replay attacks.
Question: What are the potential privacy risks associated with linking a user’s online identities through their credential IDs and public keys?
You’re right to be concerned about the privacy risks of linking a user’s online identities through their credential IDs and public keys. While designed to be anonymous and scoped to individual Relying Parties, there are some potential vulnerabilities:
1. Correlation by Malicious Actors:
- Multiple Malicious Relying Parties: If a user has accounts with multiple malicious Relying Parties, these parties could collude to share information about the user’s credential IDs and public keys. By comparing this data, they might be able to link the user’s identities across these services, even if usernames or emails are different.
- Combined with Other Identifying Information: Even if credential IDs themselves are not directly identifying, when combined with other pieces of information like IP addresses, browsing habits, or device fingerprints, it might be possible for a sophisticated attacker to build a profile and de-anonymize the user.
2. Weaknesses in Authenticator Implementations:
- Insufficient Entropy in Credential IDs: If an authenticator generates credential IDs with low entropy, it increases the chances of collisions, making it easier for malicious actors to guess or brute-force IDs and potentially link accounts.
- Bugs or Vulnerabilities: Security flaws in authenticator software or hardware could expose credential IDs or private keys, enabling unauthorized linking of identities.
3. Circumventing Scoping Mechanisms:
- Browser or OS Vulnerabilities: If a vulnerability exists in the user agent (browser or operating system) responsible for enforcing credential scope, it might be possible for malicious Relying Parties to access credentials intended for other parties, leading to identity linking.
4. User Behavior:
- Reusing Usernames/Emails: If a user uses the same username or email across multiple Relying Parties, this information alone can be used for linking, even without exploiting credential IDs.
Mitigation Strategies:
The WebAuthn specification incorporates various safeguards to minimize these risks:
- Scoping: Credentials are scoped to specific Relying Parties, preventing unauthorized access by other parties.
- Anonymity: Credential IDs are designed to be opaque and non-identifying.
- Strong Entropy: Authenticators should generate credential IDs with sufficient randomness to prevent collisions.
- Attestation Privacy: Measures are in place to prevent attestation certificates from uniquely identifying individual authenticators, hindering tracking.
Relying Party Responsibilities:
- Responsible Data Handling: Relying Parties should avoid collecting and storing unnecessary user data, minimizing the potential for correlation.
- Robust Security Practices: Implement strong security measures to prevent code injection attacks and protect user data from breaches.
- Username/Email Enumeration Prevention: Employ techniques to prevent attackers from enumerating usernames or emails (as discussed in §14.6.2 of the spec).
User Awareness:
- Unique Usernames/Emails: Users should consider using distinct usernames and emails for different services.
- Authenticator Selection: Choose reputable authenticators from trusted vendors with strong security track records.
By understanding these risks and implementing the appropriate safeguards, the WebAuthn ecosystem can aim to achieve a balance between strong authentication and user privacy.
Question: How does WebAuthn prevent malicious Relying Parties from discovering a user’s other identities or correlating them across different services?
WebAuthn employs several mechanisms to prevent malicious Relying Parties from discovering a user’s other identities or correlating them across different services:
1. Scoped Credentials:
- RP ID: Every WebAuthn credential is bound to a specific Relying Party Identifier (RP ID), which is typically the domain name of the website. This means a credential created for
example.com
can only be used for authentication onexample.com
and its subdomains, but not on other websites likemalicious.com
. - Client-Side Enforcement: The user agent (browser) enforces this scoping. It ensures that a Relying Party can only access credentials specifically registered for its RP ID. Attempts to access credentials outside this scope are blocked.
2. User Consent & Privacy:
- Explicit Authorization: A malicious Relying Party cannot silently probe for the existence of credentials. The user agent requires explicit user consent (typically through an authorization gesture like a biometric scan or PIN entry) before a credential is used for authentication.
- Information Leakage Prevention: The WebAuthn API is designed to prevent information leakage that might reveal the presence of credentials for other Relying Parties. For example, error responses are standardized to avoid revealing whether a credential exists but the user denied consent or the credential simply doesn’t exist.
3. Uncorrelatable Identifiers:
- Unique Credential IDs: Each credential has a probabilistically unique ID generated by the authenticator. These IDs are not reused across different Relying Parties, making it difficult to correlate a user based on their credential IDs.
- Authenticator Anonymization: Attestation, the process of verifying an authenticator’s origin, is designed to prevent tracking users based on unique authenticator identifiers. Attestation certificates are often batched, meaning many authenticators share the same certificate, or anonymization techniques are employed.
4. User Control:
- Credential Management: The user has control over their credentials. They can choose which authenticators to use and can delete credentials from their devices.
- Transparency: The user agent typically provides a user interface that shows which Relying Party is requesting access to a credential, allowing the user to make informed decisions.
In Summary:
WebAuthn’s combination of scoped credentials, user consent requirements, uncorrelatable identifiers, and user control significantly limits the ability of malicious Relying Parties to track users across services or discover their other identities. These privacy protections are a fundamental aspect of the WebAuthn standard.
Question: Explain how WebAuthn ensures user consent is obtained before revealing the existence of a public key credential to a Relying Party.
WebAuthn employs a multi-layered approach to guarantee user consent before disclosing the presence of a public key credential to a Relying Party. This is crucial to protect user privacy and prevent malicious actors from probing for credentials. Here’s a detailed breakdown:
1. Secure Contexts Only:
- The WebAuthn API is only exposed to websites operating within secure contexts (HTTPS). This ensures a secure channel between the user’s browser and the Relying Party’s server, preventing basic eavesdropping and manipulation attempts.
2. User Activation Requirement:
- For both registration (
navigator.credentials.create()
) and authentication (navigator.credentials.get()
), the browser enforces a user activation requirement. This means the user must actively initiate the process, typically by clicking a button or interacting with a specific UI element. - This prevents a website from silently and automatically probing for credentials in the background.
3. Authorization Gesture:
- Once a user activates the WebAuthn process, the authenticator (e.g., a security key or built-in platform authenticator) steps in.
- The authenticator then requires an explicit “authorization gesture” from the user before proceeding. This could involve:
- User Presence: A simple touch or interaction with the authenticator to confirm presence.
- User Verification: A stronger form of consent, requiring biometric authentication (fingerprint, face scan), a PIN, or a password on the authenticator itself.
- Only after the user provides this authorization gesture, does the authenticator proceed with the WebAuthn operation (creating a new credential or generating an assertion).
4. Timeout Mechanism:
- WebAuthn operations have a built-in timeout mechanism. If the user does not respond within the defined timeframe, the operation is automatically aborted, preventing a Relying Party from keeping a request pending indefinitely to wait for an unsuspecting user to interact with an authenticator.
5. Privacy-Preserving Error Handling:
- To avoid leaking information about the existence of credentials, error handling is carefully designed. If a user declines an authorization gesture, generic error messages are returned to the Relying Party, concealing whether the refusal was due to the absence of a credential or simply the user’s choice not to proceed.
In Summary:
WebAuthn requires a sequence of events to confirm user consent:
- User-Initiated: The user must initiate the process.
- Secure Channel: Communication happens over a secure channel (HTTPS).
- Explicit Consent: The user must actively authorize the operation through an authorization gesture on the authenticator.
- Time-Limited: Operations have timeouts to prevent indefinite waiting.
- Opaque Errors: Generic error messages protect user privacy.
This comprehensive mechanism ensures that a Relying Party only learns about the existence of a public key credential if the user has explicitly and knowingly consented to its use.
Question: How does WebAuthn prevent the leakage of user biometric data to Relying Parties?
WebAuthn cleverly prevents the leakage of user biometric data to Relying Parties by keeping the biometric verification process entirely local to the authenticator. Here’s how it works:
- Biometric Data Stays on the Authenticator: The biometric data (fingerprint, facial scan, etc.) is captured and processed within the authenticator itself. It never leaves the secure confines of the authenticator, whether it’s a built-in platform authenticator or a roaming device like a security key.
- User Verification, Not Data Transmission: Instead of sending the biometric data to the Relying Party, the authenticator performs a local verification. It checks if the presented biometric matches its stored template. The result of this check, a simple “yes” or “no”, is what gets communicated.
- UV Flag Signals Verification: The “yes” outcome of the biometric verification is signaled to the Relying Party through the
UV
(User Verified) flag within the authenticator’s response. This flag is part of the digitally signed data, assuring the Relying Party that user verification occurred.
Key Points for Enhanced Privacy:
- No Centralized Biometric Database: WebAuthn eliminates the need for Relying Parties to store user biometric data, removing the risk of large-scale breaches exposing this sensitive information.
- Reduced Attack Surface: By keeping biometric data local, WebAuthn minimizes the attack surface for malicious actors seeking to steal biometric information.
- User Control: Users have control over their biometric data. They can choose which authenticators to trust and how their biometrics are used for verification.
In essence, WebAuthn treats biometric verification as an internal process of the authenticator. The Relying Party only receives confirmation that the verification was successful, not the actual biometric data itself. This design ensures enhanced user privacy and minimizes the risks associated with handling sensitive biometric information.
Question: What measures are taken to prevent the correlation of users between Relying Parties based on their authenticato
The WebAuthn specification takes several measures to prevent Relying Parties from correlating users across different websites based on their authenticators:
1. Scoped Credentials:
- Relying Party ID (RP ID): Each credential is bound to a specific RP ID, which is usually the domain of the website. This means that a credential generated for
example.com
can’t be used onanothersite.com
. - Authenticator Isolation: Authenticators maintain strict isolation between credentials for different RP IDs. Even if a user uses the same authenticator on multiple websites, those websites cannot directly see or interact with credentials meant for other websites.
2. Minimizing Identifying Information in Attestation:
- Batch Attestation: Authenticators can be manufactured in batches that share the same attestation certificate. This means that multiple authenticators will appear identical to Relying Parties, making it harder to track individual users based on their unique device.
- Anonymization CA: Some authenticators employ Anonymization Certificate Authorities (AnonCA). These CAs issue unique attestation certificates for each credential, making it practically impossible to link a user’s credentials across different Relying Parties based on those certificates.
- Self Attestation: In cases where extra privacy is needed or the authenticator doesn’t have a dedicated attestation key, self attestation is used. Here, the credential’s own private key is used for attestation, meaning no external identifying information is shared with the Relying Party.
3. Client-Side Enforcement:
- User Agent as Intermediary: The user agent (browser) acts as a gatekeeper, mediating all interactions between the authenticator and Relying Parties. This prevents websites from directly probing authenticators for information or attempting to access credentials outside their scope.
- User Consent: The user agent requires explicit user consent before a credential is created or used for authentication. This gives users control over what information is shared with websites.
4. Additional Privacy Considerations:
- Credential IDs: Credential IDs are generated by the authenticator and are meant to be opaque and unlinkable across different Relying Parties.
- User Handles: Relying Parties are encouraged to use randomly generated, non-identifying user handles instead of usernames or email addresses, further reducing the possibility of correlation.
Summary:
These combined measures significantly reduce the risk of user tracking based on authenticator data. However, no system is perfectly foolproof. Users should be aware that other factors, such as using the same username across multiple websites, can still contribute to cross-site tracking.
Question: Explain the privacy risks associated with returning distinguishable errors in [[Create]]() and [[DiscoverFromExternalSource]]() methods.
Privacy Risks of Distinguishable Errors in WebAuthn
The WebAuthn specification emphasizes user privacy, ensuring that relying parties can’t glean sensitive information about a user’s authenticators without explicit consent. One crucial aspect of this is the handling of errors in the [[Create]]()
and [[DiscoverFromExternalSource]]()
methods. These methods, respectively responsible for registering new credentials and using existing ones, can unintentionally leak information if their error responses are distinguishable.
Here’s a breakdown of the privacy risks associated with distinguishable errors in these methods:
[[Create]]()
- Scenario: Imagine a malicious relying party wants to know if a user has a specific credential registered from a previous interaction. During registration (
[[Create]]()
), they include this credential in theexcludeCredentials
list. - Distinguishable Errors:
- Case 1: No authenticators present: The browser might return a specific error indicating no authenticators are available.
- Case 2: Excluded authenticator present: If the user has the targeted credential, the browser might immediately return a different error (perhaps “InvalidStateError”) as soon as it detects the excluded authenticator.
- Privacy Risk: The malicious relying party can now distinguish between the two cases. If the error response suggests an immediate cancellation (before the timeout and likely user action), they can infer that the user possesses the credential listed in
excludeCredentials
. This leaks information about the user’s authentication history without their consent.
[[DiscoverFromExternalSource]]()
- Scenario: A malicious relying party tries to ascertain if a user has a specific credential available. They include this credential in the
allowCredentials
list during authentication ([[DiscoverFromExternalSource]]()
). - Distinguishable Errors:
- Case 1: Named credential not available: The browser might return a general “NotAllowedError” after the timeout, indicating no suitable credential was found.
- Case 2: Named credential present, user denies consent: If the user has the credential but declines to use it, the browser might return a different “NotAllowedError” immediately.
- Privacy Risk: The malicious relying party can differentiate between the errors. A fast error response strongly suggests the user has the credential but chose not to use it, revealing information about their available authenticators without consent.
Mitigations:
The WebAuthn specification strongly recommends clients to make these error responses indistinguishable. This can be achieved by:
- Uniform error responses: Returning a generic “NotAllowedError” in all error scenarios, regardless of the cause.
- Delaying error responses: Even if different errors are used internally, delay the response until the timeout expires in all cases. This ensures the relying party cannot deduce anything from the timing.
In Conclusion: Distinguishable errors in [[Create]]()
and [[DiscoverFromExternalSource]]()
create vulnerabilities that can be exploited to reveal sensitive user information. By making error responses uniform and delaying them, client implementations can protect user privacy and uphold the security model of WebAuthn.
Question: What are the possible privacy leaks related to the length and number of credential IDs in the allowCredentials argument?
You’re right to be concerned about the privacy leaks related to the allowCredentials
argument. Here’s a breakdown of how the length and number of credential IDs within it can be exploited:
1. Correlation via Credential ID Length
- Different authenticators, different lengths: Credential IDs generated by various authenticators often have different lengths. For instance, a credential ID from a security key might be shorter than one generated by a platform authenticator.
- Building a fingerprint: An attacker observing multiple
allowCredentials
requests for the same user across different websites can start to build a “fingerprint” of the user’s authenticators based on the lengths of the IDs. - Cross-site tracking: Even if usernames differ across sites, this fingerprint can be used to link a user’s activity and potentially identify them.
2. Correlation via Number of Credential IDs
- Multiple authenticators, more IDs: A user with multiple registered authenticators will naturally have more credential IDs in their
allowCredentials
list. - Unique pattern: The number of credentials itself, combined with their varying lengths, creates a more unique pattern associated with the user.
- Enhanced tracking: This enhances the attacker’s ability to track the user across different Relying Parties.
Mitigations
The WebAuthn specification highlights these privacy risks and recommends several mitigations for Relying Parties:
- Prior Authentication: Perform an initial authentication step (like username/password or cookie-based authentication) before invoking WebAuthn and revealing
allowCredentials
. This ensures credential IDs are exposed only after the user is authenticated. - Discoverable Credentials: Favor client-side discoverable credentials (passkeys). These credentials don’t require
allowCredentials
, eliminating the leak entirely. - Imaginary Credentials: If you must expose
allowCredentials
early in the flow, consider populating it with plausible but fake credential IDs. This makes it difficult for attackers to distinguish between real and fake requests, hindering their ability to track users.
Important Note: Implementing these mitigations requires careful consideration. Imaginary credentials, for instance, must be generated in a way that prevents attackers from easily recognizing them as fake.
Let me know if you’d like to dive deeper into any of these mitigation techniques!
Question: How can the use of plausible imaginary values mitigate privacy risks during registration and authentication?
The WebAuthn specification highlights a privacy risk during both registration and authentication ceremonies called username enumeration. This happens when an attacker can probe a Relying Party’s system to determine whether specific usernames are registered. Here’s how it works and how plausible imaginary values can help:
The Problem: Username Enumeration
- Attacker Probes: An attacker tries to register or authenticate using common usernames (e.g., “johndoe”, “janesmith”, “testuser”).
- Relying Party Response: The Relying Party will respond differently depending on whether the username exists:
- Existing Username: The Relying Party proceeds with the registration/authentication flow, possibly asking for more information.
- Non-Existing Username: The Relying Party immediately returns an error, indicating the username isn’t found.
- Information Leak: This difference in response allows the attacker to build a list of valid usernames, even if they fail to complete the registration/authentication.
Mitigating with Plausible Imaginary Values
Instead of immediately returning an error for non-existing usernames, the Relying Party can:
- Craft a Fake Response: Create a
PublicKeyCredentialCreationOptions
orPublicKeyCredentialRequestOptions
object with plausible but imaginary values. These values should resemble genuine responses but not correspond to an actual user. - Continue the Ceremony: Proceed with the ceremony as if the username existed, interacting with the authenticator using the fake data.
- Controlled Failure: After the interaction with the authenticator, return a generic error that doesn’t reveal whether the username was the cause of the failure.
Why this Works
- Uniform Response: By always returning a similar response, the attacker can’t distinguish between existing and non-existing usernames based on the initial interaction.
- Mimicking Real Flow: The use of imaginary values makes the fake flow closely resemble a genuine one, further obscuring the true reason for the eventual failure.
Example
An attacker tries to authenticate with “johndoe”. Instead of returning “Username not found”, the Relying Party:
- Generates a fake challenge.
- Creates a fake list of allowed credentials.
- Proceeds to interact with the authenticator using this fake data.
- Finally, returns a generic “Authentication failed” error.
Important Considerations
- Plausibility is Key: The imaginary values need to be convincing enough to fool an attacker. Using predictable or constant values might allow the attacker to differentiate between real and fake responses.
- Not a Silver Bullet: This technique helps mitigate username enumeration but doesn’t completely eliminate it. Attackers could still use other methods to try and guess usernames.
In Summary:
Plausible imaginary values can enhance privacy by making the initial stages of registration and authentication ceremonies uniform, regardless of whether the provided username exists. This helps to prevent attackers from gleaning information about registered usernames through probing.
Question: What privacy considerations should clients take into account when handling platform credentials on multi-user operating systems?
The WebAuthn specification highlights a crucial privacy concern for clients handling platform credentials on multi-user operating systems:
Preventing Cross-Account Access:
The primary concern is ensuring that a user’s platform credentials are only accessible to that specific user’s operating system account. Here’s why this is vital and how to address it:
- Risk: If not properly isolated, a user logged into one OS account could potentially access the platform credentials (and thus the accounts) of another user on the same device. This undermines the security benefits of platform authenticators as a “something you have” factor.
- Mitigation: The client (typically the browser or OS-level WebAuthn implementation) must enforce strict separation of platform credential access based on OS user accounts. This might involve:
- Secure storage: Storing platform credentials within a user-specific protected area of the operating system, inaccessible to other users.
- OS-level API integration: Utilizing OS-level APIs to manage and isolate platform credentials on a per-user basis.
- User-specific UI prompts: Displaying authentication prompts (e.g., for biometrics or PIN) only to the OS user associated with the requested credential.
Additional Considerations:
- Clear User Communication: The client should clearly indicate to the user which OS account is being used for WebAuthn operations. This helps prevent confusion and potential security issues if multiple users are logged in.
- Authenticator Management: Provide mechanisms for users to manage their own platform credentials within their respective OS accounts. This might include viewing, deleting, or resetting credentials.
- Backup and Recovery: Account recovery processes should be robust enough to handle scenarios where a user loses access to their OS account but needs to regain access to their WebAuthn-protected accounts.
In essence, the client must act as a gatekeeper, ensuring platform credentials remain firmly tied to the correct OS user, maintaining the intended privacy and security boundaries between users on a shared device.
Question: Why is it important for user verification-capable authenticators to offer multiple verification methods?
The WebAuthn specification strongly recommends that user verification-capable authenticators offer multiple verification methods. Here’s why this is crucial:
1. Accessibility and Inclusivity:
- Diverse Needs and Preferences: Users have different abilities and preferences. Some might find fingerprint scanning convenient, while others might prefer PIN entry or facial recognition. Offering a variety of options ensures a broader range of users can effectively and comfortably interact with the authentication process.
- Situational Limitations: Certain situations might render a specific verification method unusable. A cut on your finger could prevent fingerprint recognition, a noisy environment might interfere with voice recognition, or a bright light could hinder facial recognition. Having alternative methods ensures users aren’t locked out of their accounts.
2. Enhanced Security and Fallback Mechanisms:
- Compromised Methods: If one verification method is compromised (e.g., a stolen fingerprint), the user can fall back to a different, more secure method. This provides redundancy and strengthens overall security.
- Authenticator Errors: Occasionally, authenticators might experience technical issues with a specific method. Multiple options provide backup in case of such malfunctions.
3. User Experience and Convenience:
- Flexibility and Choice: Users appreciate having the freedom to choose the method that best suits their current circumstances and preferences.
- Smooth Authentication Flow: Providing multiple methods ensures a smoother authentication experience, reducing frustration and increasing the likelihood of successful logins.
Example:
Imagine a user who primarily relies on fingerprint authentication. If their finger is injured, they might be unable to log in. If the authenticator also supports PIN entry, they can easily switch to this alternative method without being locked out of their account.
In essence, offering multiple user verification methods promotes accessibility, strengthens security, and enhances the overall user experience. This aligns with WebAuthn’s core principles of providing robust and user-friendly authentication.
Question: What affordances can Relying Parties provide to help users complete authorization gestures correctly?
The WebAuthn specification, while robust, leaves the specific implementation of user affordances during authorization gestures largely up to the client platform (browser, operating system) and the authenticator itself. However, the spec does encourage Relying Parties (websites or services using WebAuthn) to provide helpful information during the registration process that can assist users later on. Here are some key affordances Relying Parties can implement:
During Registration:
- Meaningful Authenticator Naming: Allow users to provide a friendly name for their authenticator (e.g., “My YubiKey,” “Work Phone,” “Alice’s Fingerprint”). This name will be displayed by the client during authentication, making it easier for users to identify the correct authenticator.
- Authenticator Icon/Image: Let users choose or upload an image to represent their authenticator. This visual cue, shown during authentication, can help users quickly pick the right device, especially when multiple similar authenticators are present.
- Freeform Text Instructions: Provide a field for users to enter their own instructions or reminders. This could include details like:
- The specific gesture needed (e.g., “Tap the button twice,” “Scan your left thumb”).
- The location of the authenticator if it’s not always readily accessible.
- Any other unique characteristic of the authenticator or the required gesture.
- Step-by-Step Guidance: During the registration process, clearly guide the user through the process of interacting with their authenticator. Explain what prompts to expect and what actions they need to take on the device.
General Best Practices:
- Clear and Concise Language: Use plain language in prompts and instructions. Avoid technical jargon and make sure the user understands what is being asked of them.
- Contextual Information: When requesting an authentication gesture, provide the user with context about why it’s needed (e.g., “Sign in to your account”, “Confirm this purchase”).
Important Considerations:
- Security vs. Usability: While detailed instructions can be helpful, avoid overly revealing specific details about the authenticator or the gesture in a way that could compromise security.
- Client and Authenticator Limitations: Remember that the level of customization for prompts and UI varies between clients and authenticators. Some may offer more flexibility than others.
By providing the right information and guidance upfront, Relying Parties can significantly enhance the user experience and reduce errors during WebAuthn authorization gestures.
Question: What considerations should clients take into account when adjusting Relying Party-supplied timeouts for accessibility purposes?
The WebAuthn specification recommends that clients adjust Relying Party-supplied timeouts to meet accessibility guidelines, particularly [WCAG 2.1]’s Guideline 2.2: Enough Time. Here’s a breakdown of the considerations clients should take into account:
Understanding WCAG 2.2: Enough Time
This guideline aims to ensure users with disabilities have sufficient time to complete tasks without unexpected time limits causing difficulties. It recognizes that some users may require more time due to:
- Physical limitations: Difficulties with motor control, dexterity, or speed.
- Cognitive disabilities: Challenges with processing information, making decisions, or understanding instructions.
- Visual impairments: Users relying on screen readers or magnification may take longer to navigate and interact with content.
Considerations for Adjusting Timeouts
- User Needs: The primary consideration is understanding the diverse needs of users with disabilities. Research and user testing can help determine appropriate timeout adjustments.
- Authenticator Type:
- Platform Authenticators: These are integrated into the device (fingerprint reader, face ID). Timeout adjustments might need to account for OS-level prompts and interactions.
- Roaming Authenticators: These are external devices (security keys). Timeouts need to consider the time it takes for users to locate, connect, and interact with the device.
- User Verification Method: Different methods (PIN entry, biometrics) have varying time requirements. Complex gestures or multi-step verification will need longer timeouts.
- Context of the Ceremony:
- Registration Ceremony: Allow ample time for users to choose an authenticator, understand prompts, and complete the registration process.
- Authentication Ceremony: Consider the user’s potential need to locate an external authenticator, perform user verification, and confirm the transaction.
- Avoiding Excessive Timeouts: While extending timeouts is important, avoid making them so long that they create security risks (e.g., allowing an attacker more time to exploit a vulnerability) or negatively impact performance.
Implementation Guidance
- Default Timeouts: Clients SHOULD establish reasonable default timeouts for WebAuthn ceremonies, considering accessibility guidelines.
- Relying Party Hints: Pay attention to any hints provided by the Relying Party in the
hints
parameter. For example, aclient-device
hint suggests a platform authenticator might be used, potentially influencing timeout adjustment. - User Feedback: Allow users to extend timeouts if needed. This could involve providing a “Need More Time” option or a mechanism to customize timeout settings.
- Transparency: Document timeout adjustment logic and communicate changes to Relying Parties and users to promote understanding and avoid confusion.
Example:
A Relying Party sets a timeout of 30 seconds for an authentication ceremony. The client detects that the user is relying on a screen reader and has a platform authenticator with fingerprint verification. Based on its understanding of accessibility needs, the client extends the timeout to 60 seconds, allowing the user sufficient time to respond to OS prompts, navigate the authentication dialog with their screen reader, and perform fingerprint verification.
In Summary: Client implementations should prioritize user needs and accessibility guidelines when adjusting Relying Party-supplied timeouts. Carefully consider the context of the ceremony, the authenticator type, and the user verification method to determine appropriate timeout extensions while balancing security and performance considerations.
Question: How can Virtual Authenticators be used to simulate different authenticator types and capabilities for testing purposes?
The WebAuthn WebDriver extension, as defined in the WebAuthn Level 3 specification, introduces the concept of Virtual Authenticators for testing purposes. These software-based entities mimic the behavior of real physical authenticators, allowing developers to simulate various scenarios without needing physical devices.
Here’s a breakdown of how Virtual Authenticators can simulate different authenticator types and capabilities:
1. Defining Authenticator Capabilities:
The Add Virtual Authenticator
command lets you create a virtual authenticator with specific properties, mirroring the characteristics of real-world devices:
- Protocol: You can specify the protocol version, choosing from “ctap1/u2f”, “ctap2”, or “ctap2_1”. This dictates the feature set available, allowing you to test compatibility with different WebAuthn implementations.
- Transport: You can simulate different transports like “usb”, “nfc”, “ble”, “internal” (platform authenticator), etc. This tests your application’s handling of diverse connection mechanisms.
- Resident Key Support: By toggling
hasResidentKey
, you can simulate authenticators that support discoverable credentials (“passkeys”) or those that only work with server-side credentials. - User Verification: The
hasUserVerification
flag controls whether the virtual authenticator supports PINs, biometrics, or other user verification mechanisms. - User Consent and Verification Results: You can pre-determine the outcome of user interactions by setting
isUserConsenting
andisUserVerified
. This helps test both successful and failed registration/authentication flows. - Extensions: You can choose which extensions the virtual authenticator supports (e.g., “uvm”, “prf”, “largeBlob”). This is crucial for testing extension-specific functionality.
2. Injecting Credentials:
The Add Credential
command allows you to populate a virtual authenticator with pre-defined credentials:
- Credential ID: You can inject a specific credential ID, useful for testing scenarios with known credential identifiers.
- Credential Type: You can choose to create a discoverable (resident) or server-side (non-resident) credential.
- RP ID: Define the Relying Party ID to which this credential is scoped, ensuring the virtual authenticator behaves correctly in relation to the website being tested.
- Private Key: You provide the private key associated with the credential, allowing for the generation of valid signatures during simulated authentication ceremonies.
- User Handle, Sign Count, Large Blob: You can optionally add a user handle, set an initial signature count, and even include a “large blob” to test those specific features.
3. Simulating User Interactions:
- By setting
isUserConsenting
totrue
, you automatically approve all user prompts, speeding up test execution. Setting it tofalse
forces ceremonies to fail due to lack of user consent. - The
isUserVerified
property, in combination withhasUserVerification
, lets you simulate scenarios where user verification is successful or fails.
4. Comprehensive Testing:
By combining these capabilities, you can thoroughly test various scenarios:
- Different Authenticator Types: Simulate security keys, platform authenticators, hybrid authenticators (like smartphones), and even legacy U2F devices.
- User Verification Flows: Test PIN entry, biometric authentication, and user presence checks, including both successful and failed attempts.
- Credential Management: Experiment with discoverable credentials (passkeys) and server-side credentials, including registration, authentication, and potential error conditions.
- Extension Compatibility: Ensure your application correctly interacts with different extensions and handles situations where extensions are not supported.
In essence, Virtual Authenticators provide a powerful tool for web developers to create a controlled testing environment, allowing them to build robust and reliable WebAuthn implementations that function correctly across a wide range of authenticators and user interactions.
Question: Explain the process of injecting a Public Key Credential Source into a Virtual Authenticator using the “Add Credential” command.
The “Add Credential” command in the WebAuthn WebDriver extension allows you to inject a Public Key Credential Source into a Virtual Authenticator, essentially simulating the creation of a credential on a real authenticator. This is extremely valuable for testing WebAuthn Relying Party applications without needing actual physical authenticators. Here’s a breakdown of the process:
1. Command Structure
- HTTP Method:
POST
- URI Template:
/session/{session id}/webauthn/authenticator/{authenticatorId}/credential
2. Credential Parameters (Payload)
You send a JSON object called “Credential Parameters” as the payload for this command. This object defines the properties of the credential you are injecting. Here’s a description of each key:
credentialId
: A string containing the Credential ID of the public key credential. This ID should be encoded using Base64url Encoding.isResidentCredential
: A boolean value that determines the type of credential:true
: Creates a client-side discoverable credential (also known as a “resident credential” or “passkey”).false
: Creates a server-side credential.
rpId
: A string representing the Relying Party ID the credential is associated with. This should be a valid domain string.privateKey
: A string containing a Base64url encoded representation of the credential’s private key. This must follow the [RFC5958] standard for asymmetric key packages, specifically using a single ECDSA private key on the P-256 curve.userHandle
: An optional string containing the user handle associated with the credential, encoded using Base64url Encoding. This property is only required for client-side discoverable credentials (isResidentCredential: true
).signCount
: A number representing the initial value of the signature counter for this credential.largeBlob
: An optional string containing a Base64url encoded opaque byte string that the Relying Party wants to store with the credential. This is used for simulating the largeBlob extension of WebAuthn.
3. Remote End Steps
When the WebDriver server (which is controlling the browser) receives this command, it performs the following steps:
- Validation:
- The server verifies that the received parameters are a valid JSON object and that each property is present with the correct value type and format.
- It decodes the Base64url encoded
credentialId
,privateKey
, anduserHandle
. - It ensures that the
rpId
is a valid domain string. - If
largeBlob
is present, it is also decoded from Base64url encoding.
- Authenticator Check:
- The server confirms that the
authenticatorId
provided in the URI template matches an existing Virtual Authenticator in its Virtual Authenticator Database. - It checks if the Virtual Authenticator supports the requested credential type (
isResidentCredential
). If you’re trying to inject a client-side discoverable credential, the Virtual Authenticator must havehasResidentKey
set totrue
. - If the
largeBlob
property is specified, it verifies that the Virtual Authenticator supports the largeBlob extension.
- The server confirms that the
- Credential Creation:
- The server creates a new Public Key Credential Source object (either client-side discoverable or server-side based on
isResidentCredential
) and populates it with the values from the decodedcredentialId
,privateKey
,rpId
, anduserHandle
(if present). - A signature counter is associated with this new credential and initialized with the value from
signCount
, defaulting to 0 if not provided. - If
largeBlob
is specified, it is associated with the credential.
- The server creates a new Public Key Credential Source object (either client-side discoverable or server-side based on
- Storage:
- The newly created credential and its associated counter are stored within the Virtual Authenticator’s database.
- Response:
- The server returns a “success” response to confirm that the credential was successfully injected.
In Summary
The “Add Credential” command in the WebAuthn WebDriver extension provides a powerful way to set up specific credential scenarios within a virtual environment for testing purposes. It allows you to define all the necessary parameters of a credential and simulate its presence on a virtual authenticator, enabling thorough and controlled testing of your WebAuthn implementations.
Question: How can the “Get Credentials” command be used to inspect the credentials stored within a Virtual Authenticator?
The “Get Credentials” command, defined in the WebAuthn WebDriver extension, allows you to retrieve details about every Public Key Credential Source stored within a specified Virtual Authenticator. This is how it can be used for inspection:
- Target a Virtual Authenticator: You need the
authenticatorId
of the Virtual Authenticator you want to inspect. This ID was returned when you created the Virtual Authenticator using the “Add Virtual Authenticator” command. - Execute the Command: Send a GET request to the endpoint
/session/{session id}/webauthn/authenticator/{authenticatorId}/credentials
. Replace{session id}
with the current WebDriver session ID and{authenticatorId}
with the ID obtained in step 1. - Process the Response: A successful response will contain a JSON array named
credentialsArray
. Each element in this array is aCredential Parameters
object representing a credential stored within the Virtual Authenticator. - Inspect the Credential Details: Each
Credential Parameters
object provides the following information about the credential:
credentialId
: The credential ID, Base64url encoded.isResidentCredential
: A boolean indicating whether this is a client-side discoverable credential (true
) or a server-side credential (false
).rpId
: The Relying Party ID associated with this credential.privateKey
: The private key portion of the credential key pair, encoded as an asymmetric key package using Base64url encoding (per RFC 5958). Important Note: Accessing the private key in this manner is only possible because this is a Virtual Authenticator designed for testing. Real authenticators would never expose the private key.userHandle
: The user handle (Base64url encoded) associated with the credential (this might not be present).signCount
: The current value of the signature counter for the credential.largeBlob
: If the Virtual Authenticator supports thelargeBlob
extension, this field (Base64url encoded) will hold any large blob data associated with the credential.
Use Cases for Inspection:
- Test Credential Creation: After using the WebAuthn API to register a new credential within a Virtual Authenticator, you can use “Get Credentials” to confirm that the credential was stored with the correct parameters.
- Simulate Account Recovery: By inspecting the credential details, you can simulate scenarios where a user loses their authenticator and needs to recover their account using, for example, a backup credential.
- Debug Security Issues: If you encounter unexpected behavior during WebAuthn registration or authentication, you can use “Get Credentials” to examine the internal state of the Virtual Authenticator and pinpoint potential problems.
Remember: Virtual Authenticators are specifically for testing. They provide a controlled environment to inspect and manipulate credentials. This level of access is never possible with real-world authenticators, which are designed to keep private key material strictly confidential.
Question: What are the steps involved in removing a specific credential from a Virtual Authenticator using the “Remove Credential” command?
The “Remove Credential” WebDriver extension command is used to delete a specific public key credential source from a virtual authenticator. Here are the steps involved, based on the WebAuthn spec:
1. Command Structure:
- HTTP Method: DELETE
- URI Template:
/session/{session id}/webauthn/authenticator/{authenticatorId}/credentials/{credentialId}
2. Input Parameters:
- authenticatorId: A string that uniquely identifies the virtual authenticator from which the credential should be removed.
- credentialId: A string containing the base64url encoded Credential ID of the credential to be removed.
3. Remote End Steps
The WebDriver endpoint receiving this command performs the following steps:
a) Validate authenticatorId:
* Check if authenticatorId
matches any virtual authenticator in the Virtual Authenticator Database.
* If no match is found, return a WebDriver error with error code invalid argument
.
b) Locate Authenticator:
* Retrieve the virtual authenticator object identified by authenticatorId
from the Virtual Authenticator Database.
c) Validate credentialId:
* Check if credentialId
matches any public key credential source managed by the retrieved virtual authenticator.
* If no match is found, return a WebDriver error with error code invalid argument
.
d) Remove Credential:
* Delete the public key credential source identified by credentialId
from the virtual authenticator’s database.
e) Return Success:
* Return a success response to indicate that the credential has been successfully removed.
In summary, the process involves validating the input parameters, locating the authenticator and the specific credential, deleting the credential, and then returning a success response.
Question: How can the “Set User Verified” command be used to simulate successful user verification during automated testing?
The “Set User Verified” command is essential for simulating successful user verification scenarios in a testing environment. Here’s a breakdown of how it works and how it contributes to comprehensive WebAuthn testing:
Understanding User Verification
- User verification is a crucial step in WebAuthn. It ensures that the person attempting to use a credential is indeed the authorized user. This is often achieved through biometrics (fingerprint, face scan), PIN codes, or other secure methods.
- During real-world authentication, the authenticator (e.g., a security key or the user’s device) is responsible for handling user verification.
The “Set User Verified” Command
- In automated testing, we don’t have a real user physically interacting with an authenticator. The “Set User Verified” WebDriver extension command addresses this gap.
- This command specifically targets virtual authenticators – software emulations of real authenticators that are created for testing purposes.
- By issuing the “Set User Verified” command, you can directly control the
isUserVerified
property of a virtual authenticator. Setting this property totrue
instructs the virtual authenticator to behave as if user verification has been successfully completed.
How It’s Used in Testing
- Create a Virtual Authenticator: Use the
Add Virtual Authenticator
command to establish a virtual authenticator with desired properties (including support for user verification). - Add Credentials: Inject one or more credentials into the virtual authenticator using the
Add Credential
command. - Force User Verification Success: Execute the
Set User Verified
command, settingisUserVerified
totrue
for the specific virtual authenticator. - Trigger Authentication: Call
navigator.credentials.get()
in your test code to initiate a WebAuthn authentication flow. - Validate the Result: Since
isUserVerified
is set totrue
, the virtual authenticator will automatically consider user verification as successful, allowing you to test the subsequent steps in your authentication process.
Example (Conceptual)
// Assuming a WebDriver instance 'driver' and the authenticator ID 'myVirtualAuthenticatorId'
// Force user verification success on the virtual authenticator
driver.execute("POST", "/session/{session id}/webauthn/authenticator/myVirtualAuthenticatorId/uv", { isUserVerified: true });
// Now start your WebAuthn authentication flow in the test environment
navigator.credentials.get({ /* your WebAuthn options */ })
.then(assertion => {
// ... assert that the authentication proceeds as expected ...
});
Key Benefits for Testing
- Controlled Simulation: Allows you to precisely replicate user verification success without requiring a real user or a physical authenticator.
- Thorough Test Coverage: Enables you to explore various user verification success scenarios that would be difficult to reproduce manually.
- Streamlined Testing: Simplifies your test setup and automation.
Important Notes
- The “Set User Verified” command only works with virtual authenticators. It has no effect on physical authenticators.
- Always use appropriate test environments and avoid executing these commands against production systems.
Question: Why is it important to ensure that Virtual Authenticators only support the authenticator extensions listed in their extensions array?
It’s crucial to restrict Virtual Authenticators to only supporting the extensions explicitly listed in their extensions
array for these primary reasons:
1. Security and Predictability:
- Clear Scope of Functionality: By limiting supported extensions, you establish a clear and defined scope of what the Virtual Authenticator can do. This makes its behavior predictable and reduces the attack surface. If it could implicitly support extensions not listed, its actions might become unpredictable, potentially opening up vulnerabilities.
- Controlled Extension Interaction: Authenticator extensions often handle sensitive operations (e.g., user verification methods, large blob storage, etc.). Allowing unlisted extensions could mean these sensitive operations might be triggered unexpectedly or manipulated by malicious actors.
2. Testing Accuracy:
- Replicating Real-World Scenarios: WebDriver and Virtual Authenticators are designed for testing web applications. To ensure these tests are accurate, the Virtual Authenticator must closely mimic the behavior of real authenticators. Allowing arbitrary extensions deviates from this, making the tests unreliable.
- Isolate Extension Behavior: In testing, you often want to isolate and test the behavior of specific extensions. Allowing all extensions indiscriminately would make it difficult to pinpoint the effects of the extension under test.
3. Interoperability:
- Standard Conformance: The WebAuthn specification mandates that authenticators only support the extensions they explicitly declare. Enforcing this in Virtual Authenticators ensures consistency and interoperability with the broader WebAuthn ecosystem.
- Avoiding Unexpected Results: If a Virtual Authenticator implicitly supported all extensions, it might lead to unexpected results when interacting with web applications designed to work with specific extension sets.
In summary: Restricting Virtual Authenticators to their declared extensions is essential for maintaining security, ensuring accurate testing, and upholding the WebAuthn specification’s interoperability requirements. It provides a controlled and predictable environment for developing and testing web authentication flows.
Question: What are some limitations of the Web Authentication API that developers should be aware of?
Here are some limitations of the WebAuthn API that developers should keep in mind:
Authenticator Availability & Compatibility:
- Not Universally Supported: While WebAuthn adoption is growing, not all browsers and platforms fully support all its features. Older browsers or niche platforms might lack support, limiting the reach of your application.
- Authenticator Variety: Different authenticators have varying capabilities. Some might support resident keys (discoverable credentials/passkeys), while others might only support non-resident keys. You can specify preferences, but ultimately, the user’s authenticator dictates what’s possible.
- Extension Support Inconsistency: Not all authenticators implement all WebAuthn extensions (e.g.,
uvm
,largeBlob
,credProps
). This can create challenges if your application relies on specific extension features for advanced functionality.
User Experience & Usability:
- User Education: WebAuthn is a relatively new concept for many users. Relying solely on WebAuthn might confuse some, especially those accustomed to passwords. Providing clear instructions and fallbacks (like password options) is crucial.
- Authenticator Setup: The process of setting up and registering authenticators can be a barrier for some users.
- Error Handling: WebAuthn errors can be cryptic, making it difficult to provide user-friendly error messages. You’ll need robust error handling to guide users effectively.
- Limited UI Customization: The user interface for WebAuthn interactions is largely controlled by the browser and authenticator. You have limited control over its appearance and customization.
Security & Privacy:
- Phishing Susceptibility: While WebAuthn combats phishing better than passwords, users can still be tricked into authenticating to a malicious site that looks legitimate. Education about domain verification is essential.
- Single Point of Failure: Losing an authenticator can lock a user out of their account if no backup or recovery mechanisms are in place. Encourage users to register multiple authenticators or establish alternative recovery methods.
- Attestation Trustworthiness: Attestation provides information about the authenticator but doesn’t guarantee its security. Trusting attestation relies on the trustworthiness of the attestation provider and the absence of vulnerabilities in the attestation process.
Other Considerations:
- Credential Management: WebAuthn doesn’t inherently address credential management tasks like password resets or account recovery. You’ll need to implement separate mechanisms for these.
- Server-Side Complexity: Integrating WebAuthn on the server-side requires careful implementation to handle cryptographic operations, credential storage, and potential attacks.
Key Takeaway:
While WebAuthn offers significant security advantages, developers need to carefully consider its limitations and design their applications accordingly, prioritizing user experience, security, and compatibility.
Question: How can developers contribute to the development and improvement of the Web Authentication specification?
The Web Authentication (WebAuthn) specification is a living document, constantly being refined and improved. Developers play a crucial role in this process, and there are several ways you can contribute:
1. Provide Feedback and File Issues:
- Read the Spec: The most direct way to contribute is to familiarize yourself with the specification. The latest version is available on the W3C website (https://www.w3.org/TR/webauthn-3/).
- File Issues on GitHub: The WebAuthn specification is actively maintained on GitHub. If you find errors, inconsistencies, areas of ambiguity, or have suggestions for improvements, file detailed issues: https://github.com/w3c/webauthn/issues
- Participate in Discussions: Join the public-webauthn@w3.org mailing list to participate in discussions and follow ongoing conversations: https://lists.w3.org/Archives/Public/public-webauthn/
2. Implement and Experiment:
- Build WebAuthn into your Applications: Practical experience is invaluable. By integrating WebAuthn into your projects, you’ll gain firsthand knowledge of its strengths, limitations, and potential areas for improvement.
- Share your Experiences: Blog posts, articles, talks, and open-source code examples based on your implementations are highly valuable to the community. They provide real-world context and help other developers learn.
3. Develop Test Cases:
- Write Web Platform Tests: Web Platform Tests (WPT) are essential for ensuring the interoperability and consistency of WebAuthn implementations across different browsers. Contribute new tests to the WebAuthn WPT suite: https://github.com/web-platform-tests/wpt/tree/master/webauthn
4. Engage with the Working Group:
- Join the Web Authentication Working Group: Active participation in the working group discussions and meetings offers the most direct impact on the specification’s future: https://www.w3.org/groups/wg/webauthn
Key Areas for Future Development:
The WebAuthn spec is constantly evolving. Here are some areas where your contributions can be especially impactful:
- Passkeys: Passkeys are a new type of credential designed for a passwordless future. They offer significant usability and security advantages. Contribute to their adoption and refinement.
- New Attestation Formats and Extensions: As new authenticators and use cases emerge, there’s a need for additional attestation formats and extensions to address specific scenarios.
- Privacy Enhancements: Continuously improve the privacy protections offered by WebAuthn, ensuring that users’ sensitive information is safeguarded.
- Accessibility: Make WebAuthn accessible to users with diverse needs and abilities, contributing to a more inclusive web.
By actively contributing your expertise and perspectives, you can help shape the future of secure and user-friendly authentication on the web!
Question: What are some resources and best practices available for developers implementing Web Authentication in their applications?
Here are some of the best resources and practices for developers implementing WebAuthn:
Resources
- W3C Web Authentication Specification: This is the source of truth. While technical, it outlines everything you need. https://www.w3.org/TR/webauthn-3/
- WebAuthn Guide (MDN): Mozilla’s Developer Network provides an excellent, approachable guide with explanations and code examples. https://developer.mozilla.org/en-US/docs/Web/API/Web_Authentication_API
- FIDO Alliance: The organization behind WebAuthn, with specifications, white papers, and implementation guides. https://fidoalliance.org/
- WebAuthn.io: A community resource with guides, tools, and libraries for various programming languages. https://webauthn.io/
- WebAuthn.me: A testing tool to check WebAuthn support in your browser. https://webauthn.me/
Best Practices
- Security is Paramount:
- Secure Contexts Only: Implement WebAuthn exclusively over HTTPS.
- Strong Challenges: Generate cryptographically secure random challenges on the server-side.
- Validate Origins: Rigorously check the
origin
andrpId
values from the client data to prevent spoofing. - Protect Private Keys: Store credential private keys securely on the authenticator; they should never leave the device.
- Attestation Verification: (If applicable) Verify attestation statements to gain assurance about the authenticator.
- User Experience is Key:
- Clear Prompts: Provide users with clear and concise instructions during registration and authentication.
- Fallback Options: Offer fallback mechanisms (like one-time passwords) in case of authenticator issues.
- Manage Multiple Credentials: Enable users to register multiple authenticators for account recovery.
- User Choice: Support different authenticator types (platform, roaming) and let users choose.
- Implementation:
- Server-Side Validation: Perform the crucial signature verification and other checks on the server, not in client-side JavaScript.
- Leverage Libraries: Use existing WebAuthn libraries to simplify implementation in your chosen language.
- Thorough Testing: Test your implementation across various browsers and authenticator types.
- Stay Updated: WebAuthn is evolving. Keep up-to-date with the latest specifications and best practices.
Specific Considerations
- Passwordless Authentication: WebAuthn is powerful for passwordless flows. Carefully design your user experience and account recovery mechanisms.
- Multi-Device Credentials: These are a newer feature of WebAuthn that allows credentials to be backed up and synced. Evaluate the security and privacy implications before using them.
- FIDO Metadata Service: This service can provide information about certified authenticators, aiding in attestation verification.
Remember:
WebAuthn implementation can seem daunting at first, but it’s well worth the effort to enhance security. Start by understanding the core concepts, leverage the available resources, and follow best practices to build a secure and user-friendly authentication experience.
Question: How can developers stay informed about updates and changes to the Web Authentication specification?
Staying informed about the ever-evolving landscape of Web Authentication is crucial for any developer working with this technology. Here’s a breakdown of key resources and strategies:
1. Official W3C Channels:
- WebAuthn Specification: The official W3C Web Authentication specification is the definitive source of truth. Subscribe to the public-webauthn mailing list for updates on drafts and publications. The latest editor’s draft and links to previous versions are found here.
- GitHub Repository: The W3C maintains a GitHub repository for the WebAuthn specification. Follow the repository to track issue discussions, pull requests, and commits.
2. FIDO Alliance Resources:
- FIDO Alliance Specifications: The WebAuthn standard is developed in close collaboration with the FIDO Alliance. Stay updated on related FIDO specifications like CTAP (Client to Authenticator Protocol) on their specifications page.
- FIDO Developer Resources: FIDO provides various resources, guides, and best practices on their website, specifically targeted towards developers. Explore their developer resources section.
3. Community and Industry Blogs:
- Web Security Blogs: Prominent web security blogs often cover WebAuthn updates and advancements. Some well-regarded sources include:
- Authenticator Vendor Blogs: Many companies that manufacture authenticators (e.g., Yubico, Google, Microsoft) publish blogs with news and insights into WebAuthn developments.
4. Developer Communities and Forums:
- Stack Overflow: The “webauthn” tag on Stack Overflow is a valuable resource for asking questions, finding solutions to problems, and learning from other developers.
- WebAuthn Community Group: Join online communities and forums focused on WebAuthn. Look for relevant groups on platforms like Reddit, Slack, or Discord.
5. Conferences and Workshops:
- Security Conferences: Major security conferences like Black Hat, DEF CON, and RSA Conference often feature talks and presentations on WebAuthn and authentication security.
- FIDO Alliance Events: Attend FIDO Alliance events and webinars to connect with experts and learn about the latest developments in the FIDO ecosystem.
Best Practices:
- Regularly Review Official Specifications: Make it a habit to periodically revisit the official WebAuthn specification and related FIDO documents to catch any changes.
- Set Up Alerts: Configure email alerts or RSS feeds for keywords like “WebAuthn,” “FIDO,” and “authentication security” to receive notifications about relevant news and updates.
- Engage in the Community: Actively participate in developer communities, ask questions, and share your knowledge to contribute to the advancement of WebAuthn.
By following these strategies, you can ensure that you’re using the most current and secure implementations of Web Authentication in your projects.
Question: What are the future directions and potential advancements for Web Authentication technology?
WebAuthn is a powerful technology, but it’s still evolving. Here’s a look at some promising future directions and advancements for WebAuthn:
1. Enhanced User Experience:
- Streamlined Passkey Management: Passkeys, the newest form of WebAuthn credentials, still need more intuitive management solutions. We could see dedicated apps or browser interfaces for better organization, sharing, and recovery of passkeys.
- Improved UI/UX for Multi-Device Credentials: User interfaces need to be smarter about prompting users to use the most appropriate authenticator (platform or roaming) in different contexts. We can expect more user-friendly ways to manage backups and sync credentials across devices.
- Conditional UI: Current conditional UI primarily focuses on credential discovery. Future improvements could dynamically adjust the entire user flow based on available authenticators, risk assessments, and user preferences.
- Fallback Mechanisms: Robust fallback options are crucial when passkeys are unavailable (e.g., on older devices or in emergencies). We may see more integrated alternatives like one-time codes, magic links, or biometrics in combination with other factors.
2. Broader Device Support:
- Legacy Device Compatibility: To achieve widespread adoption, WebAuthn must find solutions for older devices that may not have built-in biometric sensors or support the latest protocols. This might involve bridge solutions or hybrid approaches.
- Extending Beyond the Web: Integrating WebAuthn into native mobile apps, desktop applications, and IoT devices will make it more versatile. We are seeing initial efforts in this direction, like the use of QR codes for cross-platform authentication.
3. Increased Security and Privacy:
- Post-Quantum Cryptography: As quantum computing evolves, we need to ensure WebAuthn’s cryptographic foundations remain secure. Migrating to post-quantum algorithms will be essential to maintain long-term robustness.
- Advanced Attestation Mechanisms: New attestation formats and methods could provide greater transparency and flexibility for Relying Parties to assess authenticator properties and security guarantees.
- Privacy-Preserving Extensions: Techniques like differential privacy and zero-knowledge proofs could further enhance user privacy while maintaining strong authentication.
4. New Use Cases and Applications:
- Passwordless Everything: WebAuthn’s passwordless approach can extend beyond websites to applications, operating systems, and network access (SSH, VPNs).
- Stronger Identity Verification: Using WebAuthn-based credentials in combination with verified identity data could lead to more secure and trustworthy digital identity systems.
- Decentralized Identity (DID): WebAuthn can play a role in managing and authenticating decentralized identifiers, giving users more control over their online identities.
5. Standardization and Collaboration:
- Continued Development of WebAuthn Standards: The W3C and FIDO Alliance are actively working on new specifications and extensions.
- Industry Collaboration: Stronger collaboration between browser vendors, operating system developers, authenticator manufacturers, and Relying Parties will be crucial to drive adoption and ensure interoperability.
In Summary:
The future of WebAuthn holds great potential to significantly enhance online security and user experience. By addressing current limitations, embracing new technologies, and fostering collaboration, we can create a more secure, privacy-respecting, and passwordless digital world.
References:
Web Authentication: An API for accessing Public Key Credentials – Level 3 (w3.org)