Table of Contents
Introduction
In this blog series, we’ll dive into a variety of salesforce scenarios—ranging from simple to complex. Together, we’ll explore multiple ways to tackle each challenge, weighing the pros and cons of each approach. By the end of each post, I’ll share the final solution I recommend. Get ready to master salesforce with innovative strategies and practical insights!
Scenario: Upon account creation, if the industry field has value as ‘Agriculture’ or ‘Biotechnology’, then populate the rating as ’Hot’.
Analysis
Check which condition we are writing this solution for (as the problem states upon account creation, we are considering only the following two scenarios):
- Before save
- After save
So, we are creating this before save, at the time of account creation, before saving it to the database.
A quick tip: There are a few small things to watch out for along the way, but they will make a big contribution towards good quality development.
Solutions
Solution 1: Salesforce record triggered flow
I am using a before save fast update because the computing power of before save operations is greater than that of after create. They are faster than after create.
There are two ways to construct this flow, depending on the scenario you are working on.
Approach 1
We can add the conditions in the entry itself if this is the only operation for the account before save. This will save an additional decision component from being added to the flow, as the conditions are defined at the entry itself.
This also eliminates all the accounts that do not satisfy the conditions from even starting to execute this flow.
The name of the flow should follow this theme if it is a suitable option for your implementation.
‘Account: Before Save’ is the name of the flow below, which gives an idea about the flow with its name itself.
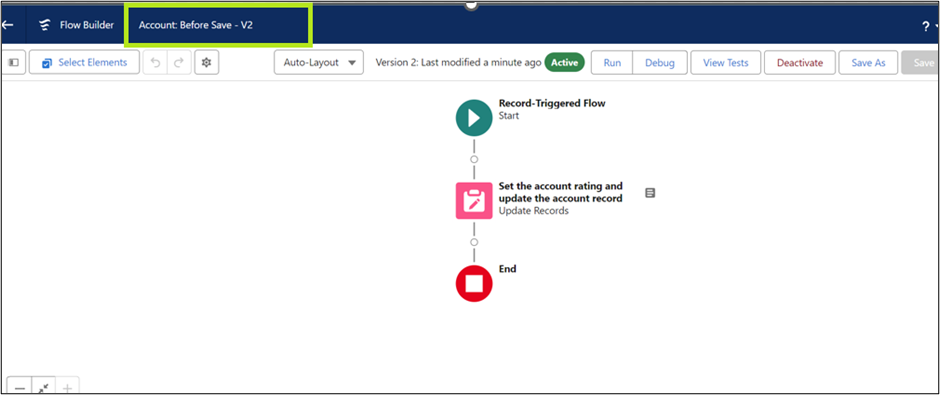
Follow a naming convention for the names and descriptions of each element, including the flow itself.
Example: Use camel casing for flow names, and sentence casing for all other components.
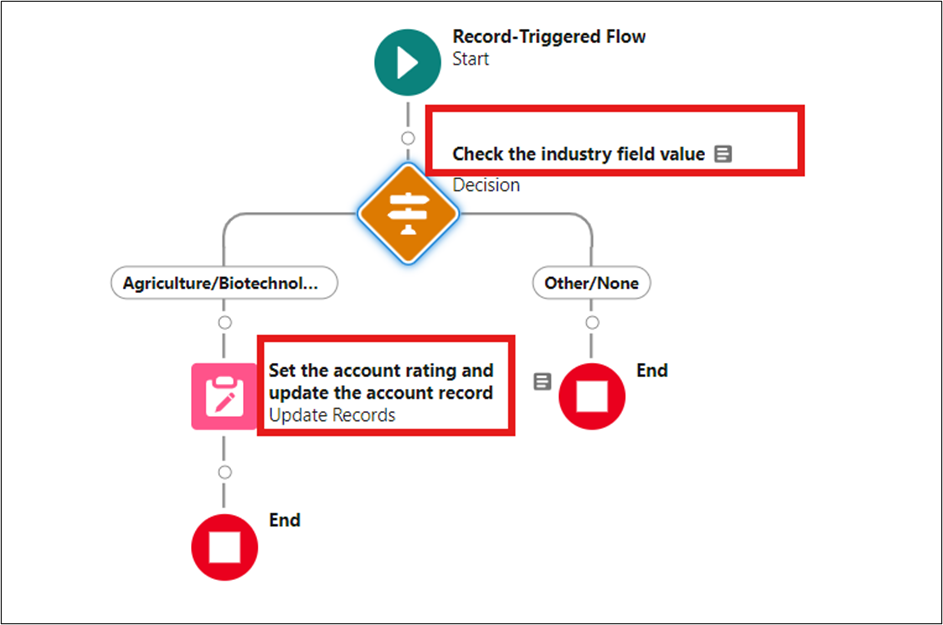
The description should include one line to give a general idea about the flow, and we can have pointers for each operation/task happening in the flow. This will be an incremental change as and when a developer makes changes or adds functionality to the flow.
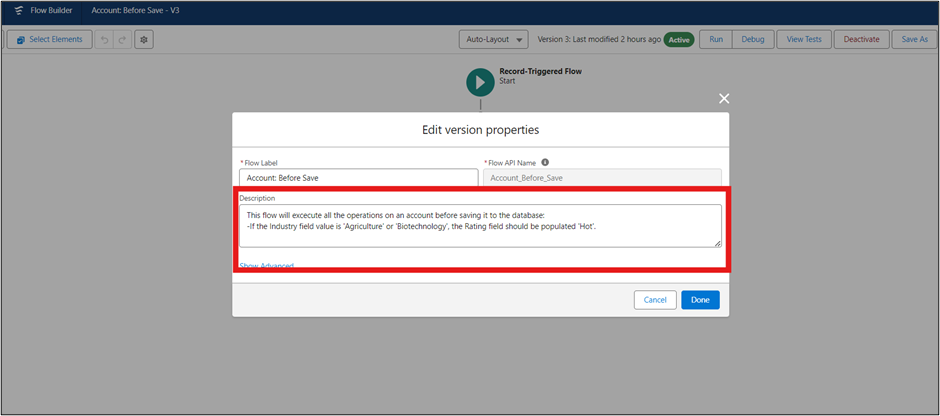
Each component in the flow should have a description detailing what the component is actually doing.
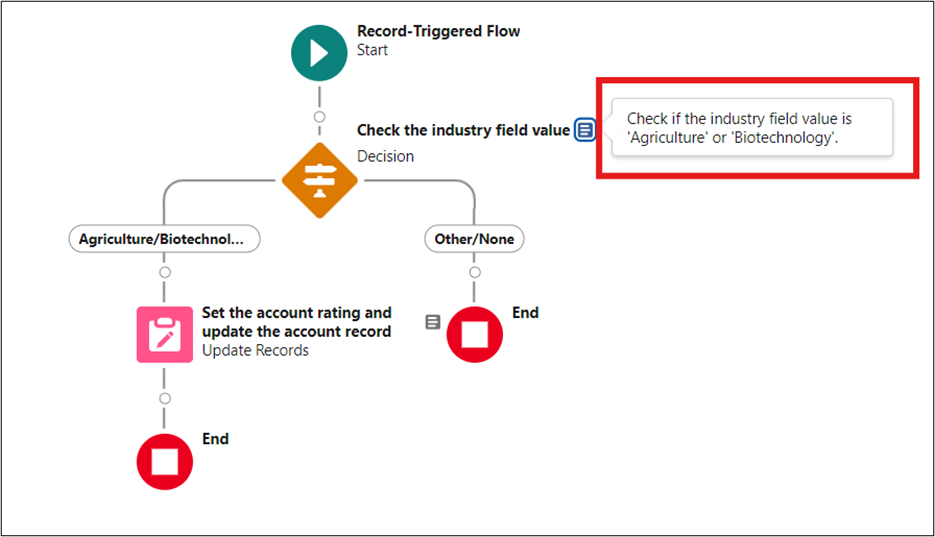
- Complete flow screenshot:
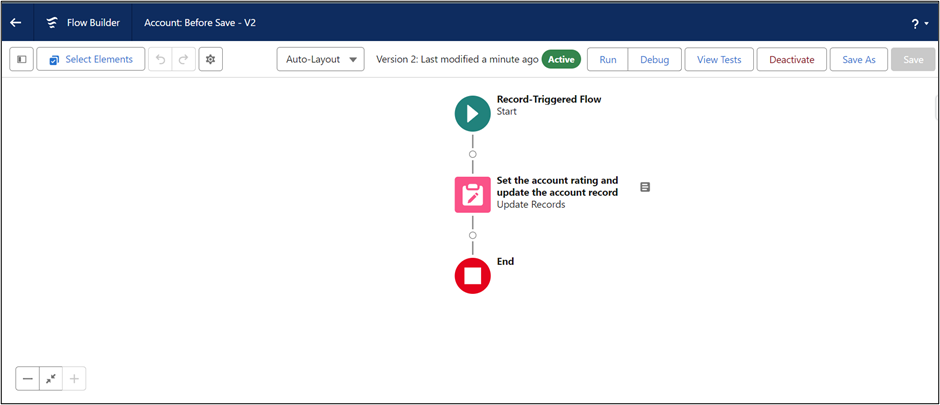
Start element screenshot:
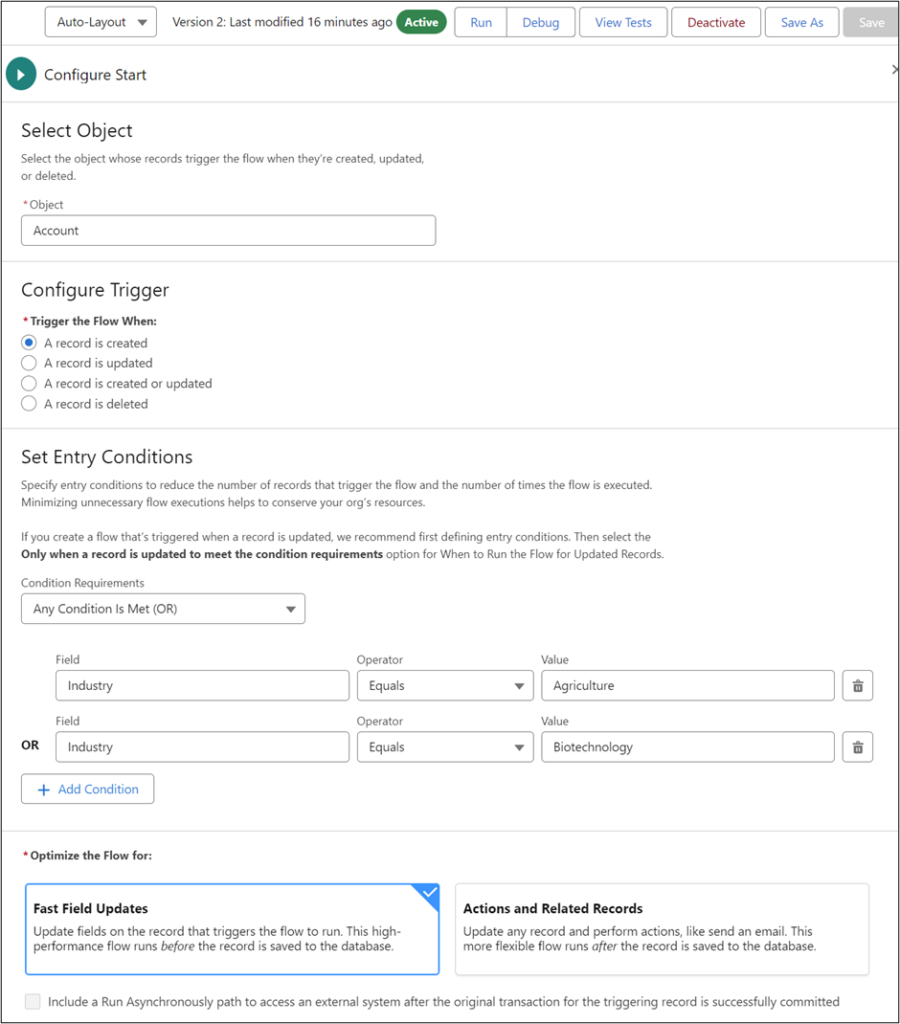
Update the triggering record screenshot:
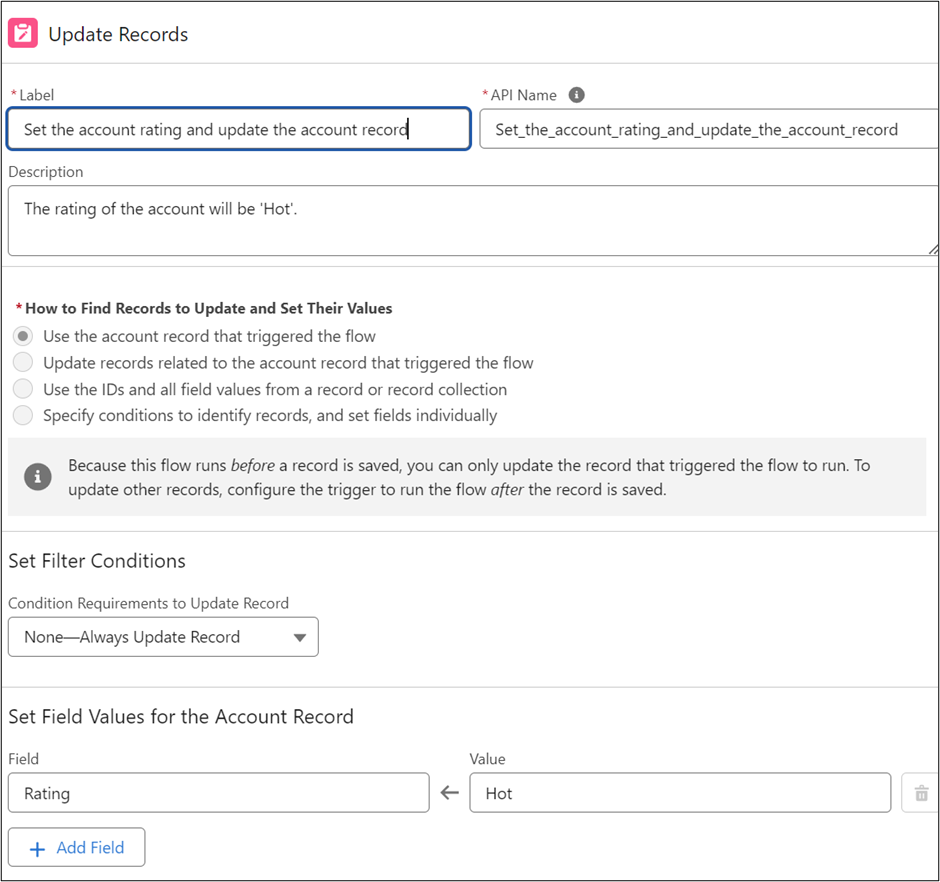
- End.
- Test Cases:
- It is always a good practice to write test cases for a flow.
- Cover as many scenarios as possible, including positive and negative ones.
Below is the screenshot of the four test cases for the above flow (created manually). The negative scenarios will fail in this case because the entry conditions are written at the start element of the flow. Since the triggering record didn’t meet the condition requirements, the flow did not run and thus failed the two scenarios where the Industry field value is ‘Apparel’ and another where the Industry field value is blank, both constituting negative scenarios.
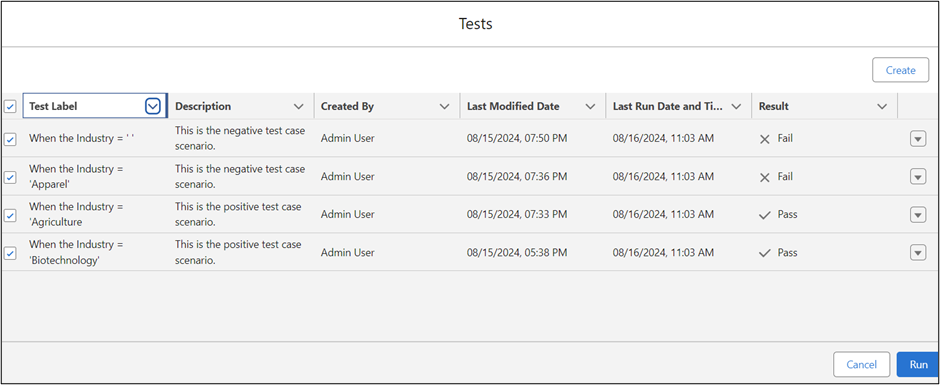
Approach 2
We leave the entry component as it is without defining any conditions, as there might be other operations we need to carry out before saving the account.
- There will be an additional decision element to add the filter conditions.
- All accounts will start the execution of this flow and then exit according to the decision criteria met.
Complete flow screenshot:
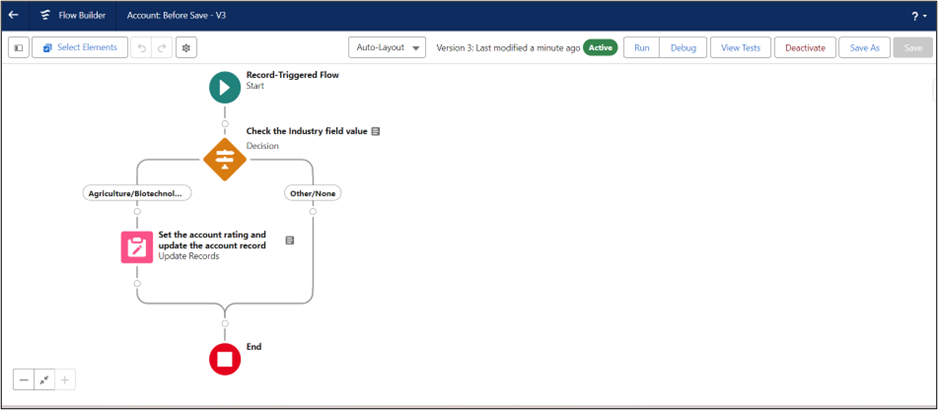
Start element screenshot:
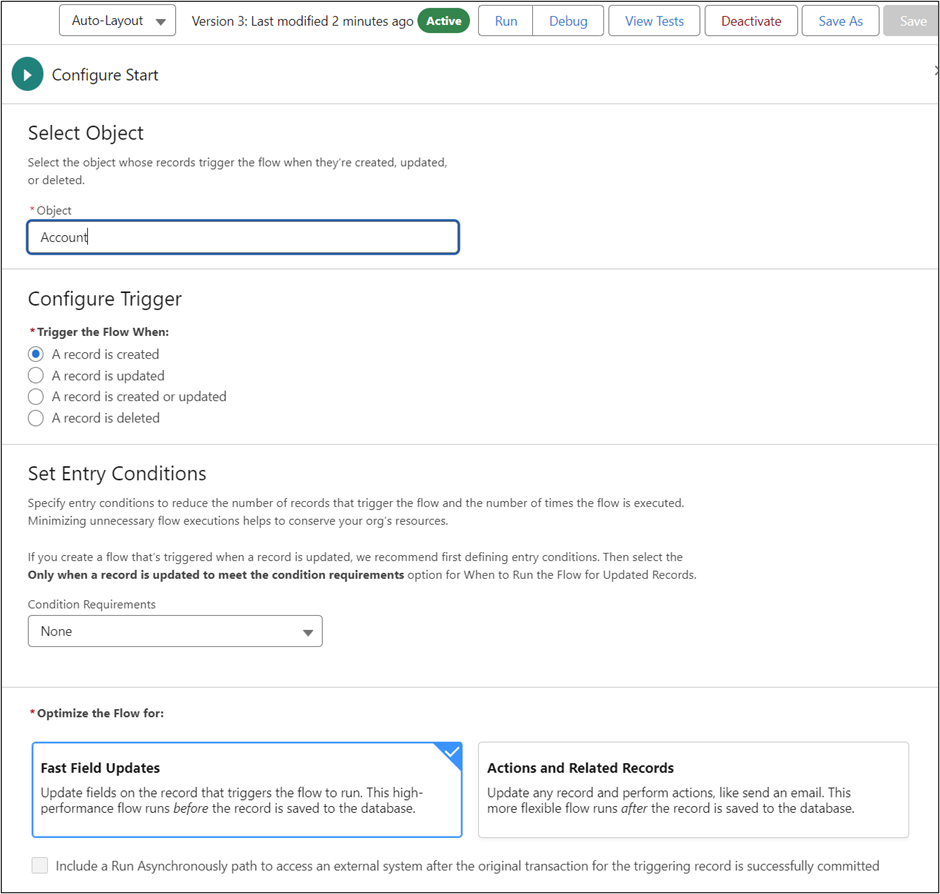
Check Industry decision element screenshot:
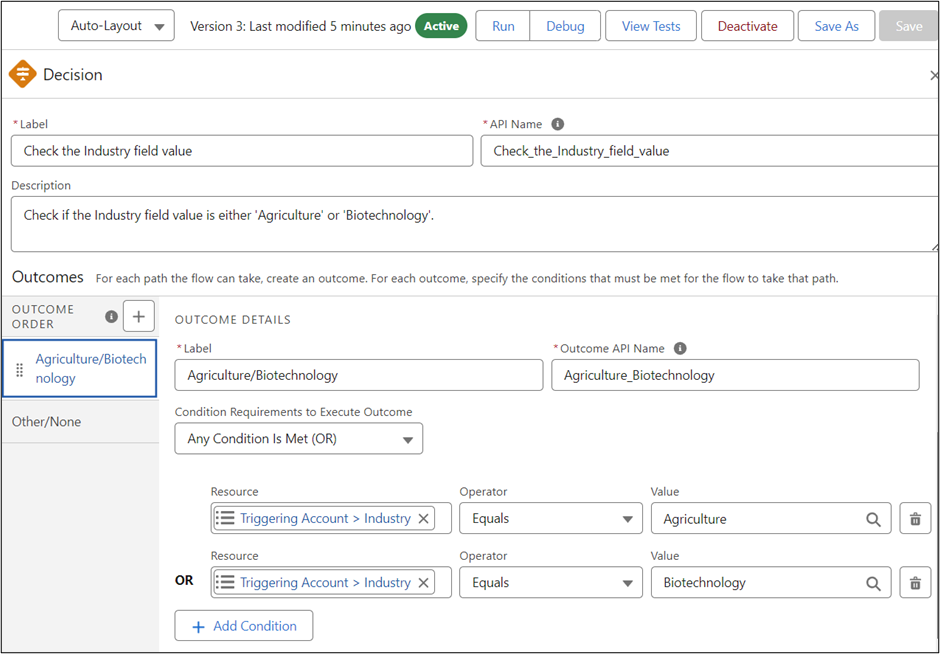
Update the triggering record screenshot:
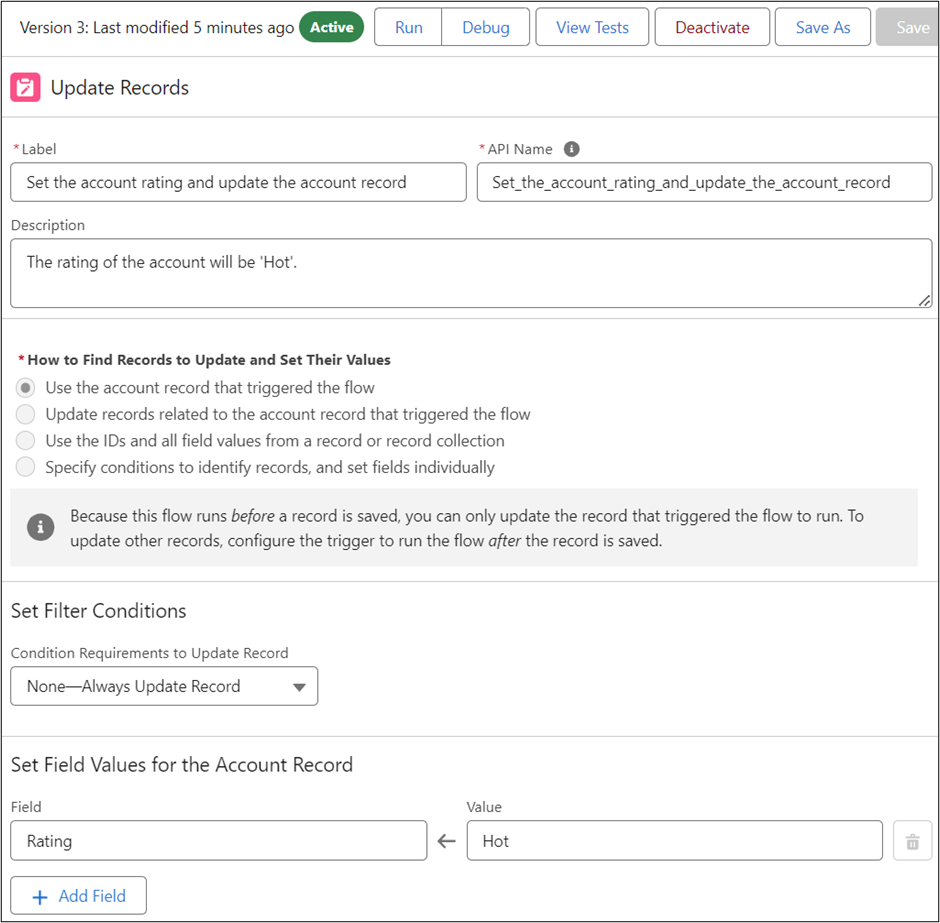
- End.
- Test cases:
- It is always a good practice to write the test cases for a flow.
- Cover as much scenarios as possible. Positive, negative, etc.
Below is the screenshot of the 4 test cases for the above flow:
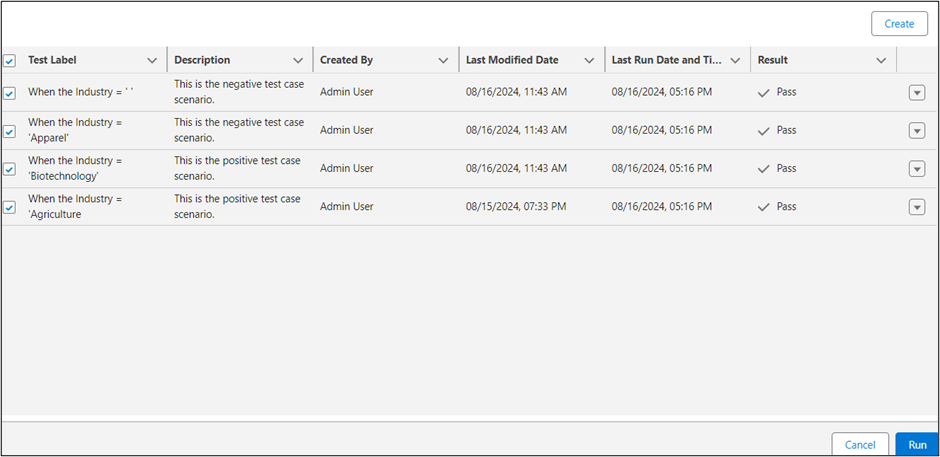
Solution 2: Apex trigger before insert
Here, we are following a basic trigger framework where the code will be in the handler and not in the trigger itself.
We are using a before insert trigger because it allows us to modify the account records before they are saved to the database. This ensures that any necessary updates or validations are applied efficiently, leveraging the higher computing power and faster execution of before insert operations compared to after insert triggers.
AccountTrigger code
trigger AccountTrigger on Account (before insert) {
//call the updateAccountRating from the AccountTriggerHandler class
AccountTriggerHandler.updateAccountRating(Trigger.New);
}
AccountTriggerHandler class code
public class AccountTriggerHandler {
public static void updateAccountRating(List<Account> accList){
for(Account acc: AccList){
//check if the industry field value is 'Agriculture' or 'Biotechnology'
if(acc.Industry == 'Agriculture' || acc.Industry == 'Biotechnology'){
//If yes, then update the rating to 'Hot'
acc.Rating = 'Hot';
}
}
}
}
AccountTriggerHandlerTest class code
@isTest
public class AccountTriggerHandlerTest {
@isTest
public static void updateAccountRating(){
//create sample data
List<Account> accList = new List<Account>();
for(Integer i=1;i<=5;i++){
Account acc = new Account();
acc.Name = 'Test account '+i;
acc.Industry = 'Agriculture';
accList.add(acc);
}
//perform the dml
Test.startTest();
insert accList;
Test.stopTest();
//query one of the inserted records and check for assertion
List<Account> accs = [SELECT ID, name, Industry, Rating from Account WHERE ID
=:accList[0].ID];
System.assertEquals('Hot',accs[0].Rating);
}
}
Bulk code execution test: It is always important to test your code for bulk execution in case if it fails. I am doing the above test by inserting 1000 account records in the system and checking for any failures.
Below is the code executed in the anonymous window to test for bulk execution for both flow and trigger.
List<Account> accList = new List<Account>();
for(Integer i=0;i<1000;i++){
Account acc = new Account();
acc.Name = 'Test trigger acc '+i;
acc.Industry = 'Agriculture';
accList.add(acc);
}
insert accList;
For both the above scenarios, the records were inserted without any issues. Yay we are good to go.
Conclusion
We have seen the execution with both trigger and flow. Usage of each will depend on the org and scenario’s that are being tackled. For this scenario here are my thoughts:
- If performance and efficiency are critical, and you have the necessary coding skills, an apex trigger would be preferred.
- If ease of maintenance and visualization are more important, and the logic is relatively simple, a flow would be a good choice.
Final thoughts
We’d love to hear your thoughts on the blog above!
What approach would you prefer if faced with this scenario? Are there any other methods you would consider as a solution to this problem statement?